How to make a variable nonlocal in Python?
How to make a variable nonlocal in Python?
To make a variable nonlocal in Python, you can use the nonlocal
keyword within a function. Nonlocal variables are those that are defined outside of the function where they're being used, but are still accessible within it.
Here's an example:
def outer():
x = 10
def inner():
nonlocal x
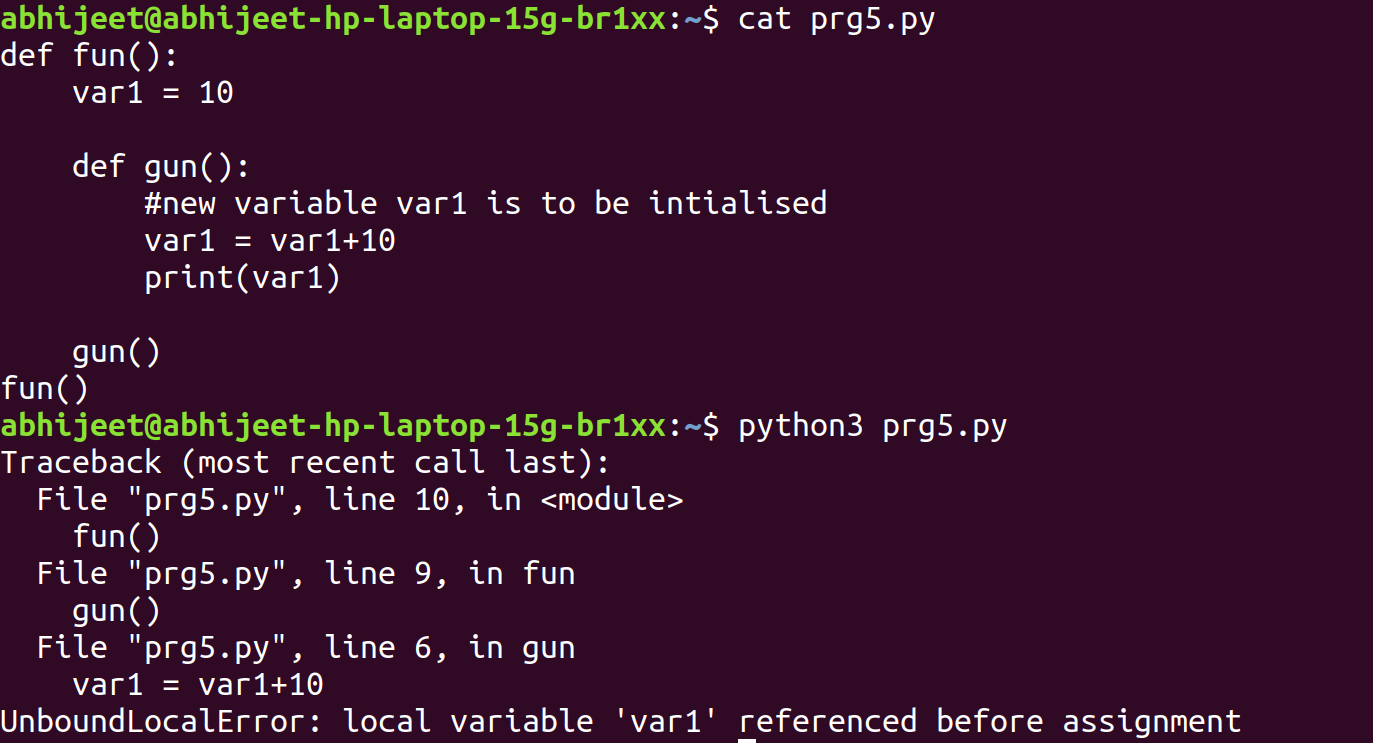
print("Inner function: ", x)
x = 20
