python for loop range(start at 1)
python for loop range(start at 1)
I'd be happy to help!
In Python, the range()
function is used to create an iterable sequence of numbers. By default, the range starts from 0. However, you can specify the starting value by passing the first argument to the range()
function.
Here's an example:
for i in range(1, 6):
print(i)
In this case, the output would be:
1
2
3
4
5
As you can see, the loop starts from 1 and goes up to 5.
Now, let's explore some interesting facts about range()
:
Starting value: By default, the range starts from 0. However, you can specify a different starting value by passing the first argument to the range()
function.
for i in range(5): # equivalent to range(0, 5)
print(i)
output:
0
1
2
3
4
Ending value: You can also specify an ending value by passing the second argument to the range()
function.
for i in range(1, 6):
print(i)
output:
1
2
3
4
5
Step size: By default, the step size is 1. However, you can specify a different step size by passing the third argument to the range()
function.
for i in range(0, 10, 2): # iterate over even numbers from 0 to 8
print(i)
output:
0
2
4
6
8
Negative values: You can also specify a negative starting value and/or ending value. The behavior is similar to the positive case.
for i in range(-5, 0): # iterate over numbers from -5 to 0
print(i)
output:
-5
-4
-3
-2
-1
0
Combining with other data structures: You can combine the range()
function with other data structures, such as lists or dictionaries.
numbers = list(range(1, 6))
print(numbers) # output: [1, 2, 3, 4, 5]
fruits = ['apple', 'banana', 'cherry']
for i in range(len(fruits)):
print(fruits[i])
output:
apple
banana
cherry
These are just a few examples of what you can do with the range()
function. Python's built-in functions and data structures make it easy to write concise and efficient code.
for loop in python with range list
I'll respond in English since you specifically requested it!
For loops are a fundamental construct in Python programming, allowing you to execute a block of code repeatedly for a specified number of iterations. In this response, we'll explore the for
loop in Python using the range()
function, which is particularly useful when working with lists or arrays.
Understanding the range()
Function
The range()
function generates an iterator that produces consecutive integers from a starting value to a stopping value (inclusive). The general syntax for using range()
is as follows:
for variable in range(start, stop):
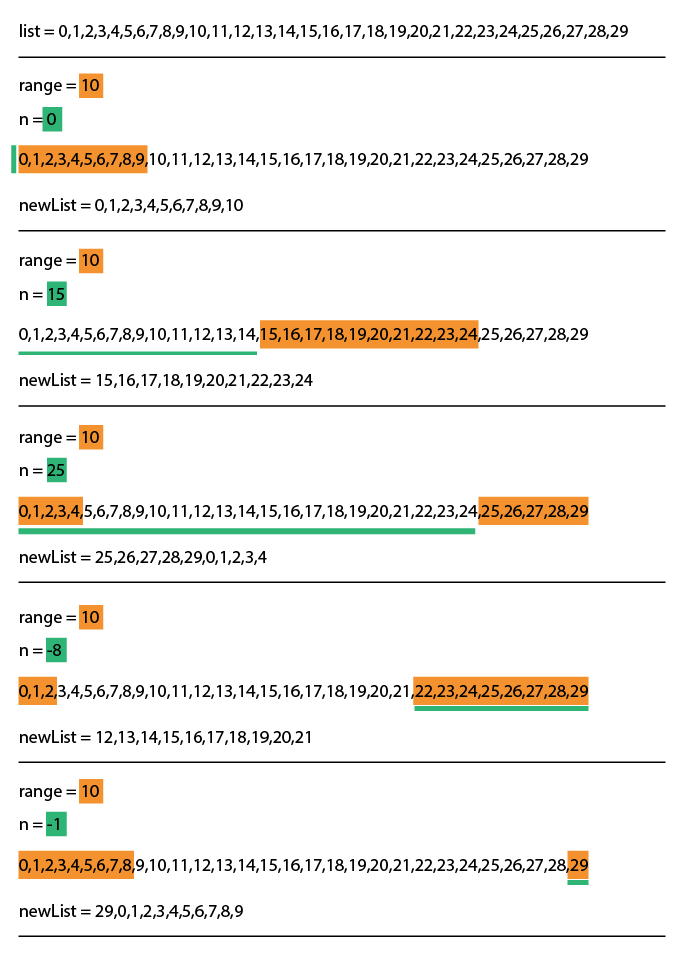
code to be executed
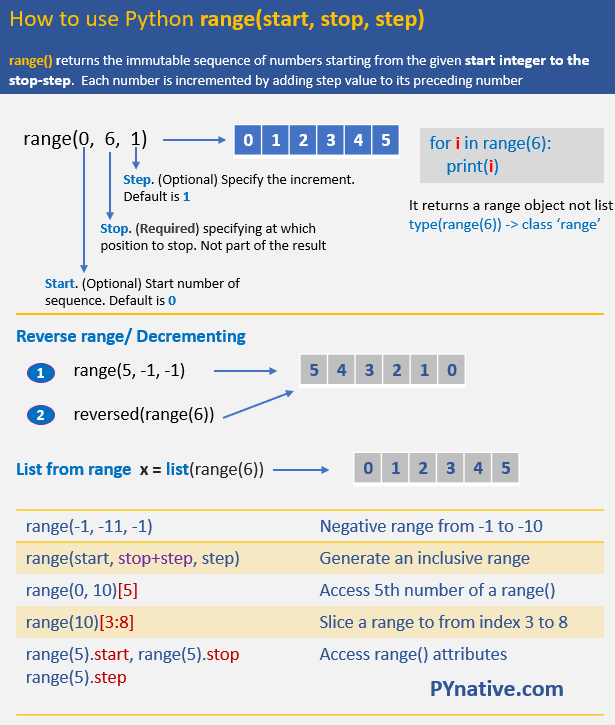
Here, start
is the initial value of the iteration, and stop
is the terminal value. If you omit the start
value, it defaults to 0.
Example 1: Simple Counting
Let's start with a simple example that demonstrates how to use the range()
function in a for
loop:
for i in range(5): # from 0 to 4 (inclusive)
print(i)
This will output the numbers 0, 1, 2, 3, and 4.
Example 2: Looping Through a List
Suppose you have a list of colors and want to iterate over it using a for
loop:
colors = ['red', 'green', 'blue', 'yellow']
for color in range(len(colors)): # iterate through the indices of the list
print(f"The {color}th color is: {colors[color]}")
This will output the following:
The 0th color is: red
The 1st color is: green
The 2nd color is: blue
The 3rd color is: yellow
Example 3: Looping Through a Specific Range
Now, let's assume you want to iterate over the numbers from 10 to 15 (inclusive):
for num in range(10, 16): # from 10 to 15 (inclusive)
print(num)
This will output:
10
11
12
13
14
15
Example 4: Looping Through a Negative Range
You can also use range()
with negative values. For instance, let's iterate over the numbers from -5 to 0 (inclusive):
for num in range(-5, 1): # from -5 to 0 (inclusive)
print(num)
This will output:
-5
-4
-3
-2
-1
0
Conclusion
In this response, we explored the range()
function and its application in Python for
loops. We saw examples of using range()
to iterate over a simple counting sequence, loop through a list, and iterate over a specific range (positive or negative).
Mastering the range()
function will enable you to write efficient and readable code that takes advantage of Python's built-in iteration capabilities.
Now, go forth and iterate with confidence!