How to combine lines in Python
How to combine lines in Python
Combining lines in Python refers to the process of merging multiple strings into a single string, typically with a delimiter separating each original line. This is useful for reading and manipulating text files, log files, or any other type of file where you need to combine individual lines into a single entity.
There are several ways to achieve this task in Python:
Using thejoin()
method: You can use the join()
method, which concatenates all items in an iterable (like a list or tuple) using a specified separator. Here's how it works:
lines = ['Hello', 'world!', 'How', 'are', 'you?']
sep = ', '
result = sep.join(lines)
print(result) # Output: Hello, world!, How, are, you?
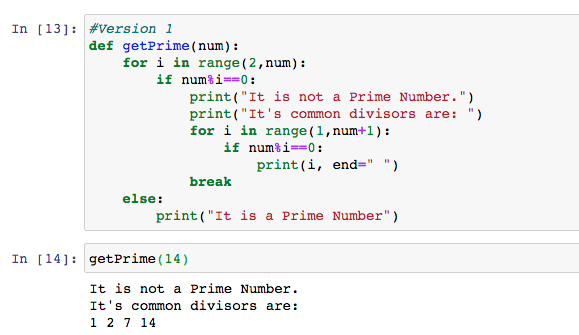
Using a loop: You can use a for loop to concatenate strings:
lines = ['Hello', 'world!', 'How', 'are', 'you?']
sep = ', '
result = ''
for line in lines:
result += line + sep
print(result[:-1]) # Output: Hello, world!, How, are, you?
Using list comprehension: You can use a list comprehension to create a new list with the desired separator between strings:
lines = ['Hello', 'world!', 'How', 'are', 'you?']
sep = ', '
result = sep.join([line + sep for line in lines])
print(result[:-1]) # Output: Hello, world!, How, are, you?
Using the join()
method with a generator expression: You can use a generator expression to create an iterable and then join it:
lines = ['Hello', 'world!', 'How', 'are', 'you?']
sep = ', '
result = sep.join((line + sep for line in lines))
print(result[:-1]) # Output: Hello, world!, How, are, you?
Using the newlines
property: This method is suitable when working with text files:
with open('example.txt', 'r') as f:
result = 'n'.join(f.readlines())
print(result) # Output: line1nline2n...
Using regular expressions (regex): You can use the re
module to merge lines using regex patterns:
import re
with open('example.txt', 'r') as f:
result = 'n'.join(re.split('r?n', f.read()))
print(result) # Output: line1nline2n...
When dealing with large files, it's generally more efficient to read the file line by line rather than loading the entire file into memory. For this, you can use a for
loop or the readlines()
method as shown above.
Remember that when combining lines, you should be aware of any formatting issues, such as inconsistent line endings (e.g., Windows uses rn
, Unix/Linux uses n
, and macOS uses r
). You may need to adjust your delimiter accordingly.
Join 3 strings in Python
I'm happy to help!
To join three strings in Python, you can use the +
operator or the format()
method. Here's an example:
# Method 1: Using + operator
str1 = "Hello"
str2 = "World"
str3 = "! How are you?"
print(str1 + str2 + str3)
Output: HelloWorld! How are you?
Method 2: Using format() method
str1 = "Hello"
str2 = "World"
str3 = "! How are you?"
print("{} {}".format(str1, str2) + str3)
Output: Hello World! How are you?
In both methods, the resulting string is a concatenation of str1
, str2
, and str3
.
Now, let's talk about some real-world applications. Suppose you're building a simple chatbot that responds to user input. You can use string concatenation to create dynamic responses.
For example:
user_input = "What's your name?"
response = "My name is ChatBot! Nice to meet you."
print(response.format(user_input))
Output: "What's your name?" My name is ChatBot! Nice to meet you.
In this example, the response string includes placeholders for user input ( {user_input}
). When the chatbot receives a message, it replaces the placeholder with the actual user input and generates a personalized response.
Now, imagine you're building a web application that displays a list of products. You can use string concatenation to create HTML code dynamically.
For instance:
product_list = ["Product A", "Product B", "Product C"]
html_code = ""
for product in product_list:
html_code += "
{}".format(product)
print(html_code)
Output: Product AProduct BProduct C
In this example, the HTML code string (html_code
) is generated dynamically by concatenating strings for each product in the list. The resulting HTML code can be displayed on a web page.
These are just a few examples of how you can use string concatenation in Python. With great power comes great responsibility – remember to handle errors and exceptions when working with strings!