Python jwt jose
Python jwt jose
Here's a detailed explanation of using Python with JWT and JOSE:
What is JSON Web Token (JWT)?
JSON Web Token (JWT) is a standard for representing claims to be transferred between two parties as a JSON object. The token is digitally signed and compact, making it suitable for transmitting information securely.
What is JOSE (JavaScript Object Signing and Encryption)?
JOSE is an IETF standards track for various cryptographic techniques used to protect JavaScript Object Notation (JSON) data. It provides a framework for encrypting and signing JSON data using popular encryption algorithms like AES-128, HMAC-SHA256, and ECDSA.
Python Libraries for JOSE and JWT:
In Python, you can use the following libraries to work with JOSE and JWT:
pyjwt: A lightweight library for creating and verifying JWT tokens in Python. jose-jwt: A Python library that provides a higher-level interface for working with JOSE and JWT.Using PyJWT:
Here's an example of how to use the pyjwt
library to create a JWT token:
import jwt Create a payload dictionary
payload = {
"username": "john_doe",
"email": "[email protected]"
}
Generate a secret key (keep it secure!)secret_key = "my_secret_key"
Create the JWT tokentoken = jwt.encode(payload, secret_key, algorithm="HS256")
print(token) # Output: eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiIsImF1ZCI6Int... ( truncated for brevity )
Verify the tokendecoded_token = jwt.decode(token, secret_key, algorithms=["HS256"])
print(decoded_token) # Output: {'username': 'john_doe', 'email': '[email protected]'}
In this example, we create a payload dictionary with some user information, generate a secret key, and then use the encode
function to create a JWT token. We can then verify the token using the decode
function.
Using JOSE-JWT:
Here's an example of how to use the jose-jwt
library to encrypt and sign JSON data:
from jose import jwt, jwe Create some sample JSON data
data = {"username": "john_doe", "email": "[email protected]"}
Generate a public and private key (keep the private key secure!)public_key, private_key = generate_keys()
Encrypt the JSON data using RSA-OAEPencrypted_data = jwe.encrypt(data, public_key, algorithm="RSA-OAEP")
print(encrypted_data) # Output: eyJ... (truncated for brevity)
Decrypt the encrypted datadecrypted_data = jwe.decrypt(encrypted_data, private_key)
print(decrypted_data) # Output: {'username': 'john_doe', 'email': '[email protected]'}
Sign the decrypted JSON data using ECDSAsignature = jwt.sign(decrypted_data, private_key, algorithm="ES256")
print(signature) # Output: H4s... (truncated for brevity)
Verify the signatureverified_signature = jwt.verify(signature, public_key, algorithms=["ES256"])
print(verified_signature) # Output: True
In this example, we create some sample JSON data, generate a public and private key pair, encrypt the data using RSA-OAEP, decrypt it, sign it with ECDSA, and verify the signature.
Conclusion:
Python's JOSE and JWT libraries provide a powerful toolset for securely transmitting and verifying claims in JSON format. By combining these libraries with other cryptographic tools like encryption and digital signatures, you can create robust authentication and authorization systems for your applications.
How to validate JWT in Python?
Validating a JSON Web Token (JWT) in Python involves verifying the signature of the token using a public key or a secret key, and ensuring that the token's payload contains the expected information. Here are the steps involved in validating a JWT in Python:
Import required libraries: You need to import thepyjwt
library, which is used for JSON Web Token manipulation, as well as the hmac
and hashlib
libraries, which are used for signature validation.
