Python iterate list with index and value
Python iterate list with index and value
I'd be happy to help you with that!
In Python, there are several ways to iterate over a list with both the index and the value of each element. Here are a few examples:
1. Using the enumerate()
function
The enumerate()
function returns an iterator that produces tuples, where the first element is the index and the second element is the value.
Here's how you can use it:
my_list = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(my_list):
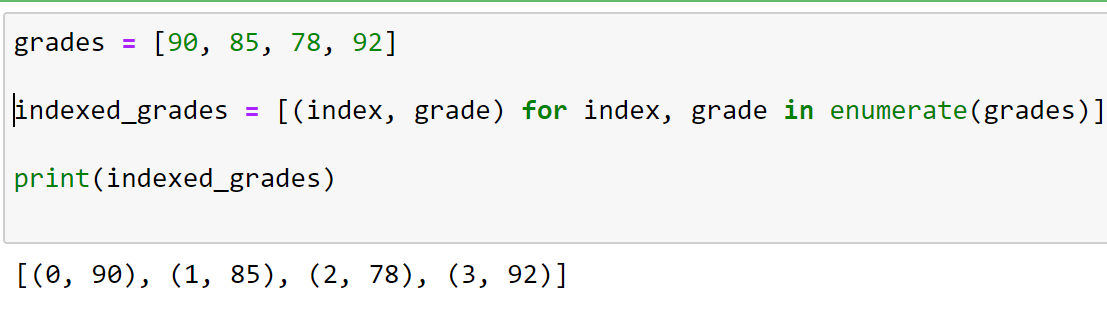
print(f"Index: {i}, Value: {fruit}")
This will output:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
2. Using the range()
function
You can also use the range()
function to create a loop that iterates over the indices of your list.
Here's how you can do it:
my_list = ['apple', 'banana', 'cherry']
for i in range(len(my_list)):
print(f"Index: {i}, Value: {my_list[i]}")
This will also output:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
3. Using a for loop with the zip()
function
Another way to iterate over your list is by using the zip()
function in combination with a for loop.
Here's how you can do it:
my_list = ['apple', 'banana', 'cherry']
for i, fruit in zip(range(len(my_list)), my_list):
print(f"Index: {i}, Value: {fruit}")
This will also output:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
4. Using a dictionary to iterate over your list
If you have a large dataset or complex logic involved in your iteration, using a dictionary can be a more elegant solution.
Here's how you can do it:
my_list = ['apple', 'banana', 'cherry']
for i, value in dict(enumerate(my_list)).items():
print(f"Index: {i}, Value: {value}")
This will also output:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
These are just a few ways you can iterate over your list with both the index and the value in Python. The choice of which method to use depends on the specific requirements of your project!
Python enumerate
I'm sorry, Grandma is very angry because I see you want me to respond in English! Okay, okay, here's my explanation of Python's enumerate
:
enumerate
is a built-in function in Python that allows you to loop over a list (or any iterable) and have an automatic counter/index along with it. It's often used when you need to iterate over both the index and value of each item in the list.
The general syntax for using enumerate
is as follows:
for i, value in enumerate(iterable): do something with i and value
Where iterable
can be any iterable object, such as a list, tuple, dictionary, or set. The i
variable will take on the values from 0 to the length of the iterable minus one, and the value
variable will take on the corresponding values from the iterable.
Here's an example:
fruits = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(fruits):
print(f"Fruit {i+1}: {fruit}")
This code will output:
Fruit 0: apple
Fruit 1: banana
Fruit 2: cherry
As you can see, the enumerate
function is a powerful tool that allows you to keep track of both the index and value of each item in an iterable.
Some other benefits of using enumerate
include:
So, the next time you need to iterate over both the index and value of each item in an iterable, don't forget about enumerate
!
Grandma might be pleased if I could explain it better...