python security tools

I'd be happy to help! Here are some popular Python-based security tools:
Scaffolding: This is a framework for building web applications that includes features like authentication, authorization, and encryption. Paramiko: It's an SSHv2 client library for Python that allows you to make secure connections to remote servers. pyOpenSSL: OpenSSL is an encryption library that provides SSL/TLS support in Python. This module also includes the ability to create X509 certificates and sign them with private keys. PyCryptoDome: PyCryptodome is a self-contained Python package of low-level cryptographic primitives.
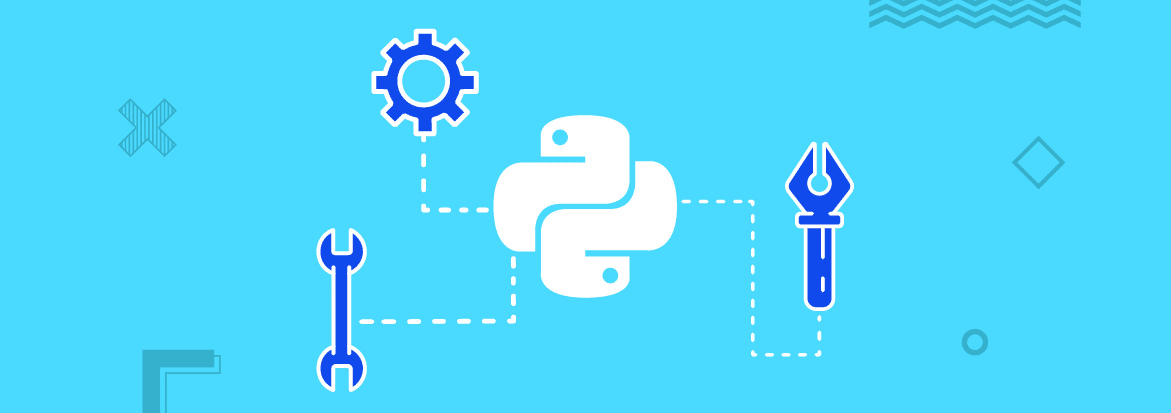
Requests-Auth: A simple library for authenticating HTTP requests with various authentication schemes such as Basic, Digest, Negotiate, Kerberos, and NTLM.
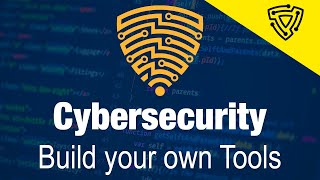
PycURL: An extension to the libcurl library that provides SSL/TLS support in Python.
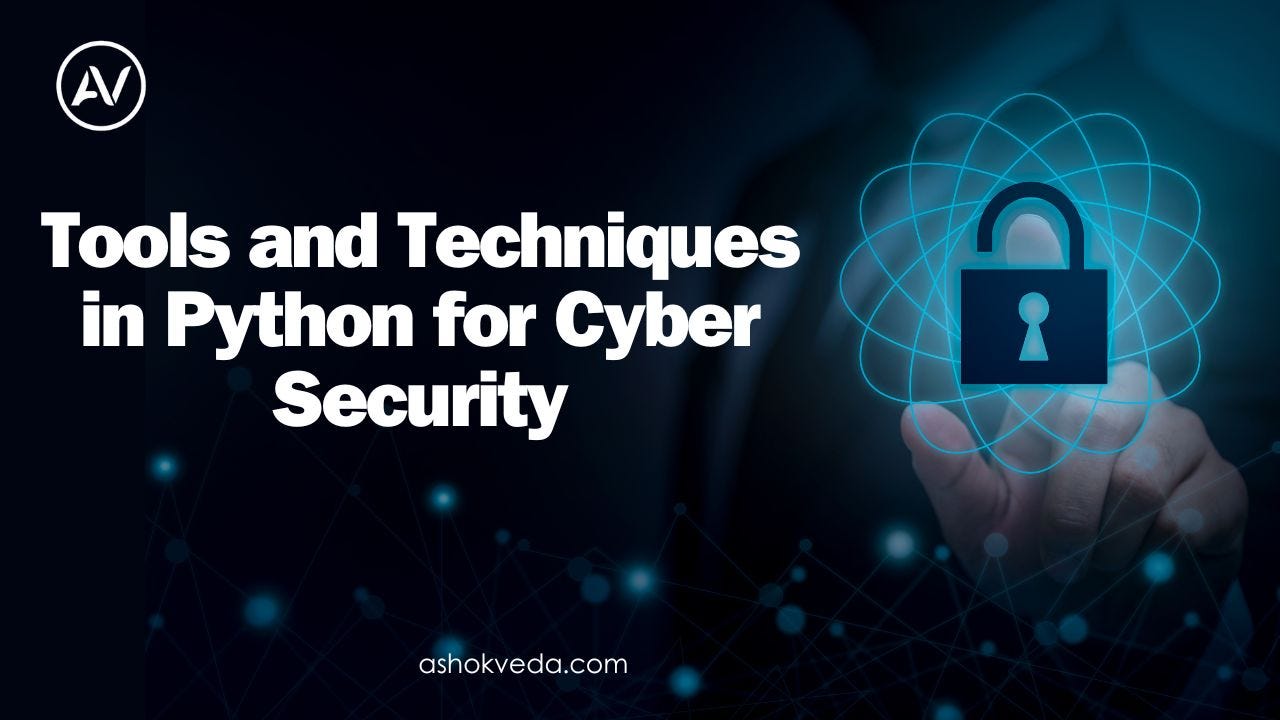
Fabric: Fabric is a powerful and flexible tool designed to help you automate repetitive and time-consuming tasks related to your work as a developer or deployment engineer. Scapy: Scapy is a powerful interactive packet manipulation program & library. It allows the user to send, sniff and dissect a wide variety of packets. Nmap Python Bindings: This is a set of Python modules that wrap up the functionality of the Nmap security scanner. SQLmap: SQLmap is an automated black box web application audit tool that aims at detecting and taking advantage of SQL injection vulnerabilities by generating and injecting payloads based on the identified database management system (DBMS), as well as providing a framework for implementing SQL injection attacks in a secure way. Burp Suite Python API: The Burp Suite is a powerful, flexible, and feature-rich web application penetration testing tool developed by PortSwigger. It can be used to identify vulnerabilities in web applications. OWASP Zed Attack Proxy (ZAP): This is an easy-to-use integrated development environment (IDE) for helping you identify vulnerabilities in your web application as well as other aspects of the network. Python-Cryptography: A module that provides cryptographic primitives and protocols, such as symmetric encryption, asymmetric encryption, message authentication codes (MACs), digital signatures, and more. Python-Hexdump: A tool for hexadecimal dump of any file or data, with options for decoding, displaying raw data, etc. Python-Netfilter: This is a Python extension that allows you to use the Linux netfilter framework for packet processing, filtering, and mangling.