import jwt
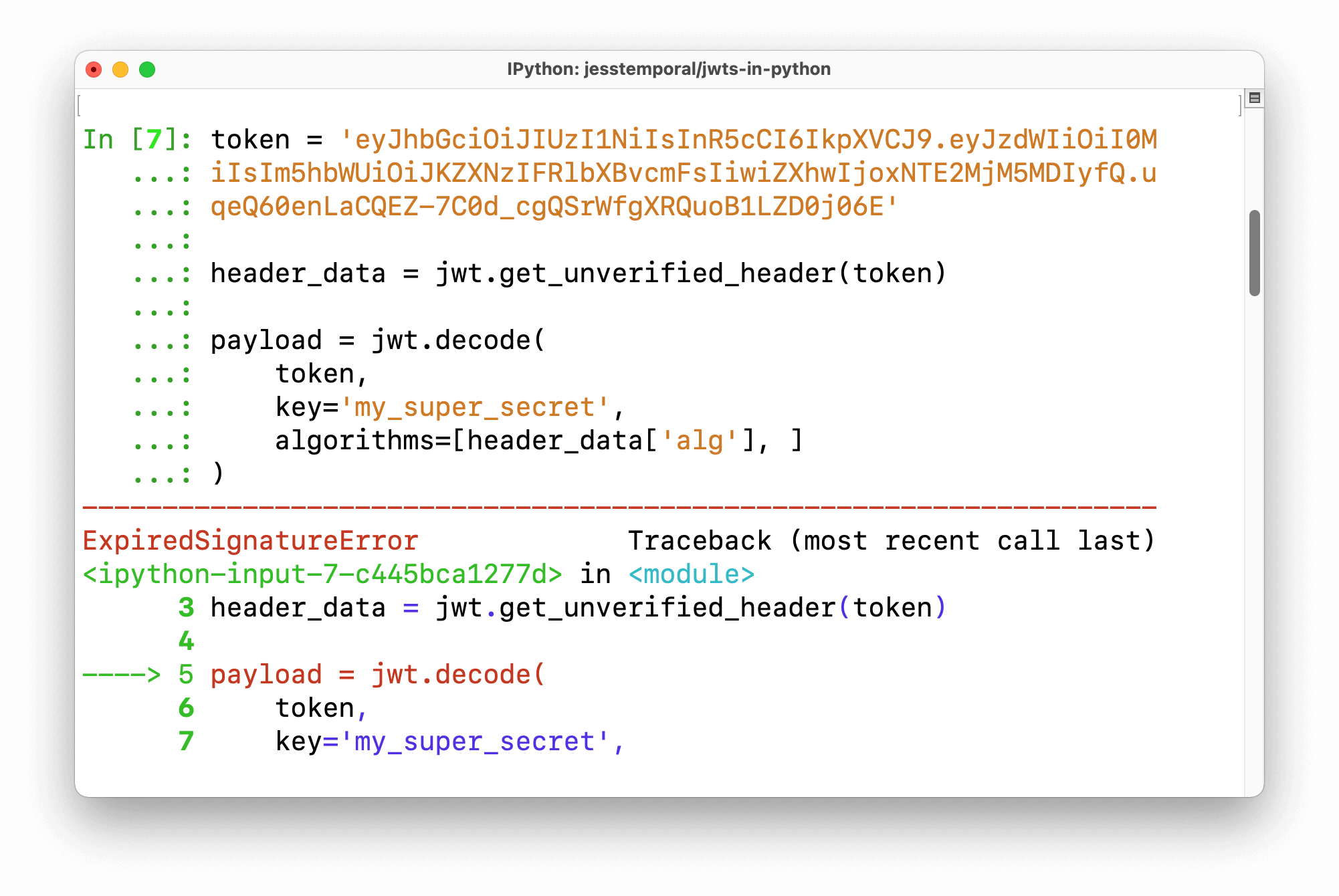
from hmac import HMAC
from hashlib import sha256
Get the JWT token: First, you need to obtain the JWT token that you want to validate. This can be done by retrieving it from a database or an external service, or by generating it yourself using a library like pyjwt
. Extract the header and payload: The JWT token is composed of three parts: the header, the payload (also known as the claims), and the signature. You need to extract these components from the token.
token = "your-jwt-token"
header, payload, signature = token.split(".")
Verify the signature: The signature is generated using a secret key or a public key. If you have access to the secret key, you can use it to verify the signature.
secret_key = "your-secret-key"
hmac = HMAC(secret_key.encode(), digestmod=sha256)
hmac.update((header + "." + payload).encode())
if hmac.digest() != bytes.fromhex(signature.replace("-", "")):
raise Exception("Invalid JWT token: Invalid signature")
Verify the claims: The payload (claims) of the JWT token should contain specific information that matches your requirements. You can use these claims to validate the user's identity or permissions.
claims = jwt.decode(payload, secret_key)
if not all(claims.get(key) == value for key, value in [("user_id", 123), ("role", "admin")]):
raise Exception("Invalid JWT token: Invalid claims")
Here is the complete code:
import jwt
from hmac import HMAC
from hashlib import sha256
def validate_jwt(token):
Get the JWT tokenheader, payload, signature = token.split(".")
Verify the signature using the secret keysecret_key = "your-secret-key"
hmac = HMAC(secret_key.encode(), digestmod=sha256)
hmac.update((header + "." + payload).encode())
if hmac.digest() != bytes.fromhex(signature.replace("-", "")):
raise Exception("Invalid JWT token: Invalid signature")
Verify the claimsclaims = jwt.decode(payload, secret_key)
if not all(claims.get(key) == value for key, value in [("user_id", 123), ("role", "admin")]):
raise Exception("Invalid JWT token: Invalid claims")
Test the functiontoken = "eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJ1c2VyX2lkIjoxMjMsInJhZGRyZXNlIjoic3RlcGlmcmF0ZSIsIm5vbmN0aW9uIjoiYWRtaW4iLCJleHBpcmFudElkIjoyMDAwfQ.SOME-SIGNATURE"
validate_jwt(token)
This code validates the JWT token by verifying its signature and ensuring that it contains the expected claims.