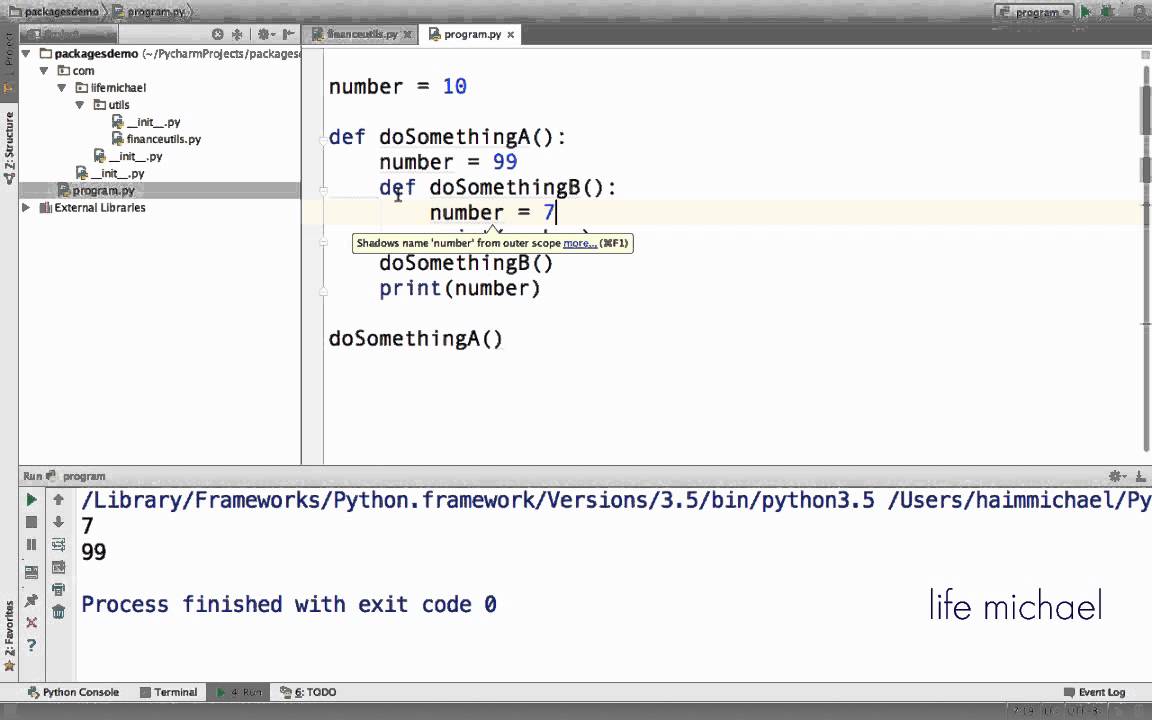
print("Inner function after modification: ", x)
inner()
print("Outer function: ", x)
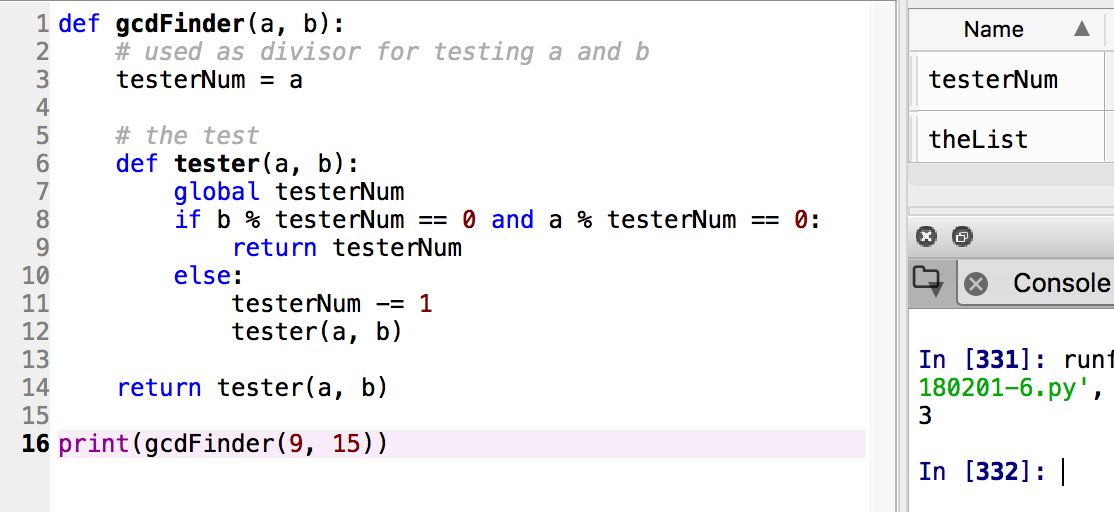
outer()
When you run this code, it will output:
Inner function: 10
Inner function after modification: 20
Outer function: 20
In the above example, x
is a nonlocal variable. The nonlocal
keyword allows the inner
function to modify the value of x
, which is defined in the outer
function.
