What is a bitwise operator in Python?
What is a bitwise operator in Python?
In Python, bitwise operators are used to manipulate the binary representation of integers. Bitwise operators perform operations on the bits that make up an integer, allowing you to perform bit-level operations on the data.
Python supports several bitwise operators:
&
(Bitwise AND): This operator compares each bit of the first operand to the corresponding bit of the second operand. If both bits are 1, the corresponding result bit is set to 1. Otherwise, the result bit is set to 0.
Example: 5 & 3
= 1
because in binary representation, 5
is 101
and 3
is 011
, so the resulting number will be 001
which is 1
.
|
(Bitwise OR): This operator compares each bit of the first operand to the corresponding bit of the second operand. If either or both bits are 1, the corresponding result bit is set to 1.
Example: 5 | 3
= 7
because in binary representation, 5
is 101
and 3
is 011
, so the resulting number will be 111
which is 7
.
~
(Bitwise NOT): This operator has the effect of flipping all bits. In other words, it gives the one's complement of a number.
Example: ~5
= -6
because in binary representation, 5
is 101
, so the resulting number will be 010
which is -2
in decimal (since Python uses negative integers to represent two's complement).
^
(Bitwise XOR): This operator compares each bit of the first operand to the corresponding bit of the second operand. If the bits are not the same, the corresponding result bit is set to 1.
Example: 5 ^ 3
= 6
because in binary representation, 5
is 101
and 3
is 011
, so the resulting number will be 110
which is 6
.
<<
(Left Shift): This operator shifts the bits of a number to the left by the specified number of positions. It fills 0 on the right side.
Example: 5 << 1
= 10
because in binary representation, 5
is 101
, shifting it one position to the left will make it 0101
.
>>
(Right Shift): This operator shifts the bits of a number to the right by the specified number of positions. It fills 0 on the left side.
Example: 10 >> 1
= 5
because in binary representation, 10
is 1010
, shifting it one position to the right will make it 0101
.
These bitwise operators are useful for performing various operations such as setting or clearing specific bits in a number, checking if a bit is set, and more. They can be used in various areas of programming such as working with flags, manipulating binary data, and more.
It's worth noting that the use of bitwise operators can make your code more efficient by avoiding unnecessary calculations or operations, but they should be used judiciously and only when necessary to avoid making the code harder to understand.
What does >> do in Python?
The >>>
symbol is not a special command in Python itself, but rather an indication that the code has been entered into an interactive shell or interpreter.
When you enter a line of code followed by >>>
and press Enter, you are essentially submitting your code to the Python interpreter for execution. The >>>
prompt indicates that you have reached the end of the input line and are waiting for the interpreter's response.
Here are some examples of what you can do with >>>
in a Python interactive shell:
>>> 2 + 2
4
math.sqrt(16)
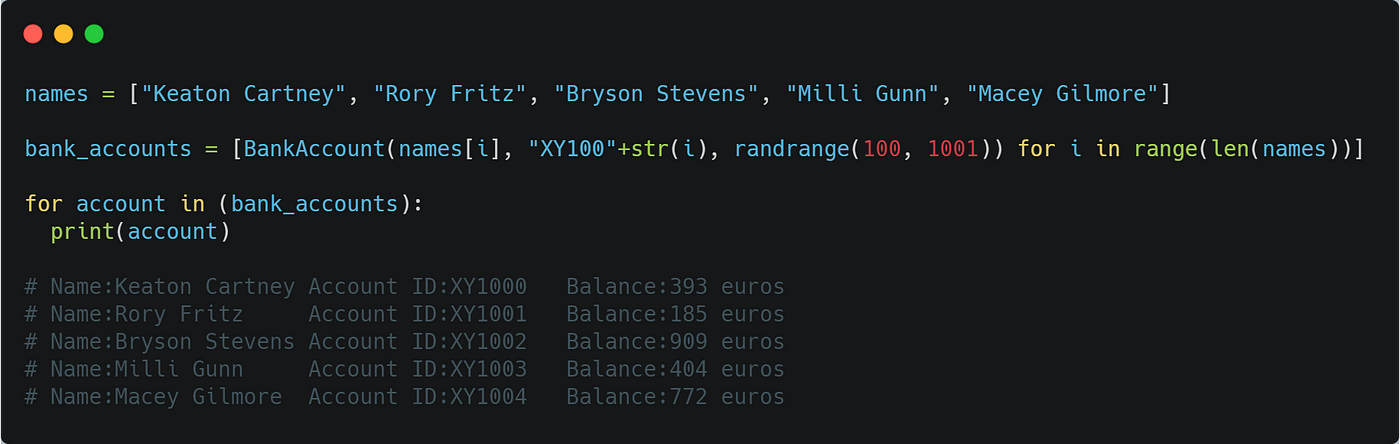
4.0
Define variables and functions: You can assign values to variables or define new functions using the =
operator or the def
keyword.
