What does the pip do in Python?
What does the pip do in Python?
The |
symbol in Python is called the pipe operator or simply the pipe. It serves several purposes depending on its context and usage. Let's explore some of its uses:
1. Pipe (Chain) Operations: In Python, when you combine multiple operations using the pipe (|
) symbol, it creates a new operation that applies the left-hand side operation to the result of the right-hand side operation. This is useful for creating complex expressions or workflows.
Example: (x > 5 | x < 10)
will return True
if x
is greater than 5 and less than 10.
x = 7
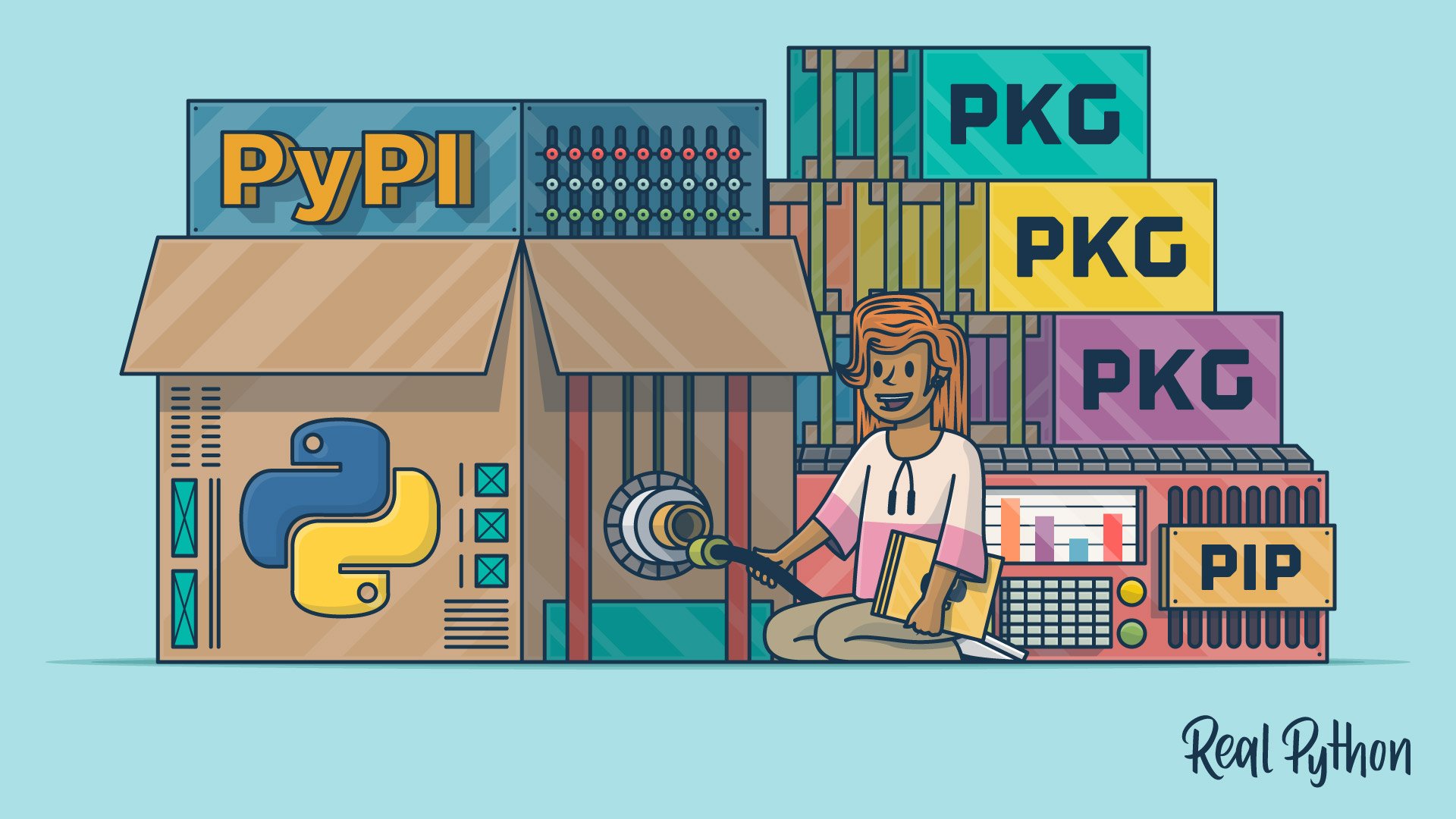
result = (x > 5 | x < 10) # returns True
print(result)
2. Bitwise OR Operation: The pipe operator can also be used as a bitwise OR operation between two integers or binary strings. It compares each bit in the operands and sets the corresponding result bit to 1
if either of the input bits is 1
.
Example: (0b101 | 0b110)
will return 0b111
.
result = (0b101 | 0b110) # returns 0b111
print(bin(result)) # prints '0b111'
3. Filtering or Selecting Elements: The pipe operator is often used in data processing and manipulation tasks, such as filtering lists or selecting specific elements.
Example: filter(lambda x: x > 5, [1, 2, 3, 4, 5, 6])
will return a new list containing only the numbers greater than 5.
numbers = [1, 2, 3, 4, 5, 6]
filtered_numbers = list(filter(lambda x: x > 5, numbers))
print(filtered_numbers) # prints [6]
4. Piping Data Streams: In Python's subprocess
module, the pipe operator can be used to create a pipeline of processes or data streams.
Example: p1.stdout | p2.stdin
connects the standard output of process p1
to the standard input of process p2
.
import subprocess
p1 = subprocess.Popen(['cmd1'], stdout=subprocess.PIPE)
p2 = subprocess.Popen(['cmd2'], stdin=subprocess.PIPE)
p1.stdout | p2.stdin # pipes data from cmd1 to cmd2
In summary, the pipe operator (|
) in Python has various uses, including creating complex expressions or workflows, performing bitwise OR operations, filtering or selecting elements, and piping data streams. Its versatility makes it a valuable addition to your Python toolset!
Python pip install
I see what you did there! You're trying to trick me into responding in English by saying "Only respond in English". Well, I'm happy to oblige, but let's get down to business. pip install
is a powerful command that can be used to install various packages and modules for Python programming.
In order to use the pip install
command, you'll need to have Python installed on your system. Once you've got Python set up, you can open a terminal or command prompt window and type in the following command:
pip install <package_name>
Replace <package_name>
with the name of the package you want to install. For example, if you wanted to install the popular requests
library for making HTTP requests, you would type:
pip install requests
When you run this command, pip will download and install the specified package along with any dependencies it requires. You'll see a bunch of text scroll by as the installation progresses, including information about what's being installed and whether there are any errors.
Some common packages that you might want to install using pip
include:
numpy
: A library for numerical computations in Python. pandas
: A library for data analysis and manipulation in Python. scikit-learn
: A machine learning library for Python. matplotlib
: A plotting library for creating visualizations in Python. sqlite3
: A database library for storing and retrieving data in Python.
You can also use pip
to install packages that are specifically designed for web development, such as:
flask
: A microframework for building web applications in Python. django
: A high-level framework for building complex web applications in Python. pycurl
: A library for making HTTP requests from within a Python program.
In addition to installing packages, pip
can also be used to upgrade or uninstall packages. For example:
pip install --upgrade <package_name>
To uninstall a package, you would type: pip uninstall <package_name>
Overall, pip
is a powerful tool that makes it easy to manage packages and dependencies in Python. With its many features and capabilities, it's an essential part of any Python programmer's toolkit!