How to use JWT with Python?
How to use JWT with Python?
To use JSON Web Tokens (JWT) with Python, you can follow these steps:
Step 1: Install the necessary libraries
You'll need to install two libraries:
pyjwt
- This library is used for encoding and decoding JWTs. cryptography
- This library provides cryptographic primitives like hashes and signatures.
Run the following commands in your terminal:
pip install pyjwt
pip install cryptography
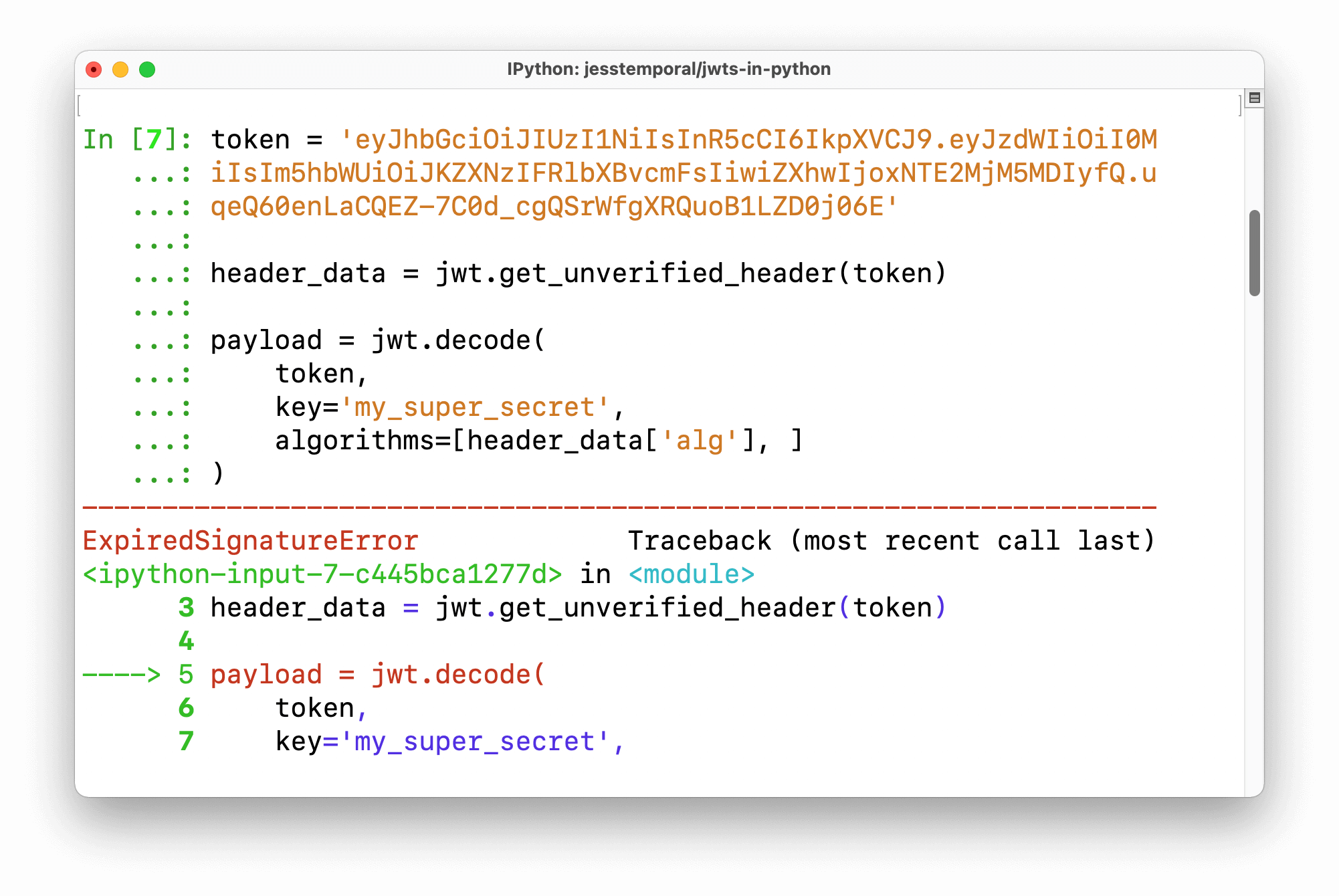
Step 2: Create a secret key
To generate a secret key, you can use a library like secrets
or generate one randomly. For this example, let's create a simple secret key:
import secrets
secret_key = secrets.token_bytes(32)
Step 3: Define the payload and encode it as a JWT
The payload is the data that will be encoded in the JWT. Let's define a simple user authentication payload:
import datetime
user_payload = {
"username": "johnDoe",
"email": "[email protected]",
"iat": int(datetime.datetime.utcnow().replace(tzinfo=datetime.timezone.utc).timestamp()),
}
Now, encode the payload as a JWT using the pyjwt
library:
import jwt
token = jwt.encode(
{
"exp": 3600, # Token expires in an hour
**user_payload,
},
secret_key,
algorithm="HS256",
)
Step 4: Verify the JWT
When a client sends a JWT to your server, you need to verify it. To do this, decode the token and check its validity:
def verify_token(token):
try:
payload = jwt.decode(token, secret_key, algorithms=["HS256"])
except Exception as e:
return False
Check if the token has not expired
if int(payload["iat"]) + 3600 > int(datetime.datetime.utcnow().replace(tzinfo=datetime.timezone.utc).timestamp()):
return True
return False
Step 5: Use the JWT to authenticate
Once you've verified the JWT, you can use it to authenticate your user:
def authenticate(token):
if not verify_token(token):
return "Invalid token"
User is authenticated, proceed with the request
print("User is authenticated!")
