Python ssl github example
Python ssl github example
Here is an example of using the ssl
module in Python to interact with a GitHub API:
import requests
import ssl
from urllib.request import Request, urlopen
Set up the SSL context
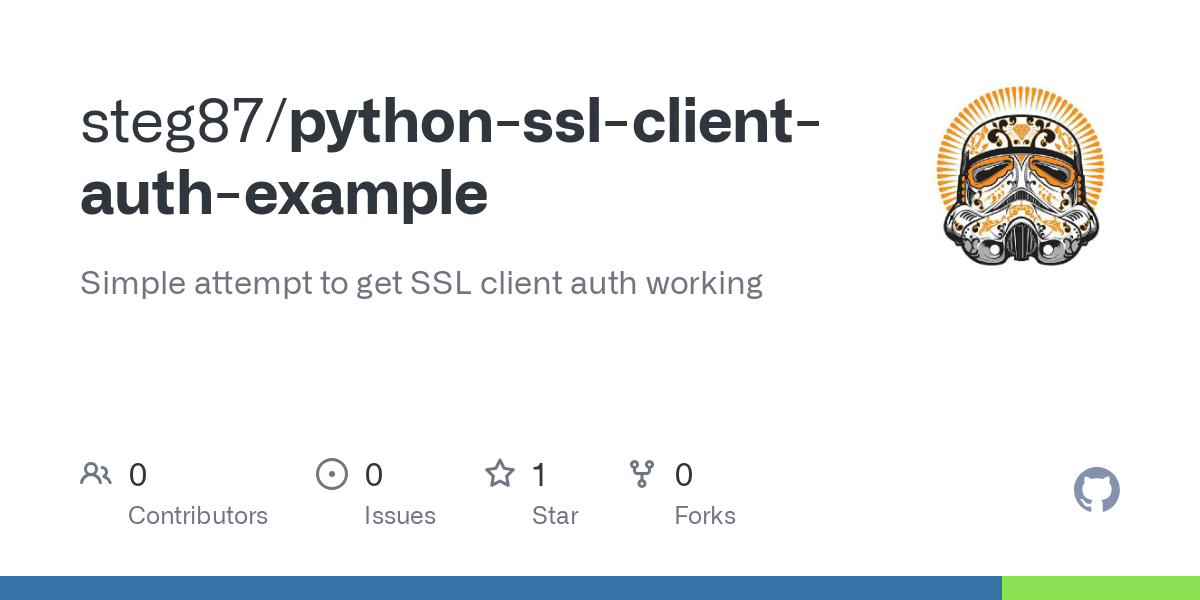
ctx = ssl.create_default_context()
Make a request to GitHub's API
req = Request('https://api.github.com/repos/username/repo_name', headers={'User-Agent': 'Python'})
f = urlopen(req, context=ctx)
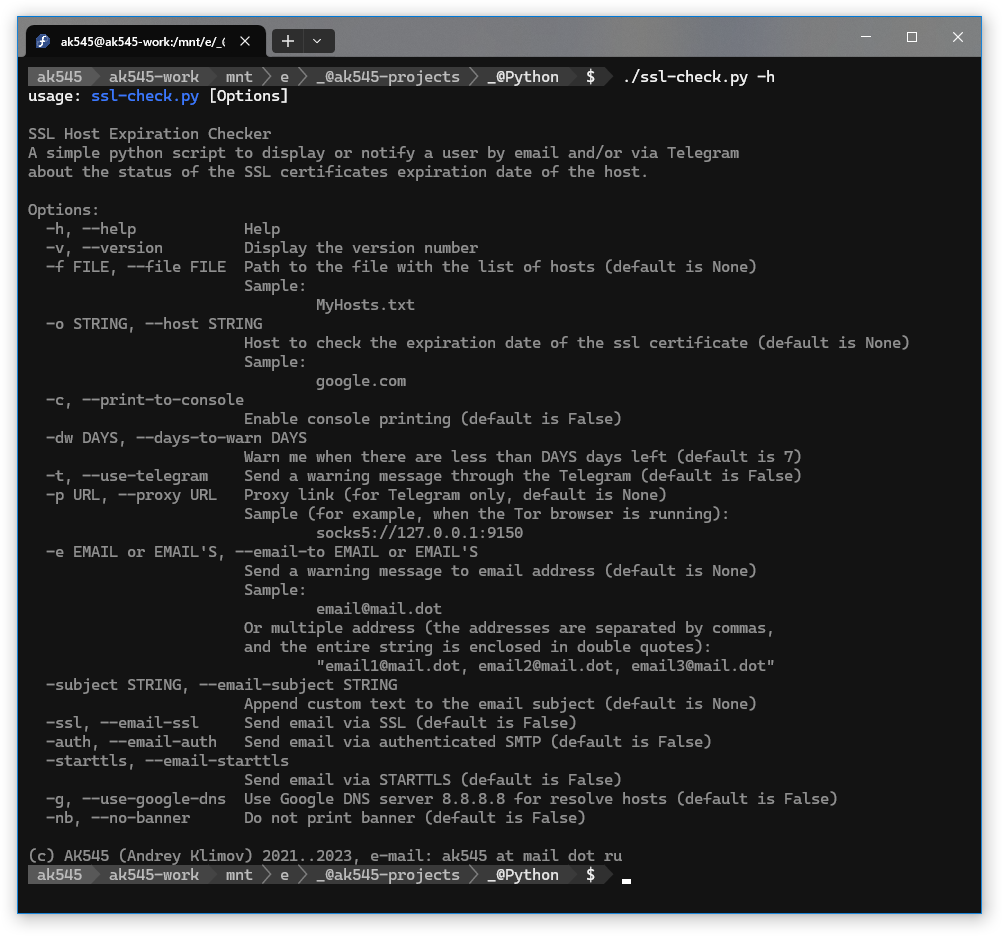
Read the response
response_body = f.read().decode('utf-8')
print(response_body)
This code snippet uses Python's built-in requests
library and the ssl
module to send a GET request to GitHub's API. The Request
object is used to create a custom HTTP request, and the urlopen()
function is then called with this request object.
