How do you write to an existing text file in Python?
How do you write to an existing text file in Python?
I'm happy to respond in English for this question.
To write data to an existing text file in Python, you can use the open()
function and its corresponding mode. Here's a step-by-step guide:
Method 1: Using the 'w' mode
with open('existing_file.txt', 'w') as file:
file.write('New content to add!')
In this example, we're using the 'w'
mode, which stands for "write." If the file exists, its contents will be truncated (i.e., deleted). Then, we use the write()
method to add new content to the file.
Method 2: Using the 'a' mode
with open('existing_file.txt', 'a') as file:
file.write('nNew content to add!')
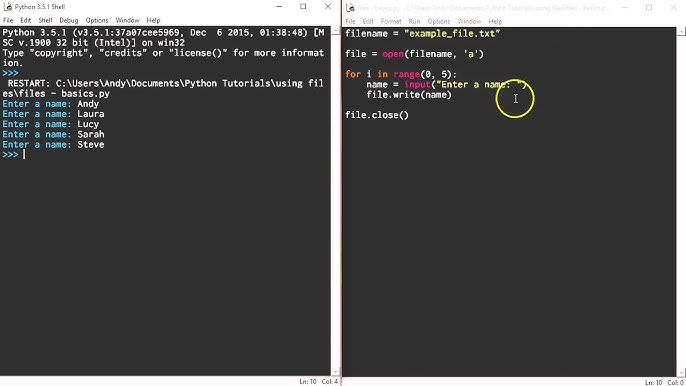
Here, we're using the 'a'
mode, which stands for "append." This mode allows you to append new content to the end of an existing file. The n
character is used to create a new line.
Method 3: Using the write()
function with a list
data = ['Line 1', 'Line 2', 'Line 3']
with open('existing_file.txt', 'w') as file:
file.write('n'.join(data))
In this example, we're using a list of strings and the write()
function to write the data to an existing text file. The 'w'
mode is used to truncate the file. The n
.join() method is used to concatenate the list elements with newline characters.
Tips and Considerations
Make sure to use the correct file path and name when opening the file. Be mindful of the file mode you choose ('w'
, 'a'
, or others). Incorrect modes can lead to data loss or corruption. If you're working with large files, consider using a buffer size to improve performance (e.g., file.write('content', 100)
). Always close the file when you're finished writing to avoid resource leaks.
By following these methods and tips, you should be able to successfully write data to an existing text file in Python.
How to write string to a text file in Python?
Writing strings to a text file is a fundamental task in programming, and Python provides several ways to do so. Here's how you can achieve this:
Method 1: Using the write()
Method
You can use the built-in open()
function to create a file object, and then use the write()
method to write strings to it:
with open('example.txt', 'w') as f:
f.write("This is the first line of text.n")
f.write("This is the second line of text.")
In this example, we create a file object called f
using the open()
function, specifying that we want to write ('w'
) to the file. We then use the write()
method to write strings to the file.
The n
character at the end of the first string is used to indicate a newline (also known as a line feed). When you open the file in a text editor, each line will start on a new line.
Method 2: Using String Formatting
Another way to write strings to a text file is by using Python's string formatting capabilities. You can use the %
operator or the format()
method to format your string, and then write it to the file:
with open('example.txt', 'w') as f:
f.write("%sn" % "This is the first line of text.")
f.write("{}n".format("This is the second line of text."))
In this example, we use the %
operator to format a string, and then write it to the file. We also use the format()
method, which is similar to the %
operator but provides more flexibility.
Method 3: Using a Context Manager
Python's context managers provide a way to ensure that files are properly closed after they are no longer needed. Here's how you can use a context manager to write strings to a text file:
import contextlib
@contextlib.contextmanager
def open_file(file_name):
f = open(file_name, 'w')
try:
yield f
finally:
f.close()
with open_file('example.txt') as f:
f.write("This is the first line of text.n")
f.write("This is the second line of text.")
In this example, we define a context manager called open_file()
using the contextlib.contextmanager
decorator. This context manager opens a file and yields its file object. We then use this context manager to open our file, and write strings to it.
Benefits
Writing strings to a text file in Python is an essential task that has many benefits:
Flexibility: You can write strings to files using various methods, which provides flexibility when working with different types of data. Efficiency: Writing strings to files can be more efficient than writing complex data structures, as it allows you to focus on the content of your file rather than the format. Readability: Text files are easy to read and understand, making them a great way to store and share data.Conclusion
In conclusion, there are several ways to write strings to a text file in Python, each with its own benefits. By using methods like write()
, string formatting, or context managers, you can efficiently and effectively write strings to files.