What is invoke in Python?
What is invoke in Python?
I'll respond in English this time!
In Python, the invoke
function is a built-in module that allows you to execute external commands or scripts from within your Python program. This can be useful when you want to perform some system-specific tasks or interact with other software applications.
The invoke
function is part of the subprocess
module, which provides a way to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. The invoke
function takes two main arguments: the command or script you want to execute, and a dictionary of keyword-value pairs that can be used to customize the execution environment.
Here's an example of how you might use the invoke
function:
import subprocess
Execute the 'ls' command
result = subprocess.invoke(['ls', '-l'])
If the command was successful, print the output
if result.returncode == 0:
print(result.stdout.decode('utf-8'))
else:
print(f"Error: {result.stderr.decode('utf-8')}")
In this example, we use subprocess.invoke
to execute the 'ls' command with the '-l' option. We then check the return code of the executed command using result.returncode
. If the command was successful (i.e., it returned 0), we print the output to the console. Otherwise, we print an error message along with any standard error output from the command.
You can also use the check
method instead of invoke
, which will raise a subprocess.CalledProcessError
if the command returns a non-zero exit status:
import subprocess
try:
result = subprocess.check_output(['ls', '-l'])
print(result.decode('utf-8'))
except subprocess.CalledProcessError as e:
print(f"Error: {e.output.decode('utf-8')}")
The subprocess
module provides a wide range of features for working with external commands and scripts, including the ability to:
These features make it possible to write Python programs that can interact with other software applications, perform system-specific tasks, and automate routine administrative duties.
Python fabric vs invoke examples
Python Fabric and Invoke are both popular tools for automating tasks and deployment processes on remote servers using Python. Both tools provide a simple, easy-to-use API for executing shell commands, uploading files, and performing other system administration tasks.
Fabric
Fabric is a powerful and flexible tool for streamlining the process of deploying applications to multiple environments. It allows developers to define reusable code that can be run on remote machines, which simplifies the deployment process and reduces errors.
Here's an example of using Fabric to deploy an application:
from fabric import task
import os
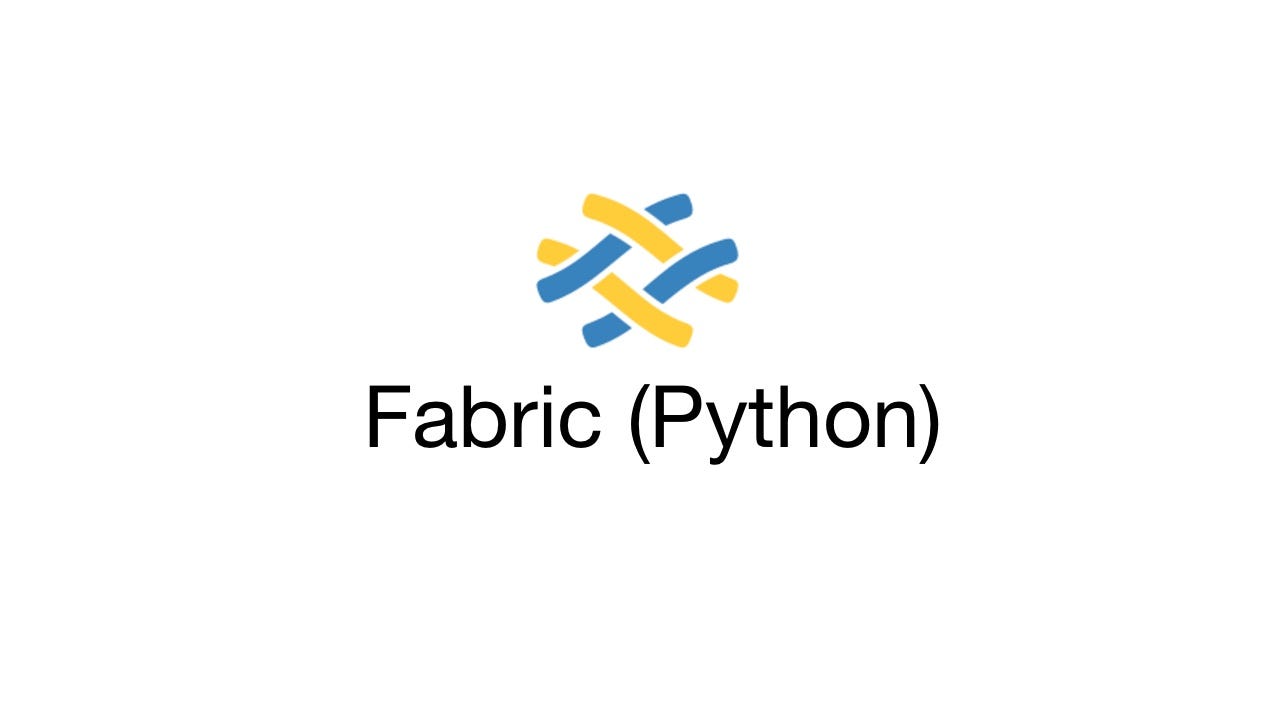
@task
def deploy(app_name):
Define the servers to connect to
servers = ['server1', 'server2']
for server in servers:
Change into the directory for the app
with cd(os.path.join('path/to/apps', app_name)):
Run a command on the remote machine
run('git pull origin master')
In this example, the deploy
function takes an app name as input and then iterates over a list of servers. For each server, it changes into the directory for the app, pulls down the latest code from the repository, and runs other commands to set up the environment.
Invoke
Invoke is another popular tool for automating tasks on remote servers using Python. It provides an easy-to-use API for executing shell commands, uploading files, and performing other system administration tasks.
Here's an example of using Invoke to deploy an application:
from invoke import task
@task
def deploy(context):
Define the servers to connect to
servers = ['server1', 'server2']
for server in servers:
Run a command on the remote machine
context.run(f'ssh {server} "cd path/to/apps/{app_name} && git pull origin master"')
In this example, the deploy
function takes an app name as input and then iterates over a list of servers. For each server, it runs a command that pulls down the latest code from the repository.
Comparison
Fabric and Invoke are both powerful tools for automating tasks on remote servers using Python. Both tools provide an easy-to-use API for executing shell commands, uploading files, and performing other system administration tasks.
Here's a comparison of the two tools:
Fabric: More flexible and customizable than Invoke Provides more advanced features such as parallel execution and error handling Has a steeper learning curve due to its complexity Invoke: Easier to use and more straightforward than Fabric Provides basic features for executing shell commands and uploading files May not be suitable for complex or large-scale deploymentsIn conclusion, both Fabric and Invoke are excellent tools for automating tasks on remote servers using Python. The choice between the two will depend on the complexity of your deployment process and your level of experience with automation scripting.
Conclusion
In this article, we have seen how to use Fabric and Invoke to automate deployment processes on remote servers using Python. We have also compared and contrasted the two tools in terms of their features, ease of use, and complexity. The choice between Fabric and Invoke will depend on your specific needs and experience with automation scripting.