Python's scope rules for variables are as follows:
Local variables are those that are defined inside a function. They can be accessed only within that function. Nonlocal variables are those that are defined outside the current function, but are still accessible within it. Global variables are those that are defined at the top-level of the module. They can be accessed from anywhere in the module.Here's an example using nonlocal variables:
x = 10
def outer():
x = 20
def inner():
nonlocal x
print("Inner function: ", x)
x = 30
print("Inner function after modification: ", x)
inner()
print("Outer function: ", x)
outer()
When you run this code, it will output:
Inner function: 20
Inner function after modification: 30
Outer function: 30
In the above example, x
is a nonlocal variable. The inner
function can modify the value of x
, which is defined in the outer
function.
To summarize:
Use thenonlocal
keyword to make variables nonlocal. Nonlocal variables are those that are defined outside the current function but are still accessible within it. Local variables are those that are defined inside a function and can be accessed only within that function. Global variables are those that are defined at the top-level of the module and can be accessed from anywhere in the module.
It's worth noting that while Python has nonlocal variables, they don't quite work like closures in languages such as Lisp or Scheme. Nonlocal variables allow you to modify variables defined outside the current function, whereas closures allow you to create functions that have access to variables defined in an outer scope.
How to combine multiple variables into one variable in Python?
Combining multiple variables into one variable is a common task in programming, and Python provides several ways to achieve this. Here are some methods:
List Comprehension: You can use list comprehension to create a new list that contains all the values from the individual variables. For example:x = 10
y = 20
z = 30
combined_variable = [x, y, z]
print(combined_variable) # Output: [10, 20, 30]
This creates a new list combined_variable
that contains the values of x
, y
, and z
.
x = 10
y = 20
z = 30
combined_variable = (x, y, z)
print(combined_variable) # Output: (10, 20, 30)
This creates a new tuple combined_variable
that contains the values of x
, y
, and z
.
x = 10
y = 20
z = 30
combined_variable = {'x': x, 'y': y, 'z': z}
print(combined_variable) # Output: {'x': 10, 'y': 20, 'z': 30}
This creates a new dictionary combined_variable
that contains the values of x
, y
, and z
as key-value pairs.
import collections
x = 10
y = 20
z = 30
combined_variable = collections.namedtuple('CombinedVariable', ['x', 'y', 'z'])(*[x, y, z])
print(combined_variable) # Output: CombinedVariable(x=10, y=20, z=30)
This creates a new named tuple combined_variable
that contains the values of x
, y
, and z
with explicit names.
import pandas as pd
x = 10
y = 20
z = 30
df = pd.DataFrame({'x': [x], 'y': [y], 'z': [z]})
print(df) # Output: x y z
0 10 20 30
import numpy as np
arr = np.array([x, y, z])
print(arr) # Output: [10 20 30]
These data structures provide more flexibility and functionality for working with combined variables.
In conclusion, there are several ways to combine multiple variables into one variable in Python. The choice of method depends on the specific use case, the structure of the data, and the level of complexity desired.