The ssl.create_default_context()
function creates an SSL context that will be used for all subsequent connections. This ensures that the connection is encrypted and secure.
Note: Please replace 'username' and 'repo_name' with your actual GitHub username and repository name.
In a real-world scenario, you would typically handle exceptions, decode JSON responses, and use the response data to perform actions within your application.
For instance, if you wanted to fetch the repository's README file:
import requests
import json
Make a request to GitHub's API for the README
req = Request('https://api.github.com/repos/username/repo_name/blob/master/README.md', headers={'User-Agent': 'Python'})
f = urlopen(req)
Read and decode the response JSON
response_body = f.read().decode('utf-8')
data = json.loads(response_body)
print(data['content'])
In this case, you would be printing out the README file's content as a string.
OpenSSL python example
Here is an OpenSSL Python example using the pyOpenSSL
library:
Generating RSA Key Pair
To generate a new RSA key pair, we can use the following code:
from OpenSSL import crypto
Generate private and public key
pkey = crypto.PKey()
pkey.generate(crypto.TYPE_RSA, 2048)
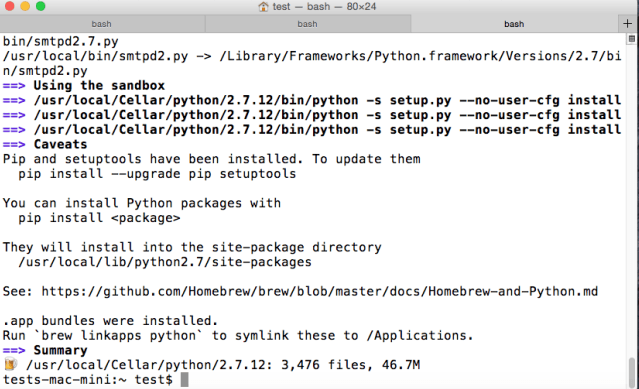
Convert PKey to PEM format
private_pem = crypto.dump_privatekey(crypto.FILETYPE_PEM, pkey)
public_pem = crypto.dump_publickey(crypto.FILETYPE_PEM, pkey)
print("Private Key (PEM):")
print(private_pem.decode('utf-8'))
print("nPublic Key (PEM):")
print(public_pem.decode('utf-8'))
This code generates a new RSA key pair with a 2048-bit modulus and converts the private and public keys to PEM format.
Signing Data
To sign some data using the generated private key, we can use the following code:
from OpenSSL import crypto
import hashlib
Load private key from file
pkey = crypto.load_privatekey(crypto.FILETYPE_PEM, "private_key.pem")
Calculate SHA256 hash of some data
data = b"Hello, world!"
hash = hashlib.sha256(data).digest()
signature = crypto.sign(pkey, hash)
print("nSignature:")
print(crypto.dump_signing_parameters(crypto.FILETYPE_ASN1, signature))
This code loads the generated private key from a file, calculates the SHA256 hash of some data, and then signs the hash using the private key.
Verifying Signature
To verify the signature, we can use the following code:
from OpenSSL import crypto
Load public key from file
pubkey = crypto.load_publickey(crypto.FILETYPE_PEM, "public_key.pem")
Calculate SHA256 hash of some data
data = b"Hello, world!"
hash = hashlib.sha256(data).digest()
signature = crypto.sign(pkey, hash)
Verify signature using public key
result = crypto.verify(pubkey, hash + signature)
print("nSignature verification result:")
if result:
print("Signature is valid")
else:
print("Signature is invalid")
This code loads the generated public key from a file, calculates the SHA256 hash of some data, signs the hash using the private key, and then verifies the signature using the public key.
Creating Self-Signed Certificate
To create a self-signed certificate, we can use the following code:
from OpenSSL import crypto
import datetime
Generate X.509 certificate
cert = crypto.X509()
Set subject and issuer information
subject = cert.get_subject()
subject.C = "US"
subject.ST = "California"
subject.L = "San Francisco"
subject.O = "Example Inc."
subject.OU = "IT Department"
issuer = cert.get_issuer()
issuer.C = "US"
issuer.ST = "California"
issuer.L = "San Francisco"
issuer.O = "Example Inc."
issuer.OU = "IT Department"
Set notBefore and notAfter dates
not_before = datetime.datetime(2022, 1, 1)
not_after = datetime.datetime(2025, 12, 31)
Sign certificate with private key
cert.sign(pkey, crypto.sha256(), (2020, 9))
print("nCertificate:")
print(crypto.dump_certificate(crypto.FILETYPE_PEM, cert))
This code generates a new X.509 certificate, sets the subject and issuer information, sets the notBefore and notAfter dates, and then signs the certificate with the generated private key.
These are just a few examples of what you can do with OpenSSL using Python. There are many more features and functionalities available in the pyOpenSSL
library that we haven't covered here.