How to check CPU usage using Python?
How to check CPU usage using Python?
To check CPU usage using Python, you can use the psutil
library which is a cross-platform library for retrieving information on running processes and system utilization (CPU, memory, disks, networking, users, etc.) in Python.
Here's an example of how to do it:
import psutil
Get CPU timeslice usage
cpu_timeslice_usage = psutil.cpu_timeslice()
print(f"Timeslice usage: {cpu_timeslice_usage}%")
Get CPU core count
cpu_core_count = psutil.cpu_count(logical=False)
print(f"CPU core count: {cpu_core_count}")
Get CPU percent usage
cpu_percent_usage = psutil.cpu_percent(interval=1, percpu=True)
print(f"CPU percentage usage: {cpu_percent_usage}%")
The psutil
library provides several ways to get CPU information:
cpu_timeslice()
: Returns the CPU timeslice usage (0-100). cpu_core_count(logical=False)
: Returns the number of CPU cores available. The logical=False
parameter means you're getting the physical core count, not the logical one (if there are hyper-threading or similar technologies involved). cpu_percent(interval=1, percpu=True)
: This method returns a tuple with four values: total CPU usage and usage for each of the available CPUs. You can specify the time interval (interval=1
in this case means 1 second).
Here's how you install psutil
library:
pip install psutil
Note that psutil
requires Python 3.5 or later, and is compatible with Windows, macOS and Linux.
Also note that on Unix-based systems (like Linux), you might need to run your script using sudo
if the system doesn't have a graphical interface:
sudo python your_script.py
I hope this helps!
Python get CPU usage of process
Here's a Python solution to get the CPU usage of a process:
import psutil
