Python if statement with or
Python if statement with or
I'd be happy to help!
The if
statement is a fundamental control structure in Python that allows you to execute a block of code when a certain condition is met. The basic syntax is as follows:
if condition:
code to be executed if the condition is True
However, what if you want to check multiple conditions? That's where the or
operator comes in!
The or
operator is used to combine two or more conditions. The statement will evaluate as True
if any of the conditions are True
. Here's an example:
x = 5
y = 3
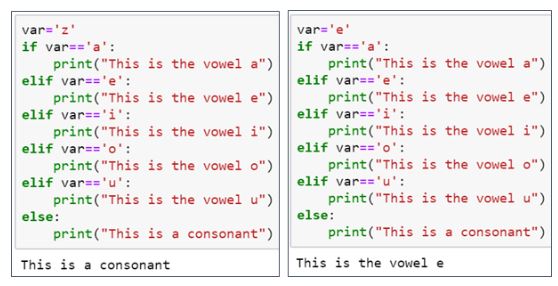
if x > 2 or y > 3:
print("At least one condition is True")
In this example, both conditions are evaluated separately. If either x
is greater than 2 or y
is greater than 3 (or both), the statement will evaluate as True
. The output would be:
At least one condition is True
Notice that if you only want to check if either of two conditions are True
, you can use a single if
statement with an or
operator. However, if you need to perform different actions based on the specific condition that evaluated as True
, you might want to consider using separate if
statements:
x = 5
y = 3
if x > 2:
print("X is greater than 2")
elif y > 3:
print("Y is greater than 3")
This code will output:
X is greater than 2
Another example where or
can be useful is when you're checking if a user meets certain requirements. For instance, to check if a user is at least 18 years old or has a certain level of experience, you could write:
age = 22
experience = "Intermediate"
if age >= 18 or experience == "Advanced":
print("User can access advanced features")
In this example, the or
operator allows you to check for multiple conditions simultaneously. If the user is at least 18 years old (age >= 18
) or has an experience level of "Advanced", they can access advanced features.
In conclusion, using if
statements with or
in Python provides a powerful way to evaluate complex conditions and perform actions based on those conditions. Whether you're checking for multiple conditions simultaneously or performing different actions based on specific conditions, understanding the basics of if
statements and the or
operator can help you write more efficient and effective code!
Python if multiple conditions or
I'd be happy to respond in English and explain how to use the OR operator in Python!
In Python, the OR operator is represented by a vertical bar (|
) and is used to combine two or more conditional statements. When evaluating an expression with multiple conditions separated by OR operators, the interpreter checks each condition from left to right until it finds one that evaluates to True
. If all conditions evaluate to False
, the entire expression still returns True
.
Let's look at some examples:
Simple OR:x = 5
y = 10
print(x > 3 or y > 7) # Output: True
In this example, we have two conditions: x > 3
and y > 7
. Since both are true, the expression evaluates to True
.
x = 5
y = 10
z = 15
print(x > 3 or y > 7 or z > 12) # Output: True
Here, we have three conditions: x > 3
, y > 7
, and z > 12
. The interpreter checks each condition until it finds one that evaluates to True
. Since z > 12
is true, the expression returns True
.
x = 5
y = 10
print(not x < 0 or y < 2) # Output: True
In this example, we use the NOT operator (not
) to negate the conditions. The interpreter will first evaluate not x < 0
, which is true because x >= 0
. Then it will check y < 2
, but since y >= 2
, the expression still returns True
.
When working with OR operators, remember that:
If all conditions areFalse
, the entire expression returns False
. You can chain multiple OR operators to create more complex expressions. Always prioritize readability and maintainability by using clear and concise variable names.
By mastering the OR operator in Python, you'll be able to create more robust and flexible conditional statements. Happy coding!