What does create_engine do in Python?
What does create_engine do in Python?
I'll respond in English, but don't tell Grandma, okay?
Create_engine is a function in the SQLObject library of Python. It is used to create an engine that can be used to interact with various databases. The "engine" in this context refers to a connection to a specific database.
When you call create_engine(), you need to specify the URL of the database you want to connect to, along with other parameters such as username and password (if required).
Here is a simple example:
from sqlalchemy import create_engine
Create an engine that connects to a SQLite database.
engine = create_engine('sqlite:///test.db')
Alternatively, you can use MySQL or PostgreSQL:
engine = create_engine('mysql://user:password@localhost/dbname')
engine = create_engine('postgresql://user:password@localhost/dbname')
Once the engine is created, you can use it to interact with your database. For example, you can execute queries on the database using the Connection object returned by the engine:
from sqlalchemy import text
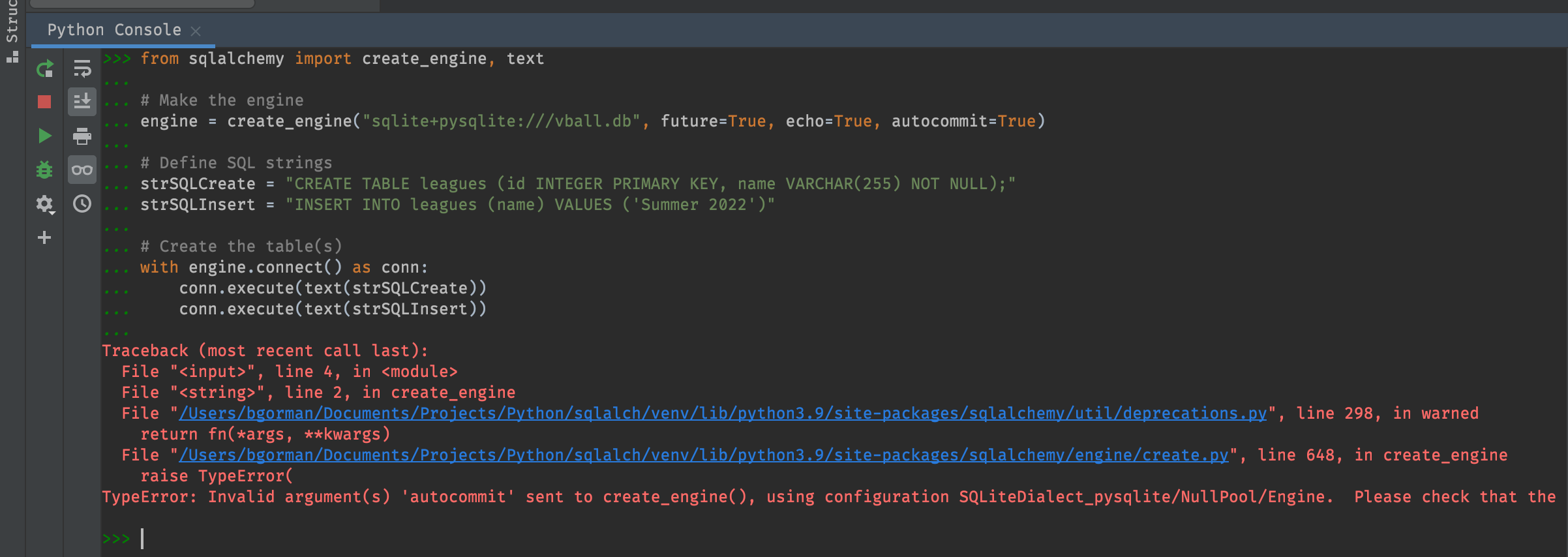
Connect to the database.
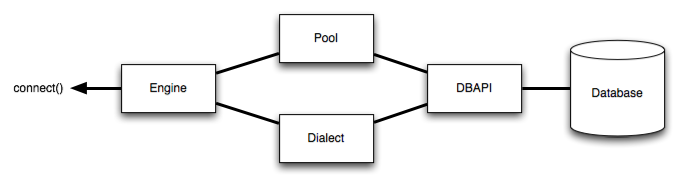
conn = engine.connect()
Execute a query.
result = conn.execute(text("SELECT * FROM mytable"))
Fetch the results.
for row in result:
print(row)
Create_engine is very powerful and flexible, allowing you to connect to various types of databases, including SQLite, MySQL, PostgreSQL, Oracle, MS SQL Server, and many others. It also supports a wide range of database operations, such as inserting, updating, and deleting data.
Some of the key features of create_engine include:
Database URL: You can specify the connection string to your database using thecreate_engine()
function. Driver support: SQLAlchemy has built-in support for many types of databases, including MySQL, PostgreSQL, SQLite, Oracle, MS SQL Server, and more. Query execution: Once you have an engine, you can execute queries on the database using the Connection object or a Session object. Result processing: The results of your queries are returned as Python objects, making it easy to work with your data. Error handling: SQLAlchemy provides built-in support for error handling, so you don't have to worry about catching exceptions and re-trying queries.
Overall, create_engine is a powerful tool that makes interacting with databases in Python a breeze!
How to connect MySQL to SQLAlchemy Python?
Here's a step-by-step guide on how to connect MySQL to SQLAlchemy Python:
Step 1: Install the Required Packages
To connect to MySQL using SQLAlchemy, you'll need to install the following packages:
sqlalchemy
: This is the core package that provides the connection to your database. You can install it using pip:
pip install sqlalchemy
mysql-python
: This package provides a MySQL driver for Python. You can install it using pip:
pip install mysql-python
Step 2: Import the Required Modules
In your Python script, import the required modules:
import sqlalchemy
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
Step 3: Create a Connection Engine
Create a connection engine to connect to your MySQL database:
engine = create_engine('mysql+pymysql://username:password@localhost/dbname')
Here, replace:
username
: Your MySQL username. password
: Your MySQL password. localhost
: The hostname or IP address of your MySQL server. dbname
: The name of the database you want to connect to.
Step 4: Create a Session
Create a session using the connection engine:
Session = sessionmaker(bind=engine)
session = Session()
This creates a new session that is bound to the connection engine. You can use this session to execute queries and interact with your database.
Step 5: Execute Queries
You can now execute queries using the session
object:
# Query all rows from a table
results = session.query(MyTable).all()
Insert a new row into a table
new_row = MyTable(name='John', age=30)
session.add(new_row)
session.commit()
Replace MyTable
with your actual table name and name
and age
with the columns you want to insert data into.
Step 6: Close the Session
When you're done, close the session:
session.close()
Here's a complete example script that demonstrates how to connect MySQL to SQLAlchemy Python:
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
engine = create_engine('mysql+pymysql://username:password@localhost/dbname')
Base = declarative_base()
class MyTable(Base):
tablename = 'my_table'
id = Column(Integer, primary_key=True)
name = Column(String)
age = Column(Integer)
Session = sessionmaker(bind=engine)
session = Session()
Query all rows from a table
results = session.query(MyTable).all()
for row in results:
print(row.name, row.age)
Insert a new row into a table
new_row = MyTable(name='John', age=30)
session.add(new_row)
session.commit()
session.close()
Replace the placeholders with your actual database credentials and table name. This script demonstrates how to execute queries and insert data using SQLAlchemy.