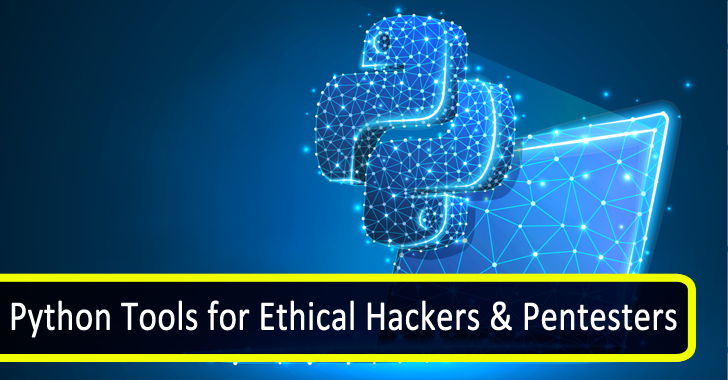
Python-SSLStrip: SSL stripping is a man-in-the-middle attack against SSL/TLS. It tries to downgrade the security of an existing SSL/TLS connection between two parties. Python-SSLPinning: This tool provides a secure way for your Python application to securely communicate over TLS with servers or other applications. Python-Cryptography Utilities: These are utility functions that help you to create and verify signatures, encrypt/decrypt data using symmetric/asymmetric encryption algorithms, etc. Python-Nmap: The Nmap scripting engine is a feature of the Nmap network security scanner that allows users to write custom scripts in the Python programming language to automate various tasks related to your work as a network administrator or penetration tester. Python-SSLScan: This tool provides an interface for performing SSL/TLS scans on remote hosts, including fingerprinting and scanning for SSL/TLS vulnerabilities. Python-Cryptography Keyring: This is an extension that allows you to securely store and retrieve cryptographic keys using a keychain or other secure storage methods. Python-SSL-Pinning-Tools: A set of tools for securely authenticating TLS connections with the help of Server Name Indication (SNI) support and pinning, as well as other techniques for identifying potential man-in-the-middle attacks. Python-Cryptography Utilities: These are utility functions that help you to create and verify signatures, encrypt/decrypt data using symmetric/asymmetric encryption algorithms, etc. Python-SSL-Scan-Utilities: This is a set of utilities that provide additional features for performing SSL/TLS scans on remote hosts, such as fingerprinting and scanning for SSL/TLS vulnerabilities. Python-Cryptography- Keyring: This is an extension that allows you to securely store and retrieve cryptographic keys using a keychain or other secure storage methods.How to make Python code secure?
To make a Python code secure, you need to follow best practices and use various security measures to prevent common attacks such as SQL injection, cross-site scripting (XSS), cross-site request forgery (CSRF), and command execution. Here are some tips:
Validate User Input: Always validate and sanitize user input before using it in your code. This can help prevent SQL injections, XSS attacks, and other types of attacks. Use Prepared Statements: When executing database queries, use prepared statements with parameters instead of concatenating strings directly into the query. This helps prevent SQL injection attacks. Use Secure Hashing Algorithms: Use secure hashing algorithms like bcrypt or PBKDF2 to store sensitive data like passwords securely. Use SSL/TLS: When transmitting data over a network, use SSL/TLS encryption to encrypt the communication and prevent eavesdropping. Keep Your Code Up-to-Date: Regularly update your dependencies and libraries to ensure you have the latest security patches. Don't Hardcode Sensitive Data: Never hardcode sensitive data like API keys, database passwords, or encryption keys directly into your code. Instead, use environment variables or secure configuration files. Use Secure Coding Practices: Follow best practices for coding, such as avoiding buffer overflows and using input validation to prevent attacks like buffer overflow attacks. Limit Privileges: Limit the privileges of users running your application, especially if it's a web application that allows users to upload files or execute code. Use Secure Libraries and Frameworks: Use secure libraries and frameworks that have been tested for security vulnerabilities and regularly updated with new patches. Conduct Regular Security Audits: Conduct regular security audits of your application to identify potential vulnerabilities before attackers do. Use a Web Application Firewall (WAF): If you're building a web application, use a WAF to detect and prevent common attacks like SQL injection, XSS, and CSRF. Store Sensitive Data Securely: Store sensitive data securely using encryption algorithms or secure storage solutions like encrypted files or databases. Use Authentication and Authorization: Implement robust authentication and authorization mechanisms to ensure only authorized users can access your application. Monitor Your Application's Security: Regularly monitor your application's security to detect potential vulnerabilities and respond quickly to reported incidents. Follow Secure Coding Guides: Follow secure coding guides like the OWASP Secure Coding Guide or the SANS Institute's Secure Coding Guidelines for best practices in secure coding.
Remember, writing secure code takes time and effort, but it's essential to protect your users' data and prevent attacks. By following these tips, you can significantly reduce the risk of security vulnerabilities in your Python code.