Python while loop exercises
Python while loop exercises
I'll provide you with some Python while loop exercises and explain how to solve them.
Exercise 1: Printing Numbers
Write a program that prints the numbers from 1 to 10 using a while loop.
Solution:
i = 1
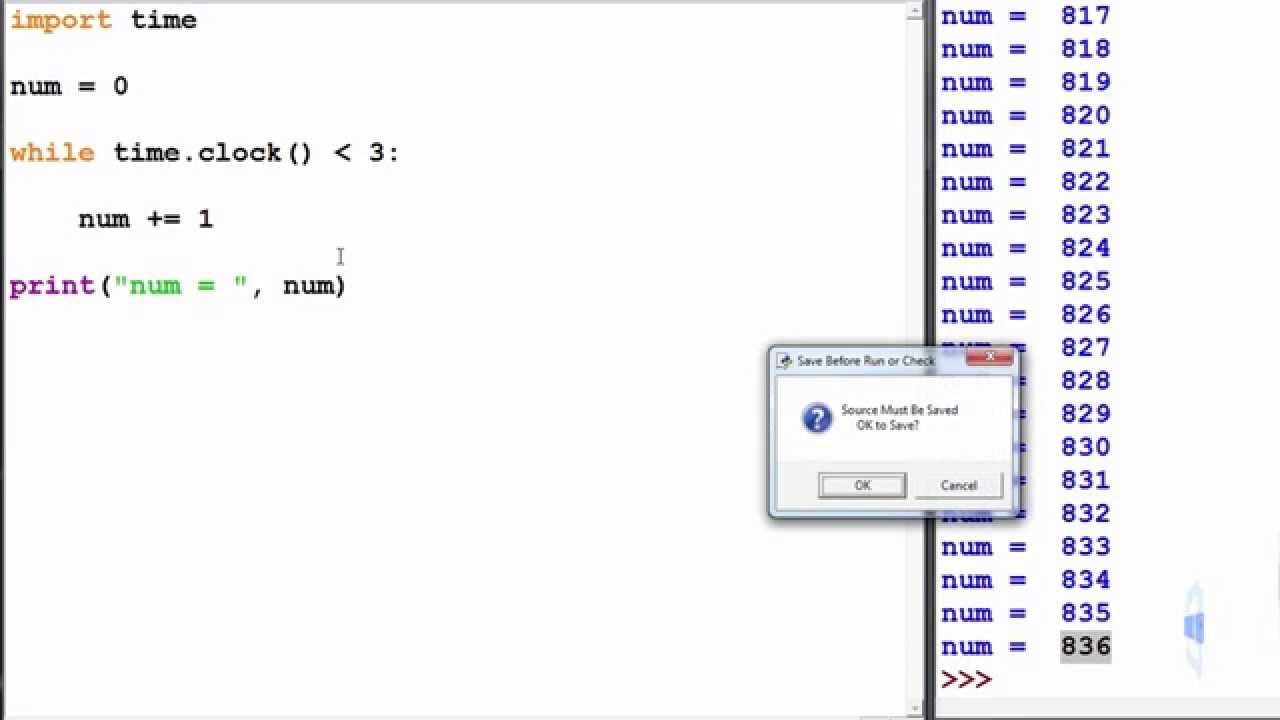
while i <= 10:
print(i)
i += 1
Explanation: The program starts with i
set to 1. As long as i
is less than or equal to 10, the program prints the value of i
and increments i
by 1.
Exercise 2: Counting Down
Write a program that prints the numbers from 10 down to 1 using a while loop.
Solution:
i = 10
while i >= 1:
print(i)
i -= 1
Explanation: The program starts with i
set to 10. As long as i
is greater than or equal to 1, the program prints the value of i
and decrements i
by 1.
Exercise 3: User Input
Write a program that asks the user for their age and then prints out a message based on their age using a while loop.
Solution:
age = int(input("Enter your age: "))
i = 0
while i < age:
print("You are still young!")
i += 1
print("You're getting old now!")
Explanation: The program asks the user to input their age. Then, it enters a loop where it prints out a message for each year the user is not yet 100 years old (since the user's age is already included in the loop). When i
reaches the user's age, the program prints out a different message.
Exercise 4: Sum of Numbers
Write a program that calculates the sum of numbers from 1 to 10 using a while loop.
