Python http requests w3schools json
Python http requests w3schools json
I'm happy to help you with that! Here's a detailed explanation on using Python's http.request()
function to send HTTP requests and working with JSON data from W3Schools:
What is JSON?
JSON (JavaScript Object Notation) is a lightweight, text-based data interchange format. It's often used to exchange data between web servers, web applications, and mobile apps. JSON data consists of name-value pairs, which are enclosed in curly braces {}. For example:
{
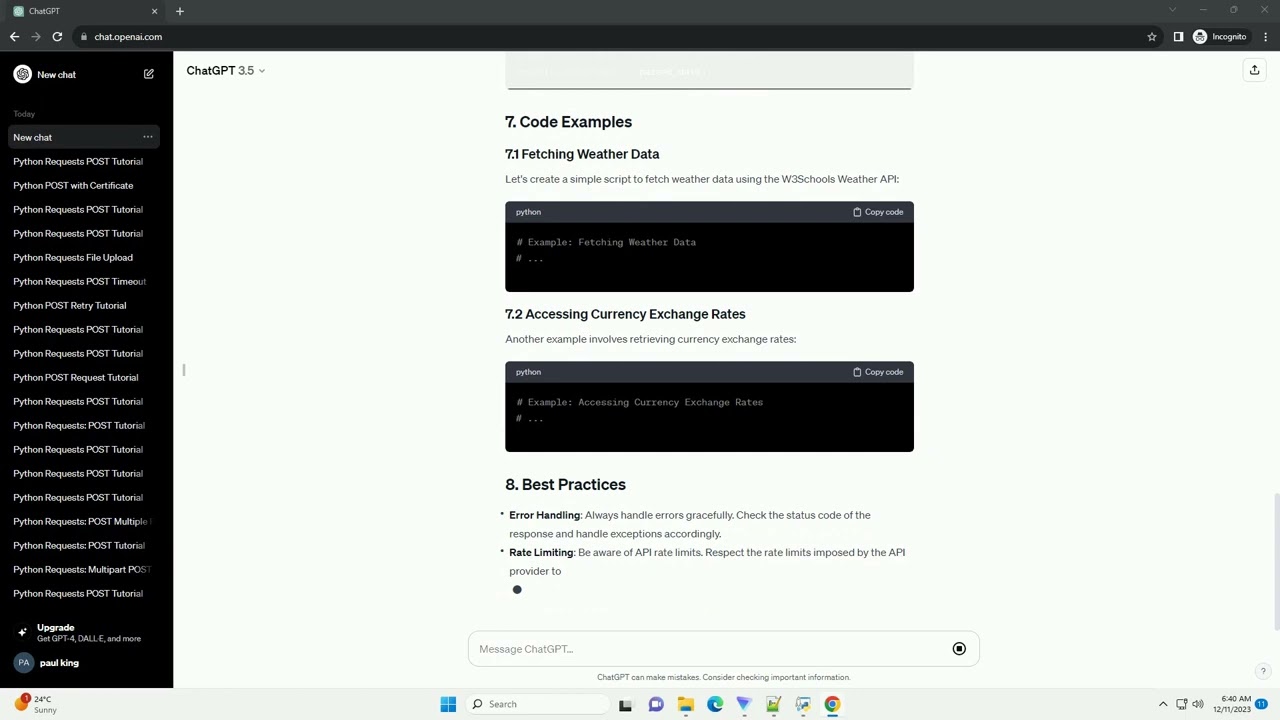
"name": "John",
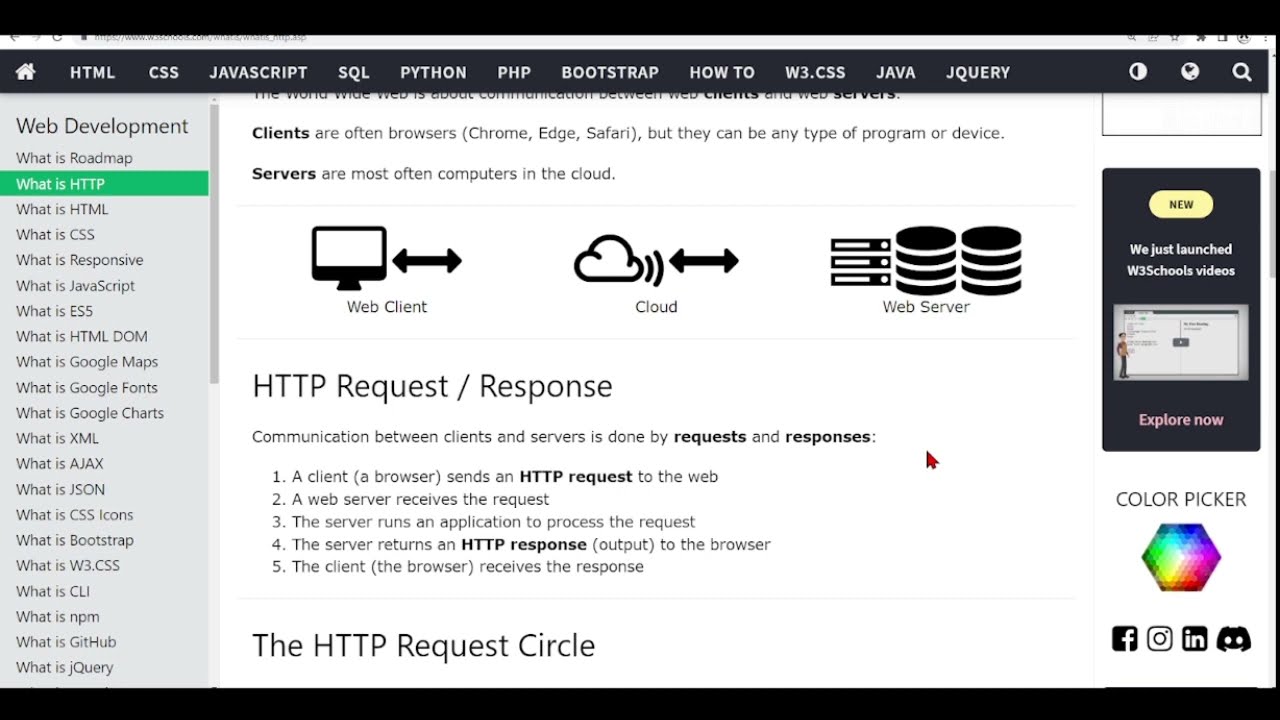
"age": 30,
"city": "New York"
}
Sending HTTP Requests with Python
The http
module in Python provides a way to send HTTP requests. One of its functions is request()
, which takes five arguments:
method
: The type of request (GET, POST, PUT, DELETE, etc.) url
: The URL of the request data
: The data to be sent with the request (optional) headers
: A dictionary containing headers for the request (optional) timeout
: The timeout value in seconds (optional)
Let's use this function to send a GET request to W3Schools' API, which returns JSON data about the top 10 programming languages:
import http
Set the URL and method
url = "https://www.w3schools.com/tags/json/top10_programming_languages.json"
method = "GET"
Set the headers (optional)
headers = {
"User-Agent": "My Python Script",
"Accept": "application/json"
}
Send the request
response = http.request(method, url, headers=headers)
Check if the response was successful
if response.status_code == 200:
Get the JSON data from the response
json_data = response.json()
print(json_data)
else:
print("Failed to retrieve data")
Working with JSON Data
Once we have the JSON data, we can work with it using Python's built-in json
module. Let's assume the JSON data is stored in a string called json_string
. We can parse this string into a Python dictionary using the following code:
import json
Parse the JSON string into a Python dictionary
data = json.loads(json_string)
print("Programming Languages:")
for language in data:
print(f"{language['name']} - {language['percentage']}")
Full Code Example
Here's the full code example that combines the previous snippets:
import http
import json
Set the URL and method
url = "https://www.w3schools.com/tags/json/top10_programming_languages.json"
method = "GET"
Set the headers (optional)
headers = {
"User-Agent": "My Python Script",
"Accept": "application/json"
}
Send the request
response = http.request(method, url, headers=headers)
Check if the response was successful
if response.status_code == 200:
Get the JSON data from the response
json_string = response.text
