Python call class method within class
Python call class method within class
I'd be happy to explain how to call a class method within the same class in Python.
In object-oriented programming, methods are functions that belong to a class and are used to perform specific tasks or actions on instances of that class. A class method is similar to an instance method, but it is called using the class itself rather than an instance of the class. This can be useful when you need to perform some action that doesn't depend on any particular instance of the class.
To call a class method within the same class in Python, you don't actually "call" it in the classical sense. Instead, you use the @classmethod
decorator to define a method as a class method, and then you can access the method using the class name like a function.
Here's an example:
class MyClass:
@classmethod
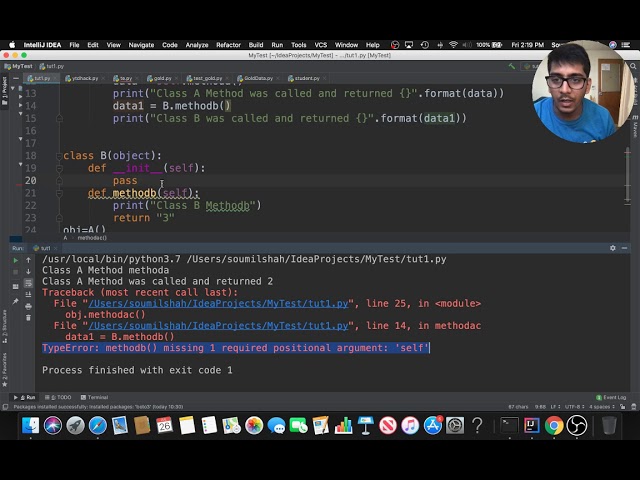
def my_class_method(cls):
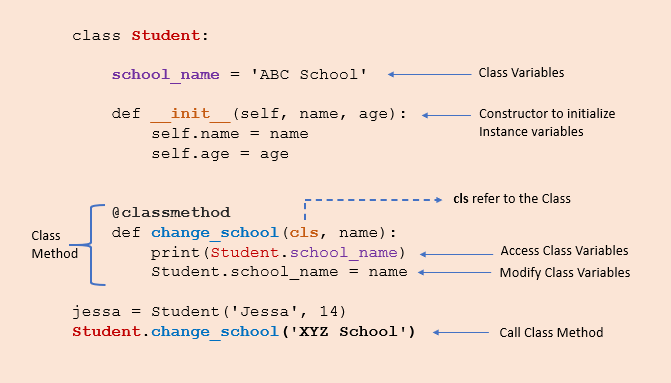
print("This is a class method!")
def init(self):
pass
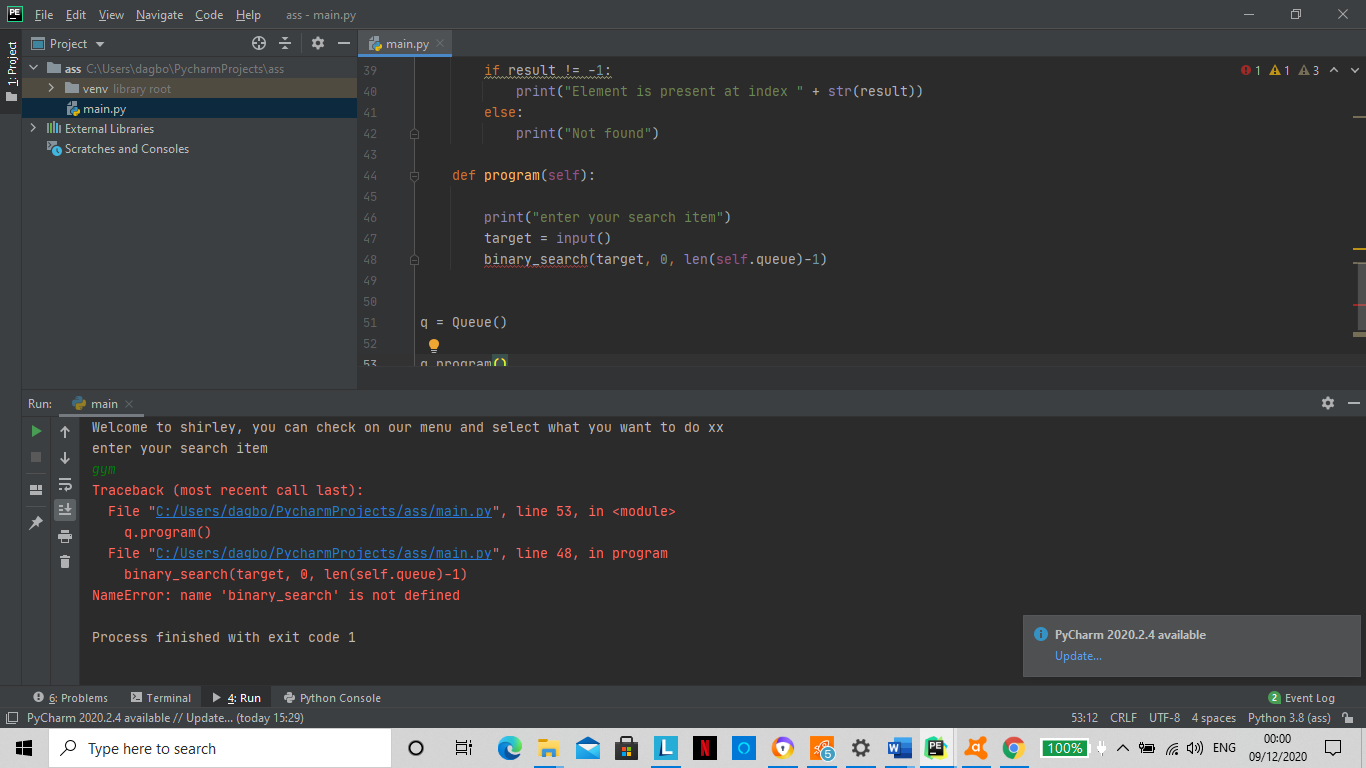
def instance_method(self):
self.my_class_method()
obj = MyClass()
obj.instance_method() # prints: This is a class method!
