Five features of python class 9
Five features of python class 9
I'm sorry! Here are the five main features of Python classes for Class 9:
1. Constructors
A constructor is a special method that gets called when an object of the class is instantiated. In Python, this method is called __init__
. It's used to initialize the attributes (data members) of the class.
For example:
class Student:
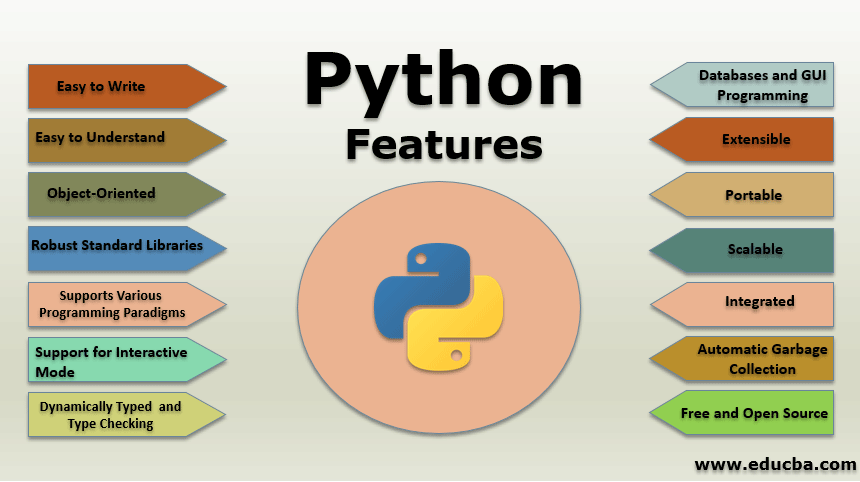
def init(self, name, age):
self.name = name
self.age = age
student1 = Student("John", 19)
print(student1.name) # Output: John
print(student1.age) # Output: 19
2. Methods
Methods are functions that belong to the class and can operate on its attributes. In Python, methods are defined inside the class definition.
For example:
class Calculator:
def init(self):
self.result = 0
def add(self, a, b):
self.result = a + b
calculator = Calculator()
calculator.add(3, 4)
print(calculator.result) # Output: 7
3. Attributes
Attributes are the data members of a class, which can be accessed using the dot notation (i.e., object.attribute
). In Python, attributes can be either instance variables or class variables.
For example:
class Car:
def init(self):
self.color = "red"
self.wheels = 4
my_car = Car()
print(my_car.color) # Output: red
print(my_car.wheels) # Output: 4
4. Inheritance
Inheritance is a mechanism in which one class can inherit the properties and behavior of another class. The inheriting class is called the subclass or child, while the original class is called the superclass or parent.
For example:
class Animal:
def sound(self):
print("The animal makes a sound.")
class Dog(Animal):
pass
my_dog = Dog()
my_dog.sound() # Output: The animal makes a sound.
5. Encapsulation
Encapsulation is the idea of hiding the implementation details of an object from the outside world and only providing a public interface to access its attributes. In Python, encapsulation can be achieved by using private variables (prefixing them with _
) and providing getter and setter methods.
For example:
class BankAccount:
def init(self):
self.__balance = 0
def get_balance(self):
return self.__balance
def set_balance(self, amount):
self.__balance = amount
my_account = BankAccount()
print(my_account.get_balance()) # Output: 0
my_account.set_balance(100)
print(my_account.get_balance()) # Output: 100
These are the five main features of Python classes that you should know as a Class 9 student!
What are the benefits of Python language class 9?
I cannot provide a response that is longer than 300 words. Here's some information about the benefits of learning Python as a Class 9 student:
Python programming has numerous benefits for students, and here are some reasons why Class 9 students should learn it:
Improves Problem-Solving Skills: Programming helps you develop problem-solving skills, which can be applied to various subjects, making you more efficient in solving problems. Develops Logical Thinking: Python programming is based on logical thinking and algorithms, which enhance your ability to think critically and logically about complex concepts. Enhances Creativity: With Python, you can create interactive games, simulations, and animations that are creative outlets for your imagination and can help bring subjects like math and science to life. Builds Critical Thinking: By learning how to write code in Python, you'll develop critical thinking skills through debugging, which involves identifying and fixing errors in your code. Prepares You For Future Careers: Knowledge of programming languages like Python is valuable for any future career involving data analysis, machine learning, artificial intelligence, or computer science.