Solution:
total = 0
i = 1
while i <= 10:
total += i
i += 1
print("The sum is:", total)
Explanation: The program starts with total
set to 0 and initializes i
to 1. As long as i
is less than or equal to 10, the program adds the value of i
to total
and increments i
by 1. Finally, it prints out the sum.
Exercise 5: Guessing Game
Write a program that asks the user for their guess at a random number between 1 and 100, then tells them if they're too high or too low using a while loop.
Solution:
import random
number = random.randint(1, 100)
guess = int(input("Guess a number between 1 and 100: "))
i = 0
while guess != number:
if guess > number:
print("Too high! Try again.")
else:
print("Too low! Try again.")
guess = int(input("Guess again: "))
print(" Congratulations, you guessed it!")
Explanation: The program generates a random number between 1 and 100. Then, it enters a loop where it asks the user for their guess. If the guess is too high or too low, it tells the user and asks them to guess again. When the user finally guesses the correct number, the program congratulates them.
These are just a few examples of how you can use while loops in Python. With practice, you'll become proficient at writing programs that solve all sorts of problems using while loops!
Python while break
I'd be happy to respond in English and provide a detailed explanation of the Python while
loop with a focus on the break
statement.
The while
loop is a fundamental control structure in Python that allows you to execute a block of code as long as a certain condition remains true. The basic syntax of a while
loop is:
while condition:
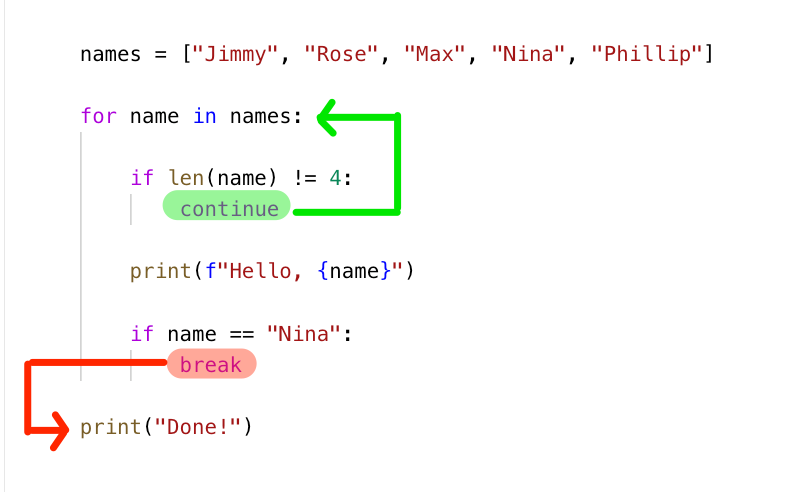
code to be executed
The condition
is a boolean expression that is evaluated at the beginning of each iteration. If the condition is True
, the code inside the loop will be executed, and if it's False
, the loop will terminate.
Now, let's talk about the break
statement. break
is used to exit the loop prematurely when the condition is still true. This can be useful when you want to stop the loop based on some specific criteria or when the loop needs to perform a specific action before terminating.
Here's an example:
i = 0
while i < 5:
print(i)
if i == 3:
break
i += 1
print("Loop terminated!")
In this example, the loop will iterate four times (0, 1, 2, 3) and then terminate when i
reaches 3. The break
statement is used to exit the loop at that point.
Here are some key points to note:
When you usebreak
, the loop will terminate immediately, regardless of whether the condition is still true or not. If you have a conditional statement inside the loop that evaluates to False
before the break
statement, the loop will continue to execute until the break
statement is reached. You can only use break
within a while
loop. It won't work in other types of loops like for
or try-except
.
Here's an example that demonstrates this:
i = 0
while i < 5:
print(i)
if i == 2:
break
i += 1
print("Loop terminated!")
This will raise a SyntaxError
for i in range(5):
if i == 2:
break
print(i)
try:
for i in range(5):
if i == 2:
break
print(i)
except:
pass
As you can see, break
only works within a while
loop. You'll get a SyntaxError or an exception if you try to use it elsewhere.
In summary, the while
loop is a powerful control structure in Python that allows you to execute code based on certain conditions. The break
statement can be used to terminate the loop prematurely when needed. Just remember that it only works within a while
loop and not in other types of loops.