>>> x = 5
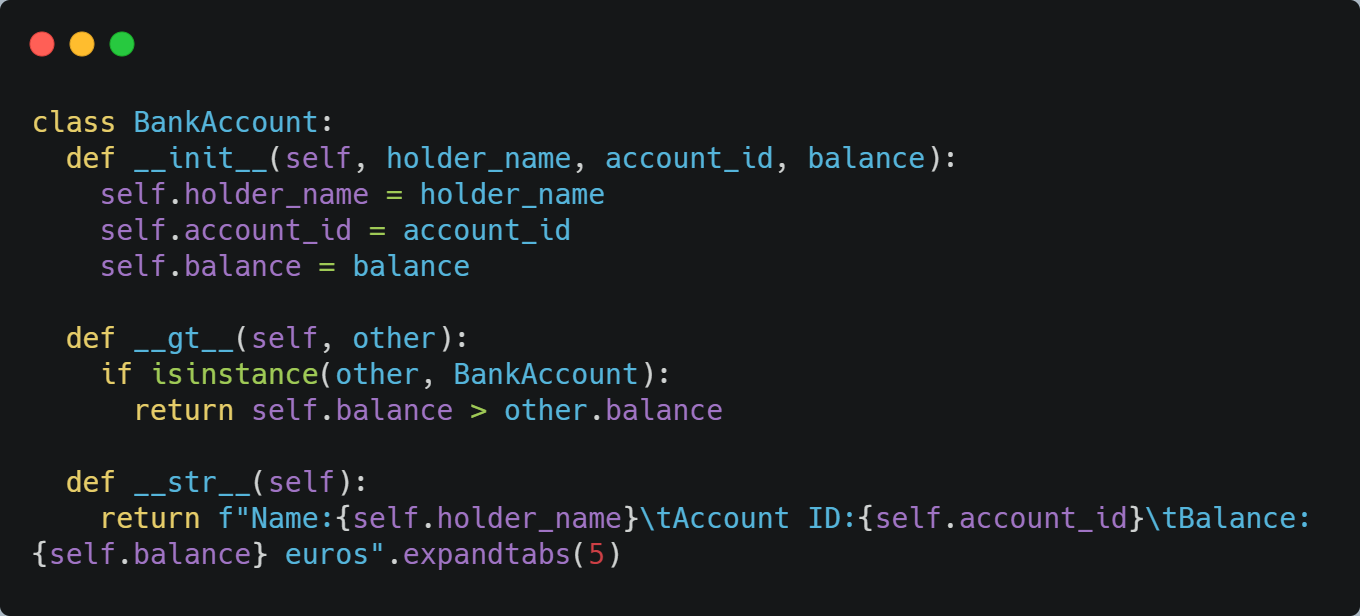
def greet(name):
... print(f"Hello, {name}!")
...
Run Python code files: If you want to execute a Python file from within the interactive shell, you can use the import
statement followed by the filename (without the .py
extension).
>>> import mymodule
Inspect data structures: You can use the dir()
or vars()
functions to examine the attributes and values of variables or objects.
>>> dir(mymodule)
['doc', 'loader', 'name', 'package', 'spec']
vars(x)
{'x': 5}
In summary, >>>
is not a command in Python itself but rather an indication that you are interacting with the interpreter. It allows you to evaluate expressions, define variables and functions, run code files, inspect data structures, and more.
In a real-world scenario, you would typically use an integrated development environment (IDE) or a text editor to write and execute your Python code. However, the interactive shell can be useful for exploratory coding, testing, or debugging, especially when working with complex data structures or trying out new ideas quickly.