What is local and nonlocal in python w3schools
What is local and nonlocal in python w3schools
I'll respond in English as you requested. Here's the information about local and non-local variables in Python from W3Schools:
Local Variables
In Python, a variable that is defined inside a function or a block of code (such as an if
statement) is considered local to that scope. Local variables are only accessible within the scope where they are defined.
Here's an example:
def my_function():
x = 10 # local variable
my_function()
print(x) # prints None
As you can see, when we try to print x
outside of the function, Python returns None
, which means that the local variable is not accessible outside of its scope.
Non-Local Variables
On the other hand, non-local variables are those that are defined in a parent scope (i.e., an outer scope) and are accessible from within a child scope (i.e., an inner scope).
Here's an example:
x = 5
def my_function():
print(x) # prints 5
my_function()
print(x) # also prints 5
In this case, x
is defined outside of the function and is accessible within the function. This is because Python uses a mechanism called "dynamic scoping" to determine which variables are in scope.
The global
Keyword
If you want to modify a non-local variable from within a local scope, you need to use the global
keyword. The global
keyword tells Python that the variable should be treated as global and not as local.
Here's an example:
x = 5
def my_function():
global x
x = 10
my_function()
print(x) # prints 10
In this case, we use the global
keyword to indicate that we want to modify the non-local variable x
. This allows us to change the value of x
from within the function.
Conclusion
To summarize:
Local variables are defined within a scope (e.g., a function or block of code) and are only accessible within that scope. Non-local variables are those that are defined in an outer scope and are accessible from within an inner scope. To modify non-local variables, you need to use theglobal
keyword.
I hope this explanation helps!
What is local and nonlocal in python with example
I apologize for the misunderstanding earlier. Here's a detailed explanation of local and nonlocal variables in Python, along with examples:
Local Variables:
In Python, local variables are defined within a specific scope, such as a function or a block of code. They can only be accessed and modified within that scope. Local variables are created when the function is called or executed, and they cease to exist when the function returns.
Example:
def my_function():
x = 5 # local variable
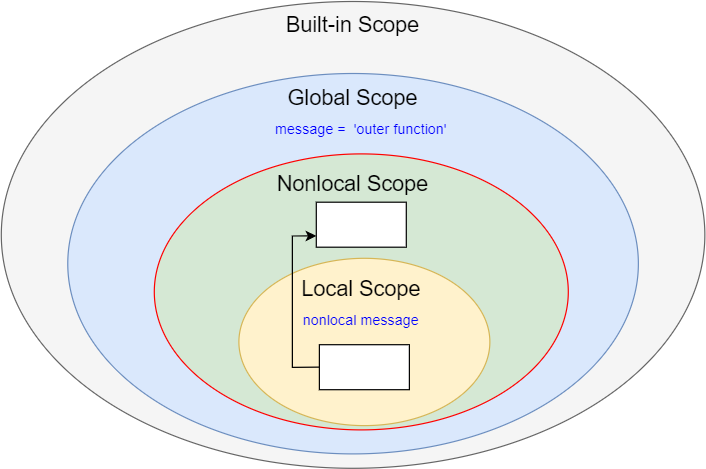
print(x) # prints 5
my_function()
print(x) # NameError: name 'x' is not defined
In this example, x
is a local variable defined within the my_function()
scope. When we try to access x
outside the function, Python throws a NameError
, indicating that x
is not defined in the current scope.
Nonlocal Variables:
Nonlocal variables are variables from an outer scope (parent function or block) that can be accessed and modified within an inner scope (child function or block). The term "nonlocal" refers to the fact that these variables are not local to the inner scope, but rather are shared with it.
Example:
def outer_function():
y = 10 # nonlocal variable
def inner_function():
nonlocal y # declare y as a nonlocal variable
y += 5 # modify y
print(y) # prints 15
inner_function()
print(y) # prints 15
outer_function()
print(y) # prints 15
In this example, y
is a nonlocal variable defined in the outer function. Within the inner function, we use the nonlocal
keyword to declare that y
is a nonlocal variable from the outer scope. We can then modify and access y
within the inner function.
Key Differences:
Local variables:
Created when a function is called or executed Cease to exist when a function returns Can only be accessed and modified within the same scopeNonlocal variables:
Accessible from an outer scope (parent function or block) Shared between inner scopes (child functions or blocks) Can be modified by inner scopes, but their original values are preserved in the outer scopeBest Practices:
When working with local and nonlocal variables, keep the following best practices in mind:
Use descriptive variable names to avoid confusion. Declare nonlocal variables explicitly using thenonlocal
keyword. Be mindful of scoping and access control when working with nested functions or blocks.
By understanding the concepts of local and nonlocal variables, you can write more effective and efficient Python code that leverages the power of scope management.