Why is Python an object?
Why is Python an object?
I'd be happy to explain why Python is considered an object!
In programming, an object is a self-contained entity that represents a real-world concept or entity. Objects have their own properties and behaviors, which are encapsulated within the object's boundaries.
Python, as a programming language, is often referred to as an "object" because it embodies many of the characteristics of an object in computer science.
Firstly, Python is self-contained: just like any other programming language, Python has its own syntax, semantics, and features that define how it works. It's not dependent on external factors or other languages; it's a complete entity on its own.
Secondly, Python has its own set of properties (or attributes) that describe what it is and what it does. For instance:
Its syntax: Python's syntax is defined by its indentation rules, variable naming conventions, etc. Its data types: Python supports various data types like integers, strings, lists, dictionaries, etc. Its libraries: Python has a vast array of built-in libraries that provide functionality for tasks like file I/O, networking, graphics, and more.These properties make Python an object in the sense that it has its own identity, characteristics, and behavior.
Lastly, Python's behavior is encapsulated within its boundaries. You can use Python to perform various tasks like data analysis, machine learning, web development, scripting, etc., but those tasks don't affect what Python is fundamentally; it remains a programming language with its own set of features.
In conclusion, Python can be considered an object because:
It's self-contained and doesn't depend on external factors. It has its own properties (or attributes) that define what it does and how it works. Its behavior is encapsulated within its boundaries and remains unchanged, regardless of the tasks performed with or through Python.Does this explanation help clarify why Python can be referred to as an object?
python object()
I'm happy to respond in English!
The object()
function in Python is a built-in constructor that returns an instance of the object
class, which is the base class of all objects in Python.
When you call object()
, it creates an instance of object
with no attributes or methods. You can think of it as a "blank slate" or a "null object".
Here are some key characteristics of the object
class:
object
class has no attributes, meaning you cannot access any variables or properties using dot notation (e.g., obj.attribute
). No methods: Similarly, the object
class has no methods, so you cannot call any functions on an instance of object
. No inheritance: The object
class is the base class of all other classes in Python, which means that every object in Python ultimately inherits from object
. However, instances of the object
class itself do not inherit from any other class. No special methods: Unlike many other Python objects, an instance of object
does not have special methods like __str__()
, __repr__()
, or __hash__()
.
Here's an example of using the object()
function:
obj = object()
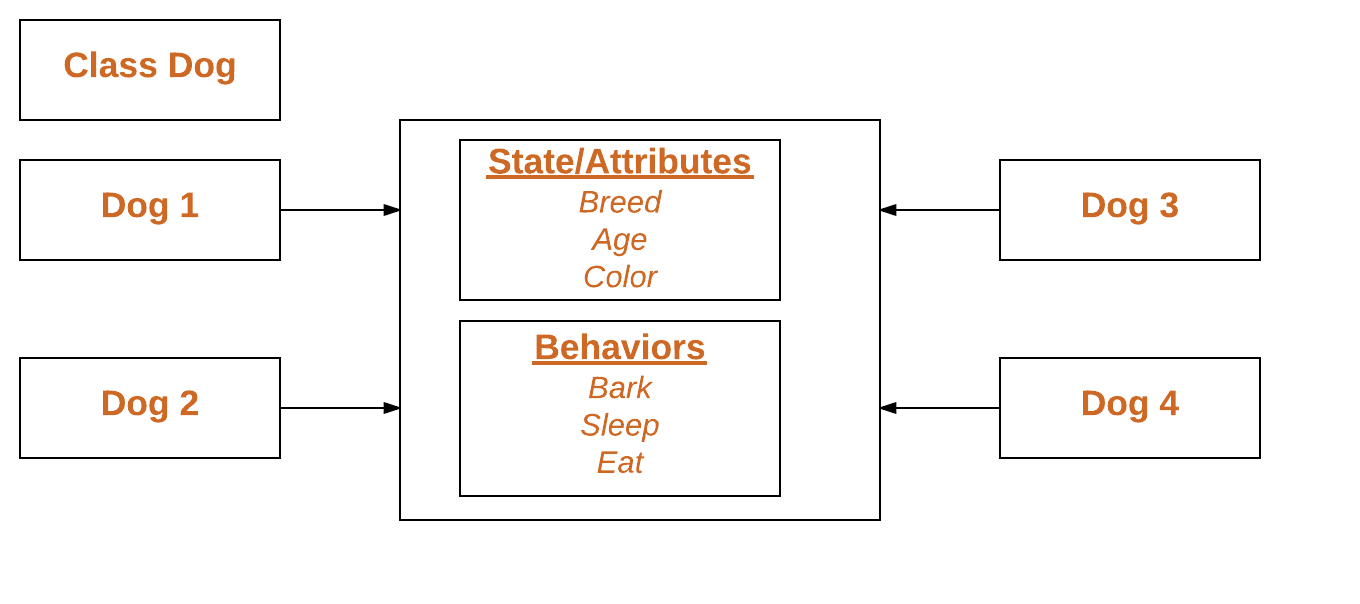
print(type(obj)) # Output: <class 'object'>
print(hasattr(obj, "attribute")) # Output: False (because obj has no attributes)
print(callable(obj)) # Output: False (because obj has no methods)
As you can see, an instance of object
is essentially a null object with no attributes or methods.
When might you use the object()
function? Well, there are a few scenarios where creating an instance of object
might be useful:
object
can serve as a placeholder until you have more information. Type hinting: When you're writing type hints for your functions or variables, using the object()
constructor can help indicate that a variable is supposed to hold an object with no specific attributes or methods. Testing and debugging: In tests and debug sessions, creating an instance of object
can be helpful when you need to simulate a null or "unknown" state for your code.
In summary, the object()
function in Python creates an instance of the base object
class, which is essentially a null object with no attributes or methods. While it might not seem like a very exciting topic, understanding how the object
class works can be useful when writing robust and reliable code!