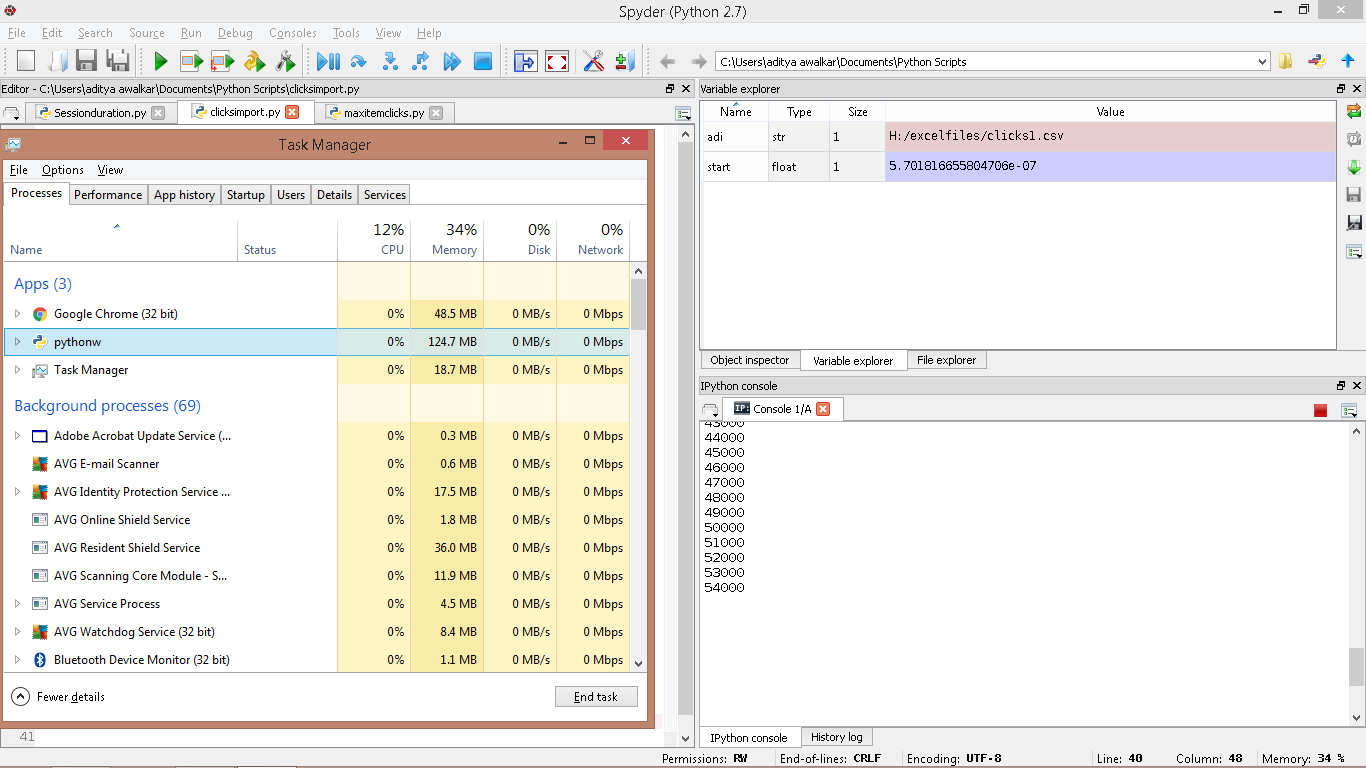
import time
Get the process ID you want to monitor
process_id = 1234
Create an instance of Process class
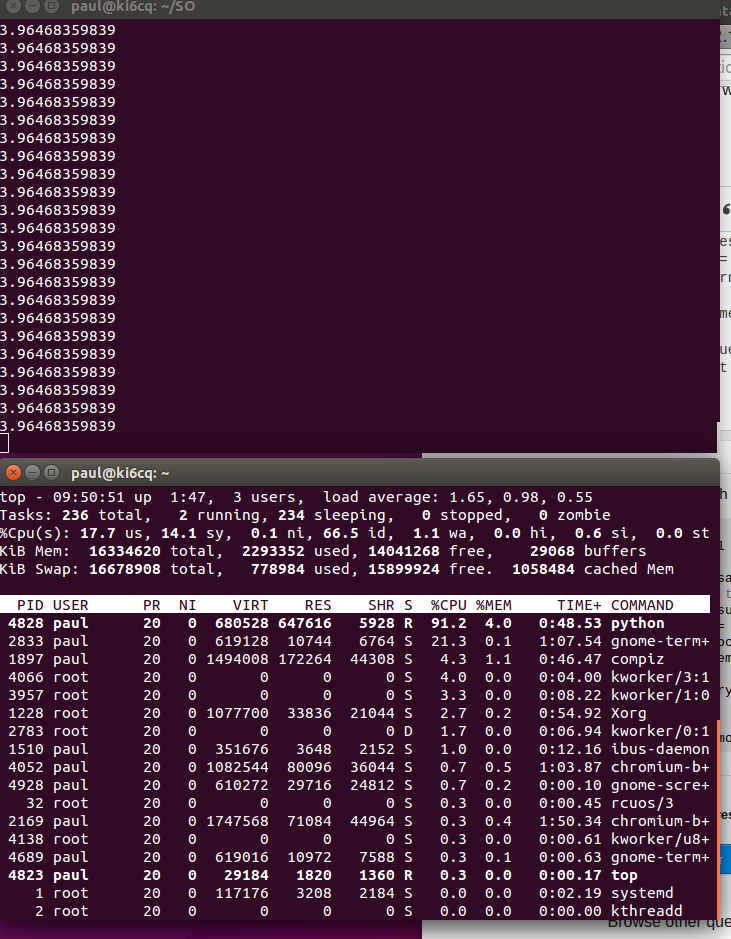
p = psutil.Process(process_id)
while True:
try:
Get the CPU usage percentage
cpu_usage = p.cpu_percent()
Print the CPU usage
print(f"CPU Usage: {cpu_usage}%")
Sleep for 1 second before getting next CPU usage
time.sleep(1)
except psutil.NoSuchProcess:
print("Process not found!")
break
except KeyboardInterrupt:
print("nKeyboardInterrupt caught! Exiting...")
break
Here's how this code works:
We first import thepsutil
library, which provides a way to access information about running processes and system utilization metrics (such as CPU usage). We then create an instance of the Process
class from psutil
, passing in the process ID we're interested in monitoring. In the while loop, we call the cpu_percent()
method on our process object. This method returns the CPU usage percentage of that process for the duration of its execution since the last time it was accessed by a call to cpu_percent()
. If you want the total CPU usage since the process started, use cpu_percent(interval=None)
with no interval argument. We print out the CPU usage percentage and then sleep for 1 second before getting next CPU usage. This can be adjusted based on how frequently you need the data. The code also includes exception handling to deal with scenarios where the specified process might not exist (e.g., if we mistakenly provide a non-existent ID) or when the user manually terminates the script while it's still running.
This code provides an effective way to monitor CPU usage of specific processes in real-time, and you can customize it according to your needs.