What are Python dictionary methods?
What are Python dictionary methods?
I'm happy to respond in English!
Python dictionaries offer several built-in methods that allow you to manipulate and analyze the key-value pairs contained within them. These methods enable you to perform various operations, such as adding new items, updating existing ones, deleting keys or values, and more. Here are some of the most commonly used Python dictionary methods:
dict.keys()
: Returns a view object that displays all the available dictionary's keys.
Example: d = {"a": 1, "b": 2, "c": 3}
; print(d.keys())
-> dict_keys(['a', 'b', 'c'])
dict.values()
: Returns a view object that displays all the values contained in the dictionary.
Example: d = {"a": 1, "b": 2, "c": 3}
; print(d.values())
-> dict_values([1, 2, 3])
dict.items()
: Returns a view object that displays all the key-value pairs in the dictionary.
Example: d = {"a": 1, "b": 2, "c": 3}
; print(d.items())
-> dict_items([('a', 1), ('b', 2), ('c', 3)])
dict.update()
: Updates the dictionary with the key-value pairs from another dictionary or an iterable of key-value pairs.
Example: d = {"a": 1, "b": 2}
; update_dict = {"c": 3, "d": 4};
d.update(update_dict)
-> `{ 'a': 1, 'b': 2, 'c': 3, 'd': 4}``
dict.pop()
: Removes the key-value pair with the specified key and returns its value if found.
Example: d = {"a": 1, "b": 2}
; print(d.pop("b"))
-> 2
, d -> {"a": 1}
dict.clear()
: Removes all items from the dictionary.
Example: d = {"a": 1, "b": 2}
; d.clear()
-> {}
, an empty dictionary
dict.copy()
: Returns a shallow copy of the dictionary.
Example: d = {"a": 1, "b": 2}
; copy_d = d.copy()
-> {"a": 1, "b": 2}
, a new dictionary that is identical to the original one
dict.fromkeys()
: Returns a new dictionary initialized with the specified keys and values.
Example: d = dict.fromkeys(["a", "b"], 0)
-> `{ 'a': 0, 'b': 0}``
These dictionary methods can significantly simplify your Python code by providing an efficient way to work with key-value pairs.
What is the __ dict __ method in Python?
The dict
method in Python! This might come as a surprise, but there isn't exactly a standalone method called dict
in Python. However, I assume you're asking about how to create and manipulate dictionaries (also known as associative arrays or hash maps) in Python.
In Python, the dict
type is an unordered collection of key-value pairs. The dict
constructor (or dict()
function) can be used to create a new empty dictionary:
my_dict = dict()
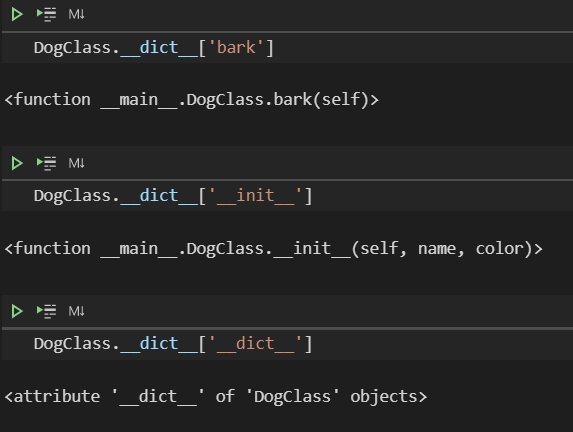
Or you can directly use the dictionary literal syntax:
my_dict = {}
A dictionary can store any immutable type, such as strings, integers, or other dictionaries. The values in a dictionary are not unique; there is no requirement that the keys be unique, although individual keys must be unique within the same dictionary.
Here's an example of how to use a dict
:
my_dict = {'name': 'John', 'age': 30}
print(my_dict['name']) # Output: John
print(my_dict['age']) # Output: 30
You can also create dictionaries using the dictionary comprehension syntax (introduced in Python 3.7):
my_dict = {'item' + str(i): i**2 for i in range(5)}
print(my_dict) # Output: {'item0': 0, 'item1': 1, 'item2': 4, 'item3': 9, 'item4': 16}
When you need to get the values from a dictionary, you can use the .values()
method:
my_dict = {'name': 'John', 'age': 30}
print(list(my_dict.values())) # Output: ['John', 30]
The dict
type has several other methods and operations. For example, you can iterate over a dictionary's key-value pairs using the .items()
method:
my_dict = {'name': 'John', 'age': 30}
for key, value in my_dict.items():
print(f"{key}: {value}")
Output:
name: John
age: 30
Or you can merge two dictionaries using the .update()
method:
dict1 = {'name': 'John', 'age': 30}
dict2 = {'country': 'USA', 'city': 'New York'}
dict1.update(dict2)
print(dict1) # Output: {'name': 'John', 'age': 30, 'country': 'USA', 'city': 'New York'}
In summary, the dict
type in Python is an unordered collection of key-value pairs. You can create dictionaries using various methods and manipulate them through their various methods and operations.
I hope this explanation has been helpful!