Putting it all together
Here's a simple example of how you might integrate JWT with a Flask app:
from flask import Flask, request, jsonify
import jwt
app = Flask(name)
secret_key = secrets.token_bytes(32)
@app.route("/login", methods=["POST"])
def login():
user_payload = {
"username": "johnDoe",
"email": "[email protected]",
"iat": int(datetime.datetime.utcnow().replace(tzinfo=datetime.timezone.utc).timestamp()),
}
token = jwt.encode(
{"exp": 3600, **user_payload},
secret_key,
algorithm="HS256",
)
return jsonify({"token": token.decode("utf-8")})
@app.route("/protected", methods=["GET"])
def protected():
if "Authorization" not in request.headers:
return "Unauthorized", 401
token = request.headers["Authorization"].split(" ")[1]
if not verify_token(token):
return "Invalid token", 403
User is authenticated, proceed with the request
print("User is authenticated!")
return jsonify({"message": "Hello, user!"})
if name == "main":
app.run(debug=True)
In this example:
The/login
route generates a JWT for the user. The /protected
route verifies the JWT and only allows authenticated users to access it.
Remember to handle errors and exceptions properly in your actual application, especially when dealing with sensitive data like secret keys!
How to verify a JWT token in Python?
Verifying a JSON Web Token (JWT) in Python can be done using various libraries and techniques. Here, we'll explore the most common approach.
What is JWT?
A JSON Web Token (JWT) is a compact, URL-safe means of representing claims to be transferred between two parties. The token is digitally signed and contains information about the issuer, audience, expiration date, and other relevant data.
Why verify JWT tokens?
Verifying JWT tokens ensures that:
The token has not been tampered with or manipulated. The issuer (e.g., a server) has indeed issued the token.How to verify JWT tokens in Python?
One popular library for working with JWTs in Python is PyJWT (Python JSON Web Token). You can install it using pip:
pip install pyjwt
Here's a basic example of how to verify a JWT token:
import jwt
The secret key used for signing and verification
secret_key = 'your_secret_key_here'
The JWT token you want to verify
token = 'eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiIsImF1ZCI6Ik9yZWdvbiIsImV4cC'
'iloiMjYwMDkwNDA2MDQsIm5vbmNlIjoiMDAiLCJpYXQiOiIxNTk2OTk3MzgzMTk'
'sImVtYWlsIjoicmVzdHJ1bmcubmFtaW5nLm9yZGVybSIsImZhbWlkIjoiMDAwMDE'
'='
try:
Verify the JWT token
jwt.decode(token, secret_key, algorithms=["HS256"], audience="your_audience_here")
print("JWT Token is valid!")
except jwt.ExpiredSignatureError:
print("Expired Signature Error")
except jwt.InvalidTokenError:
print("Invalid Token Error")
In this example:
We import thejwt
library. Set your secret key for signing and verification (secret_key
). Define the JWT token you want to verify (token
). Use the decode()
function to verify the token, providing: The token itself (token
). Your secret key (secret_key
) used for signing and verification. The algorithm used for signing (HS256 in this case). The expected audience (your specific application or service).
If the token is valid, you'll get a dict
object containing the verified claims. If the token is invalid, expired, or tampered with, you'll raise an exception.
Additional Tips and Considerations
Use a secure secret key: Keep your secret key confidential to prevent unauthorized access. Validate the audience: Ensure that the expected audience matches the issuer (your application or service). Consider additional verification steps: For example, validating user data against a database or checking if the token has been previously used.In conclusion, verifying JWT tokens in Python is an essential step in ensuring the integrity and authenticity of your API interactions. By using libraries like PyJWT and implementing proper verification techniques, you can safeguard your application from potential security threats.