How do you join an object in Python?
How do you join an object in Python?
In Python, there are several ways to join objects together into a single entity. The most common methods for joining strings and lists are described below.
String Concatenation: You can use the '+' operator or the str.format() method to concatenate two or more strings:string1 = "Hello"
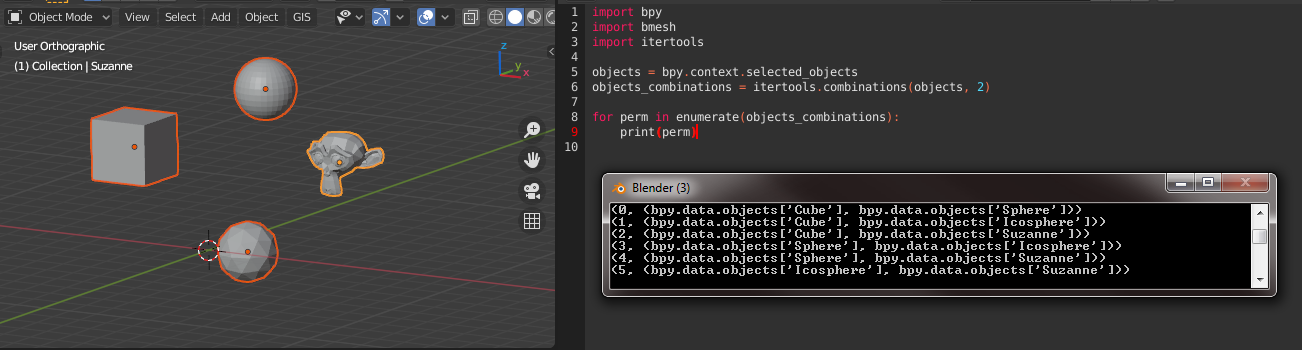
string2 = "World!"
new_string = string1 + " " + string2
print(new_string) # Output: Hello World!
Alternatively, you can use the str.format() method:
name = "John"
age = 25
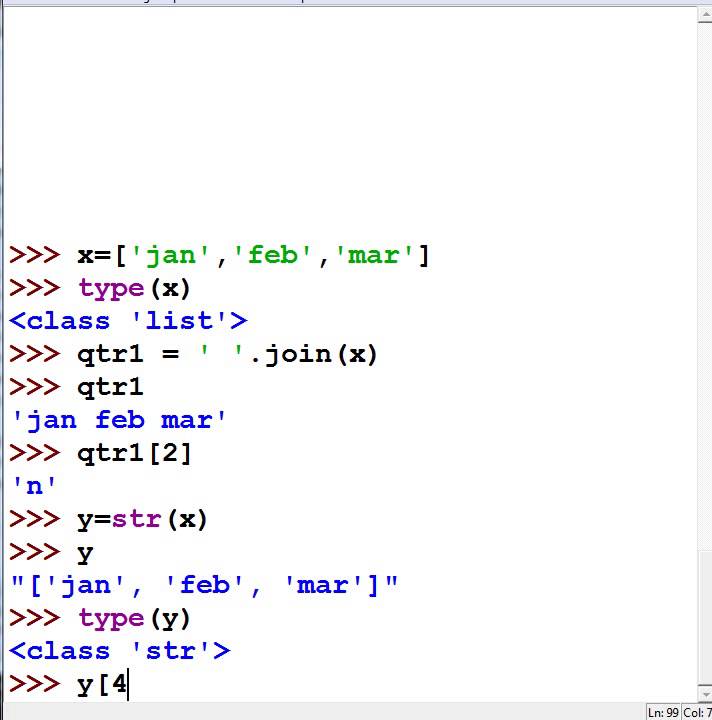
new_string = "{} is {} years old.".format(name, age)
print(new_string) # Output: John is 25 years old.
strings_list = ["apple", "banana", "cherry"]
new_string = ", ".join(strings_list)
print(new_string) # Output: apple, banana, cherry
List Joining: If you have lists of objects (e.g., strings, integers), you can use the extend() method or list comprehension to concatenate them:
list1 = ["a", "b", "c"]
list2 = ["d", "e", "f"]
list1.extend(list2)
print(list1) # Output: ['a', 'b', 'c', 'd', 'e', 'f']
Alternatively, you can use list comprehension:
list1 = ["a", "b", "c"]
list2 = ["d", "e", "f"]
new_list = [item for sublist in (list1, list2) for item in sublist]
print(new_list) # Output: ['a', 'b', 'c', 'd', 'e', 'f']
Tuple Joining: You can use the + operator to concatenate tuples:
tuple1 = ("apple", "banana")
tuple2 = ("cherry", "date")
new_tuple = tuple1 + tuple2
print(new_tuple) # Output: ('apple', 'banana', 'cherry', 'date')
Dictionary Joining (Key-Value Merging): You can merge two dictionaries into one using the ** operator:
dict1 = {"name": "John", "age": 25}
dict2 = {"city": "New York", "country": "USA"}
new_dict = {**dict1, **dict2}
print(new_dict) # Output: {'name': 'John', 'age': 25, 'city': 'New York', 'country': 'USA'}
When joining objects in Python, it is essential to consider the type and structure of the data you are working with. Understanding how to join objects effectively will help you write more efficient and readable code.
Python join list to string
I'd be happy to help you with that!
To join a list of strings into one single string in Python, you can use the join()
function. This function is part of the str
class and returns a new string made by concatenating all the elements in the input iterable (like a list or tuple) with the character given as an argument.
Here's an example:
my_list = ["Hello", "world", "this", "is", "a", "test"]
seperator = "-"
result = seperator.join(my_list)
print(result)
When you run this code, it will output: Hello-world-this-is-a-test
. The elements in the list are joined together with the specified separator (in this case, a hyphen -
) to form a single string.
Here's another example:
fruits = ["apple", "banana", "cherry"]
seperator = ", "
result = seperator.join(fruits)
print(result)
Running this code will output: apple, banana, cherry
. The elements in the list are joined together with commas to form a single string.
Note that if your list contains elements that aren't strings (like integers or booleans), you'll get an error when trying to join them. It's always a good idea to check if all elements in the list are strings before calling join()
:
my_list = [1, 2, 3, "four", 5, 6]
for element in my_list:
if not isinstance(element, str):
print("List contains non-string elements!")
break
else:
result = "-".join(map(str, my_list))
print(result)
In this example, the code will output 1-2-3-four-5-6
, as all elements are converted to strings before joining.
Remember that the join()
function returns a new string made by concatenating the input iterable. It does not modify the original list or its elements.