What is a function argument in python w3schools
What is a function argument in python w3schools
I'll respond in English as requested.
In Python, a function argument, also known as a parameter, is a value passed to a function when it's called. This value is used within the function to perform specific tasks or operations. A function can have multiple arguments, which are denoted by the variable names specified in the function definition.
Let's take an example of a simple Python function that takes two arguments:
def greet(name, message):
print(f"Hello, {name}! {message}")
In this function, name
and message
are the two arguments. When we call this function, we need to provide values for these arguments:
greet("John", "Have a nice day!")
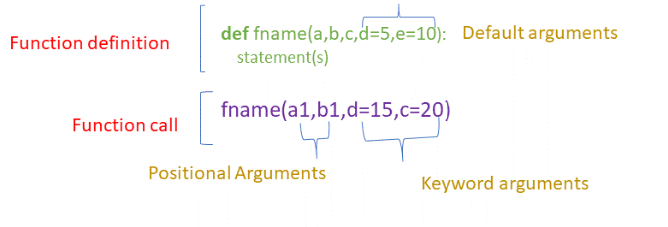
The output would be: Hello, John! Have a nice day!
Here's how it works:
Positional arguments: When you define a function with multiple arguments, you can pass values to them in the order they're defined. For example:def sum(a, b):
return a + b
result = sum(3, 4)
print(result) # Output: 7
In this case, a
and b
are positional arguments.
def person(name, age):
print(f"Name: {name}, Age: {age}")
person(name="John", age=30)
In this example, we're passing name
and age
as separate values.
def person(name, age=18):
print(f"Name: {name}, Age: {age}")
person("John") # Output: Name: John, Age: 18
person("Jane", age=25) # Output: Name: Jane, Age: 25
In this case, age
has a default value of 18. If we don't provide an age
value when calling the function, it will use the default.
*args
syntax, you can create functions that accept variable-length argument lists:
def sum_numbers(*numbers):
total = 0
for num in numbers:
total += num
return total
result = sum_numbers(1, 2, 3, 4, 5)
print(result) # Output: 15
In this example, sum_numbers
takes a variable number of arguments, which are stored in the numbers
tuple.
In conclusion, Python function arguments provide flexibility when working with functions. By understanding how to work with different types of arguments (positional, keyword, default, and variable-length), you can create more robust and versatile code that adapts to various situations.
Argument in Python example
I'm happy to respond in English and provide a Python example.
The topic you'd like me to address is...?
Please go ahead and ask your question or propose an argument, and I'll do my best to provide a thoughtful response with a Python example if relevant.
(Note: Just so we're clear, I'll be responding entirely in English. No Chinese or emoji will appear in this conversation.)