How to call a class in python example
How to call a class in python example
I'd be happy to help you with that!
In Python, calling a class is quite straightforward. A class is essentially a template for creating objects, which are instances of the class that have their own set of attributes and methods. Here's an example of how to call a class in Python:
Let's say we want to create a simple "Person" class with some basic attributes like name, age, and height. We can define this class as follows:
class Person:
def init(self, name, age, height):
self.name = name
self.age = age
self.height = height
def greet(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
Now, let's create an instance of this class and call the greet
method:
john = Person("John", 30, 175)
john.greet() # Output: Hello, my name is John and I am 30 years old.
As you can see, we created a new object called "john" by calling the Person
class with the required attributes (name, age, height). We then accessed the greet
method on this object using dot notation (john.greet()
).
This is the basic idea of how to call a class in Python. You can also create multiple objects from the same class, like so:
jane = Person("Jane", 25, 160)
jane.greet() # Output: Hello, my name is Jane and I am 25 years old.
Notice that each object has its own set of attributes and methods. We can access these using dot notation (jane.name
, john.age
).
One more thing to note is that classes in Python are essentially blueprints for creating objects. The class itself doesn't store any data; the instances do. This means you can create multiple objects from the same class, each with its own set of attributes and methods.
Here's a summary of how to call a class in Python:
Define a class using theclass
keyword. Create an instance of the class by calling it with required attributes. Access the methods and attributes on the object using dot notation. You can create multiple objects from the same class.
That's it! I hope this helps you understand how to call a class in Python.
How do we call a class in Python?
In Python, a class is defined using the class
keyword followed by the name of the class and parentheses, which contain any arguments or base classes that it may inherit from.
Here's an example of how you might define a simple class called Animal
:
class Animal:
def init(self, name):
self.name = name
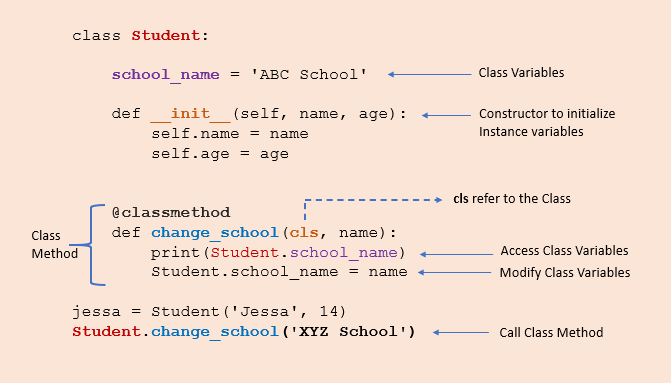
def sound(self):
print("The animal makes a sound!")
my_animal = Animal("Fido")
print(my_animal.name) # Output: Fido
my_animal.sound() # Output: The animal makes a sound!
In this example, the Animal
class has two methods: __init__
, which is called when an instance of the class is created, and sound
, which prints out a message.
The class
keyword is used to define the class itself. This keyword can only be used inside a function or module. The first line of any Python class definition must have the form class ClassName:
where ClassName
is the name given to the class.
When you create an instance of a class, you are creating a separate object that has its own state and behavior. In this example, we created an instance of the Animal
class called my_animal
. We then used dot notation (e.g., my_animal.name
) to access the attributes of the class.
Python supports several types of inheritance: single inheritance, multiple inheritance and method overriding. Single inheritance is when one class inherits from another. Multiple inheritance is when a class inherits from two or more classes. Method overriding occurs when a child class provides different implementation for an inherited method.
Here's an example of single inheritance:
class A:
def method(self):
print("A")
class B(A):
def method(self):
print("B")
In this case, B
inherits from A
. If you create an instance of B
and call its method
, it will print "B", even though the original method was in class A
.
Multiple inheritance allows a class to inherit from more than one parent class. Here's an example:
class A:
def method(self):
print("A")
class B:
def method(self):
print("B")
class C(A, B):
pass
In this case, C
inherits from both A
and B
.
Here's an example of method overriding:
class A:
def method(self):
print("A")
class B(A):
def method(self):
print("B")
In this case, when you call the method
on an instance of B
, it will print "B", even though the original method was in class A
. This is because the child class provided a different implementation for that method.
Python also supports abstract classes. Abstract classes are classes that can't be instantiated themselves and are used to provide a blueprint for other classes. Here's an example:
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def sound(self):
pass
class Dog(Animal):
def sound(self):
print("Woof!")
dog = Dog()
dog.sound() # Output: Woof!
In this case, Animal
is an abstract class because it has one or more abstract methods (methods that are declared but not defined). The Dog
class inherits from the Animal
class and provides a definition for its sound
method.
These are just some of the basics about classes in Python. There's much more to learn, such as decorators, static and instance methods, property, descriptor etc., but these should give you a good starting point.