data = json.loads(json_string)
print("Programming Languages:")
for language in data:
print(f"{language['name']} - {language['percentage']}")
else:
print("Failed to retrieve data")
This code sends a GET request to W3Schools' API, retrieves the JSON data, and then parses it into a Python dictionary. Finally, it prints out the names and percentages of the top 10 programming languages.
I hope this helps you get started with sending HTTP requests and working with JSON data in Python!
How to send a request to a URL in Python?
I'd be happy to help!
To send a request to a URL in Python, you can use the requests
library. Here's a step-by-step guide on how to do it:
Step 1: Install the requests library
You'll need to install the requests
library first. You can do this by running the following command in your terminal:
pip install requests
Step 2: Import the requests library
Once installed, you can import the requests
library in your Python script:
import requests
Step 3: Define the URL and parameters (optional)
Next, define the URL you want to send a request to. You can also add any query parameters or headers if needed:
url = "https://example.com/api/data"
params = {"param1": "value1", "param2": "value2"}
headers = {"User-Agent": "Your User Agent"}
Step 4: Send the request
Use the requests
library to send a GET, POST, PUT, or DELETE request to the URL:
response = requests.get(url, params=params, headers=headers)
In this example, we're sending a GET request with query parameters and headers.
Step 5: Handle the response
The response
variable will contain the server's response. You can check if the request was successful by looking at the status code:
if response.status_code == 200:
Success! Print the response content
print(response.content.decode("utf-8"))
else:
Error! Print the error message
print(f"Error: {response.text}")
Common request types
The requests
library supports various request types, including:
GET
: Retrieves data from the server. (default) POST
: Sends data to the server. PUT
: Updates existing data on the server. DELETE
: Deletes data from the server.
Here's an example of sending a POST request:
response = requests.post(url, json={"key": "value"}, headers=headers)
Error handling
It's essential to handle errors when making requests. You can check if there were any errors by looking at the response.status_code
:
if response.status_code >= 400:
Error! Print the error message
print(f"Error: {response.text}")
That's it! With these steps, you should be able to send a request to a URL in Python using the requests
library. Remember to always handle errors and check the response status code to ensure your requests are successful.