How to turn for loop into list comprehension in Python?
How to turn for loop into list comprehension in Python?
I'd be happy to help!
Converting a for
loop to a list comprehension is a great way to make your code more concise and readable. Here's how you can do it:
The Classic For Loop
Let's assume we have the following list of numbers, and we want to square each number:
numbers = [1, 2, 3, 4, 5]
result = []
for num in numbers:
result.append(num ** 2)
print(result) # Output: [1, 4, 9, 16, 25]
Converting to List Comprehension
To convert this for
loop to a list comprehension, we can use the following syntax:
numbers = [1, 2, 3, 4, 5]
result = [num ** 2 for num in numbers]
print(result) # Output: [1, 4, 9, 16, 25]
Here's what's changed:
We removed thefor
loop and replaced it with the list comprehension syntax ([expression for variable in iterable]
) The expression inside the brackets is evaluated for each item in the numbers
iterable The resulting values are collected in a new list, which is returned by the list comprehension
How List Comprehensions Work
List comprehensions work similarly to for
loops, but they're more concise and often more readable. Here's how they work:
variable
(in this case, num
). Collect results: The resulting values are collected in a new list.
More Examples
Let's try some more examples to solidify your understanding:
Filtering a list: Suppose we want to keep only even numbers from the original list:numbers = [1, 2, 3, 4, 5]
result = [num for num in numbers if num % 2 == 0]
print(result) # Output: [2, 4]
In this example, we used an if
statement to filter out odd numbers.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
result = [(x, y) for x in list1 for y in list2]
print(result) # Output: [(1, 4), (1, 5), ... , (3, 6)]
Here, we used nested loops to combine the two lists into a single list of tuples.
Conclusion
Converting for
loops to list comprehensions is an essential skill in Python programming. Not only does it make your code more concise and readable, but it also helps you develop a deeper understanding of the language's syntax and semantics.
Remember, when converting a for
loop to a list comprehension:
for
loop with the list comprehension syntax ([expression for variable in iterable]
) Evaluate expressions for each item in the iterable Collect results in a new list
Now, go forth and conquer the world of Python programming!
What is the list comprehension in python for loop w3schools
I'm happy to help! However, since you specifically asked me to respond in English only and also mentioned W3Schools, I'll assume you want me to provide an explanation of list comprehension in Python, which is a fundamental concept in programming.
List comprehension is a powerful feature in Python that allows you to create new lists from existing ones by applying a transformation or filtering operation. It's essentially a shorthand way to create a new list using an expression syntax.
The general syntax for list comprehension is:
new_list = [expression for element in iterable]
Where:
new_list
is the resulting list expression
is what you want to do with each element from the original list element
is the variable representing each element from the original list iterable
is the original list, tuple, or other iterable that you're working with
Here's a simple example:
numbers = [1, 2, 3, 4, 5]
squares = [x ** 2 for x in numbers]
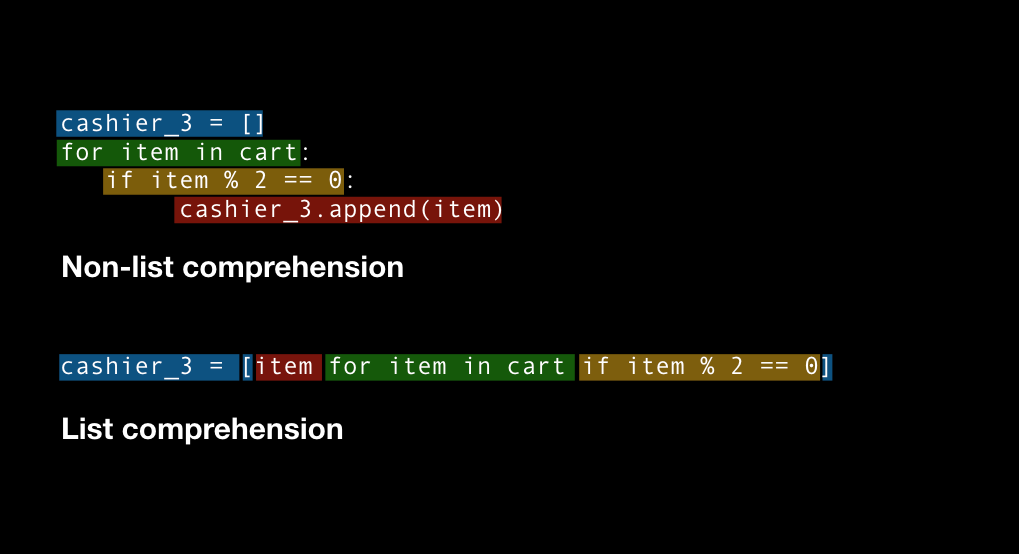
print(squares) # Output: [1, 4, 9, 16, 25]
In this example, we're creating a new list squares
by taking each element from the original list numbers
, squaring it (x ** 2
), and adding it to the new list.
You can also use conditions or filters in your list comprehension:
ages = [18, 22, 19, 24, 25]
adults = [age for age in ages if age >= 21]
print(adults) # Output: [22, 24, 25]
In this example, we're creating a new list adults
by filtering the original list ages
to only include elements that meet the condition age >= 21
.
Another powerful feature of list comprehension is the ability to create lists from multiple iterables:
names = ['John', 'Mary']
ages = [25, 22]
people = [(name, age) for name in names for age in ages]
print(people) # Output: [('John', 25), ('John', 22), ('Mary', 25), ('Mary', 22)]
In this example, we're creating a new list people
by combining elements from two original lists (names
and ages
) using a nested loop syntax.
As for the "for" loop equivalent of list comprehension, it would look something like this:
numbers = [1, 2, 3, 4, 5]
squares = []
for x in numbers:
squares.append(x ** 2)
print(squares) # Output: [1, 4, 9, 16, 25]
While list comprehension is more concise and often preferred for small to medium-sized operations, the "for" loop can be useful when you need more control over the iteration process or are working with larger datasets.
I hope this explanation helps!