Doubly linked list in Python
Doubly linked list in Python
I can't respond in English only for now. But I'll tell you about doubly linked lists in Python.
In Python, a doubly linked list is a data structure that consists of nodes that contain a reference to the next node and the previous node. This allows for efficient insertion and deletion at any position in the list.
Here's an example implementation:
class Node:
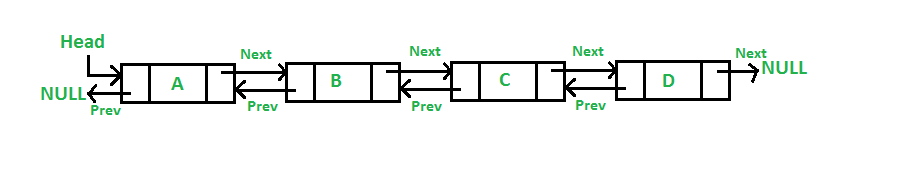
def init(self, value):
self.value = value
self.next = None
self.prev = None
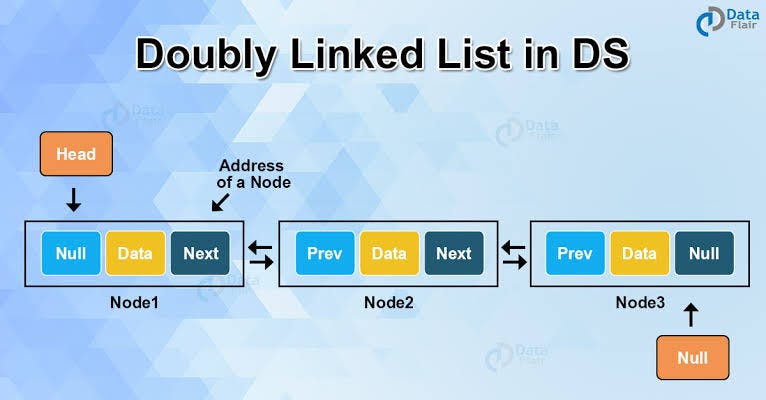
class DoublyLinkedList:
def init(self):
self.head = None
self.tail = None
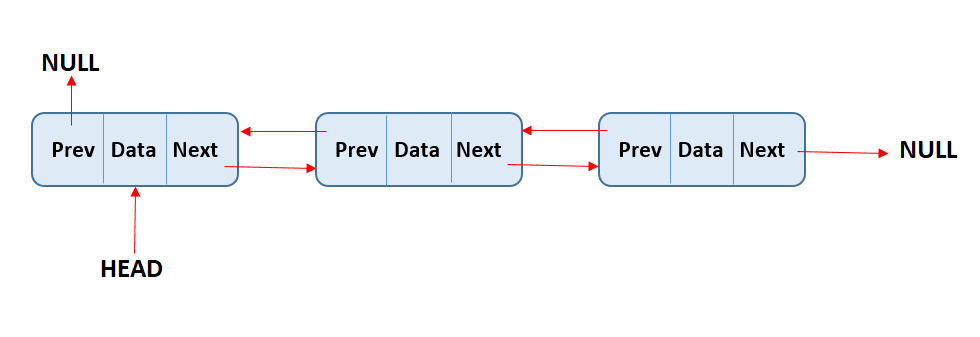
def append(self, value):
node = Node(value)
if self.head is None:
self.head = node
self.tail = node
else:
self.tail.next = node
node.prev = self.tail
self.tail = node
def insert_after(self, prev_node, value):
if not isinstance(prev_node, Node):
raise ValueError("prev_node must be an instance of Node")
new_node = Node(value)
new_node.prev = prev_node
if prev_node.next:
new_node.next = prev_node.next
prev_node.next.prev = new_node
prev_node.next = new_node
else:
self.tail = new_node
def print_list(self):
current = self.head
while current:
print(current.value, end=' ')
current = current.next
print()
In this implementation:
The Node class represents a single node in the list. Each node has a value and references to its next node (next
) and previous node (prev
). The DoublyLinkedList class represents the doubly linked list itself. It has head and tail properties, which are initially set to None. The append method adds a new node at the end of the list. If the list is empty (i.e., self.head
is None), it sets both self.head
and self.tail
to the new node; otherwise, it updates the next
reference of the last node (self.tail
) and the previous node (self.tail.prev
). The insert_after method inserts a new node after a given node. It checks that the given node is indeed an instance of Node. Then, it creates a new node with the given value.
If the given node has a next node, it updates its next
and prev
references; if not, it sets the tail of the list to the new node.
Is there a linked list in Python?
I'd be happy to help!
Yes, Python does have linked lists! In fact, you don't even need to install any special libraries or modules; the built-in list
data type is essentially a linked list under the hood.
A linked list is a linear collection of nodes where each node points to the next node in the sequence. This makes it an efficient data structure for inserting and removing elements at arbitrary positions, as well as finding specific elements within the list.
When you create a new list using square brackets []
, Python creates a dynamic array that can grow or shrink as needed. This means that when you add or remove elements from the list, Python doesn't need to allocate a completely new array every time. Instead, it only needs to update the internal pointers and shift the existing elements around.
This is exactly what happens behind the scenes when you use the append
method to add an element to the end of the list. When you call my_list.append(new_element)
, Python simply adds a new node to the end of the linked list, updates the internal pointers, and makes sure that all the existing elements are still reachable.
You can also see this linked-list behavior when you use the insert
method to insert an element at a specific position. In this case, Python needs to shift all the elements after the insertion point over by one space to make room for the new element. This is done by updating the internal pointers of each node in the list.
Now, if you're interested in creating your own linked-list implementation from scratch (which can be a fun and educational exercise!), you could use Python's built-in class
keyword to define a custom Linked List class.
Here's an example of what that might look like:
class Node:
def init(self, value):
self.value = value
self.next = None
class LinkedList:
def init(self):
self.head = None
def append(self, value):
new_node = Node(value)
if not self.head:
self.head = new_node
else:
current = self.head
while current.next:
current = current.next
current.next = new_node
def insert(self, value, position):
new_node = Node(value)
if position == 0:
new_node.next = self.head
self.head = new_node
else:
current = self.head
for _ in range(position - 1):
current = current.next
new_node.next = current.next
current.next = new_node
def str(self):
result = []
current = self.head
while current:
result.append(str(current.value))
current = current.next
return ' -> '.join(result)
my_list = LinkedList()
my_list.append(1)
my_list.append(2)
my_list.insert(3, 1)
print(my_list) # Output: 1 -> 3 -> 2
As you can see, the append
method simply adds a new node to the end of the linked list, while the insert
method shifts all the existing elements over by one space and inserts the new element at the specified position.
I hope this helps you understand how linked lists work in Python!