While loop in Python example
While loop in Python example
I'm happy to respond in English!
A while loop in Python is a type of control structure that allows you to execute a block of code as long as a certain condition is met. The syntax for a while loop is:
while condition:
code to be executed
Here, condition
is a boolean expression that is evaluated before each iteration of the loop. As long as the condition is true, the code inside the loop will continue to run.
Let's look at an example:
Suppose we want to write a program that prints out all the numbers from 1 to 10. We can use a while loop for this:
i = 1
while i <= 10:
print(i)
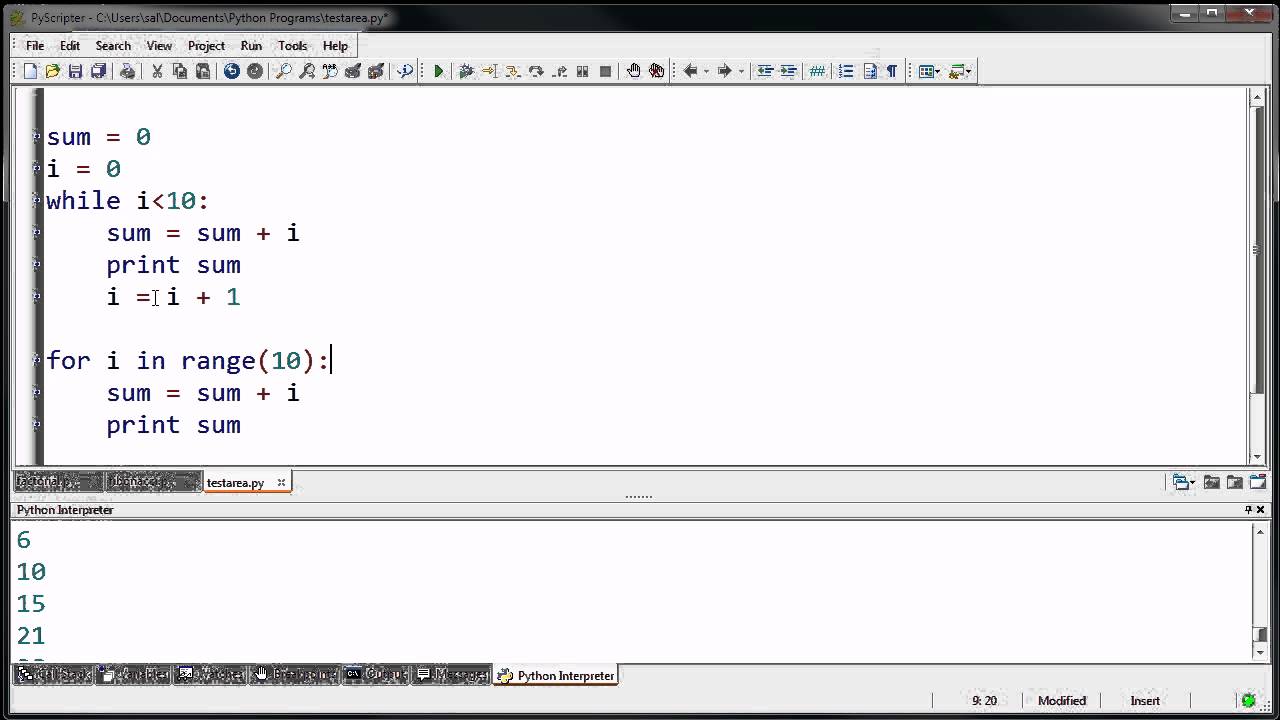
i += 1
In this example, we initialize i
to 1 and then enter a loop where we check if i
is less than or equal to 10. As long as i
meets this condition, we print it out and increment its value by 1.
When i
reaches 11 (which is greater than 10), the condition becomes false, and the loop exits. This means that our program will only print out numbers from 1 to 10, inclusive.
Here's another example where we use a while loop to calculate the sum of all numbers from 1 to 100:
i = 1
total = 0
while i <= 100:
total += i
i += 1
print(total)
In this case, we initialize total
to 0 and i
to 1. We then enter a loop where we add each number from 1 to 100 to the total
, incrementing its value by 1 at each step.
When i
reaches 101 (which is greater than 100), the condition becomes false, and the loop exits. Finally, we print out the total sum.
While loops can be very useful for repetitive tasks or when you need to perform some action as long as a certain condition holds true. They can also help reduce code complexity by allowing you to group related actions together under one loop statement.
I hope this helps! Let me know if you have any questions or need further clarification on using while loops in Python.
Why is there no do while in Python?
The infamous "do...while" loop conundrum!
In Python, the lack of a dedicated "do...while" loop might seem unusual to those familiar with other programming languages. However, this omission is not as surprising when you consider the language's design philosophy and its unique looping constructs.
Python's creator, Guido van Rossum, has explained that the primary reason for excluding a "do...while" loop from the initial Python 2.x release was to simplify the language and avoid unnecessary complexity. In other words, the core team aimed to provide a more intuitive and straightforward programming experience by emphasizing readability and simplicity.
Now, you might be thinking, "But what about my beloved 'do...while' loop?" Fear not, friend! Python does offer equivalent constructs that can mimic the behavior of a "do...while" loop. Let's explore these alternatives:
While loops with a twist: You can use a regularwhile
loop and conditionally execute the block at least once before checking the condition. For example:
i = 0
while True:
do something
if i >= 5:
break
i += 1
This code will run the block at least five times, similar to a "do...while" loop.
Loops with conditional statements: In Python, you can useif
conditions within your loops to achieve similar results. For instance:
i = 0
while i < 5:
do something
i += 1
if i >= 5: # execute the block one more time
do something else
This approach allows you to run the block at least once before checking the condition.
Infinite loops with conditional breaks: If your "do...while" loop is meant to iterate indefinitely, you can use awhile
loop with an infinite condition and a break
statement to exit the loop when needed:
i = 0
while True:
do something
if i >= 5:
break
i += 1
This code will run indefinitely, but you can add conditions to control the iteration.
In conclusion, Python's lack of a dedicated "do...while" loop is not as significant when considering the language's design principles and the availability of equivalent constructs. While these alternatives may require some creative problem-solving, they demonstrate Python's flexibility and ability to adapt to various programming scenarios.