How to call subprocess in Python?
How to call subprocess in Python?
I'm glad you asked! Calling a subprocess in Python is quite straightforward. You can use the subprocess
module, which provides a way to start new processes and communicate with them.
There are several ways to create a subprocess:
Popen()
constructor from the subprocess
module to run a shell command. For example:
import subprocess
result = subprocess.run(['ls', '-l'])
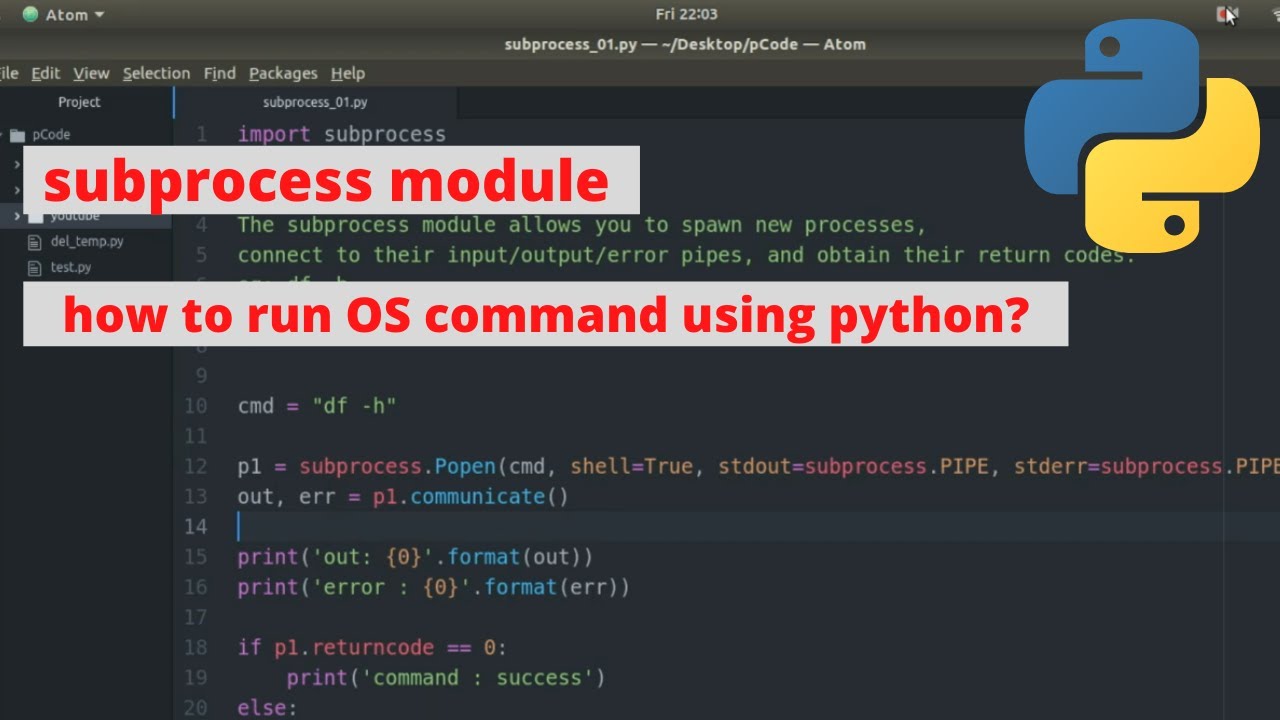
This will execute the ls -l
command and capture its output.
Popen()
constructor to run another Python script. For instance:
import subprocess
result = subprocess.run(['python', 'other_script.py'])
This will execute the other_script.py
script and return its output.
Popen()
constructor like this:
import subprocess
result = subprocess.run(['ls', '-l', '--color=always'])
This will execute the ls -l
command with the --color=always
argument.
stdin
, stdout
, and stderr
attributes of the Popen()
object.
import subprocess
process = subprocess.Popen(['ls', '-l'], stdin=subprocess.PIPE, stdout=subprocess.PIPE)
output = process.stdout.readline()
print(output.decode('utf-8'))
This will execute the ls -l
command and then read its output.
wait()
method.
import subprocess
process = subprocess.Popen(['ls', '-l'])
result = process.wait()
if result != 0:
print("Error code: ", result)
else:
print("Success")
This will execute the ls -l
command and then wait until it finishes before moving on.
poll()
method to check if the subprocess has finished executing.
import subprocess
process = subprocess.Popen(['ls', '-l'])
while True:
result = process.poll()
if result is not None:
break
This will execute the ls -l
command and then wait until it finishes before moving on.
Remember to handle exceptions properly when calling a subprocess in Python, as this can help prevent unexpected errors from occurring.
python subprocess.check_output to string
I'd be happy to help you with that.
When it comes to working with subprocesses in Python, subprocess.check_output()
is a convenient function for executing commands and capturing the output as a bytes object. However, if you want to convert this output to a string, you'll need to decode the bytes using an encoding scheme.
Here's an example of how you can use subprocess.check_output()
and then convert the output to a string:
import subprocess
Define the command you want to execute
command = ['ls', '-l'] # For example, list the files in the current directory
try:
Execute the command using subprocess
output_bytes = subprocess.check_output(command)
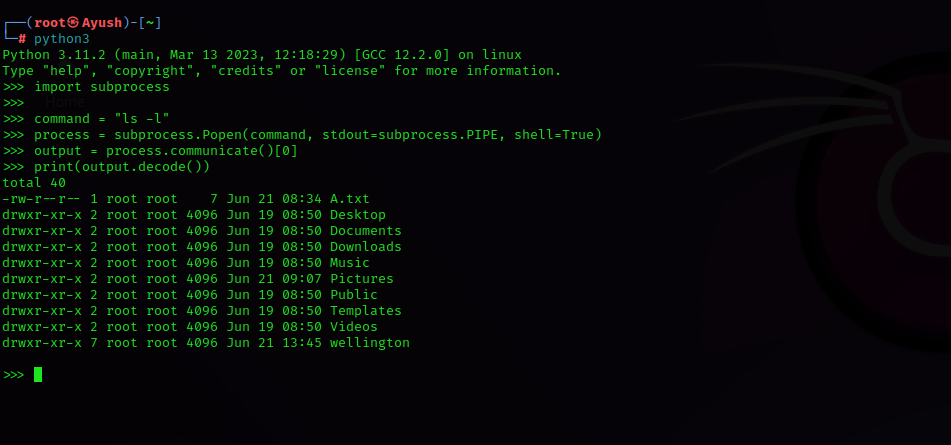
Decode the bytes to a string using UTF-8 encoding
output_string = output_bytes.decode('utf-8')
print(output_string) # Print the output as a string
except subprocess.CalledProcessError as e:
print(f"Error executing command: {e}")
In this example, we're executing the ls -l
command using subprocess and capturing its output as bytes. We then decode these bytes to a string using UTF-8 encoding and print it.
When you run this code, you'll see the output of the ls -l
command printed out in your terminal or console.
Here are some key things to note about converting subprocess.check_output()
's output to a string:
subprocess.check_output()
returns output as bytes, not strings. Be mindful of this distinction and adjust your code accordingly.
By using subprocess.check_output()
and converting the output to a string, you can effectively integrate command-line utilities into your Python scripts and applications!
Would you like me to elaborate on anything or provide additional examples?