As you can see, the my_class_method
is defined using the @classmethod
decorator. To call this method, we don't need to create an instance of the class first; we can just access it directly from the class name:
MyClass.my_class_method() # prints: This is a class method!
This is because class methods are bound to the class itself rather than any particular instance.
Now, let's talk about why you might want to use class methods. One reason is that they provide a way to perform actions that don't depend on any particular instance of the class. For example, if you have a class BankAccount
and you want to create a method to calculate the total balance of all accounts in the bank, this would be a good candidate for a class method. You wouldn't need an instance of the BankAccount
class to call this method; you could just access it directly from the class name:
class BankAccount:
@classmethod
def total_balance(cls):
calculate the total balance here
return 1000
print(BankAccount.total_balance()) # prints: 1000
Another reason to use class methods is that they can provide a way to implement singleton-like behavior. For example, you might want to create a Logger
class with a static method get_logger()
that returns the only instance of the logger:
class Logger:
_logger = None
@classmethod
def get_logger(cls):
if not cls._logger:
cls._logger = Logger()
return cls._logger
logger1 = Logger.get_logger()
logger2 = Logger.get_logger() # returns the same instance as logger1
In summary, class methods are a useful tool in object-oriented programming that provide a way to perform actions that don't depend on any particular instance of the class. They can be used to implement singleton-like behavior and provide a way to access class-level functionality without creating an instance of the class.
How to call method from another class python
I'll respond in English as you requested.
In Python, calling a method from another class is achieved through object-oriented programming (OOP) concepts like inheritance, composition, or simply creating an instance of the other class and invoking the method.
Method 1: Inheritance
One way to call a method from another class is by inheriting from that class. This involves defining your own class that inherits attributes and methods from another class using the class
keyword followed by parentheses containing the parent class name.
class ParentClass:
def parent_method(self):
print("Parent method called!")
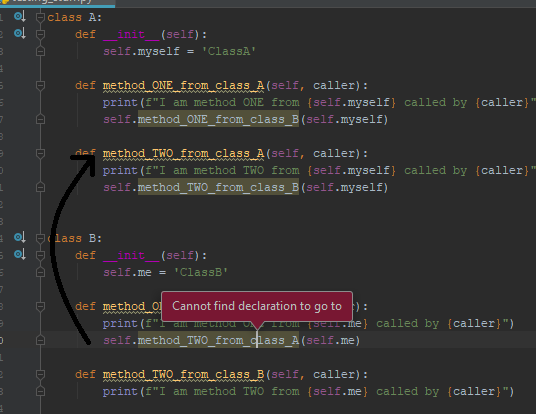
class ChildClass(ParentClass):
pass
child = ChildClass()
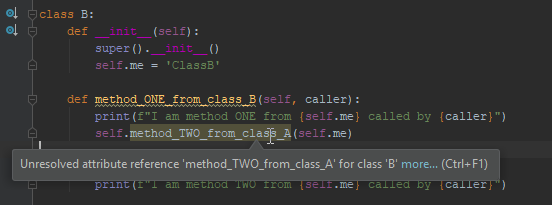
child.parent_method() # Output: Parent method called!
In this example, ChildClass
inherits from ParentClass
. You can then create an instance of ChildClass
and call the inherited method using dot notation (child.parent_method()
).
Method 2: Composition
Another approach is composition. You can create instances of both classes and pass the instance of one class to another as a parameter or attribute.
class ParentClass:
def parent_method(self):
print("Parent method called!")
class ChildClass:
def init(self, parent):
self.parent = parent
child = ChildClass(ParentClass())
child.parent.parent_method() # Output: Parent method called!
In this example, ChildClass
takes a ParentClass
instance as an attribute during construction. You can then call the parent_method()
from the ChildClass
instance using dot notation (child.parent.parent_method()
).
Method 3: Instantiation and Method Call
You can also create instances of both classes and directly call the method on one class, passing arguments if necessary.
class ParentClass:
def parent_method(self, arg):
print(f"Parent method called with {arg}!")
parent = ParentClass()
child = ChildClass()
parent.parent_method("hello") # Output: Parent method called with hello!
In this example, you create instances of both classes and call the parent_method()
directly on the ParentClass
instance, passing a string argument ("hello").
Additional Tips
Make sure to import the necessary modules or define the classes in the same file if you're using them from different files. Verify that the method is publicly accessible (i.e., not private) and can be called by other classes. You can use access modifiers likepublic
, private
, or protected
to control accessibility. Consider using design patterns, such as the Singleton pattern, to manage class instances and ensure a single instance of a class exists in your program.
In summary, calling a method from another class in Python involves techniques like inheritance, composition, or direct instantiation. By mastering these approaches, you can create robust and scalable software systems that leverage object-oriented programming principles.