How to automate test cases in Selenium Python?
How to automate test cases in Selenium Python?
To automate test cases using Selenium with Python, you can follow these steps:
Step 1: Install Selenium
First, you need to install Selenium. You can do this by running the following command in your terminal:
pip install selenium
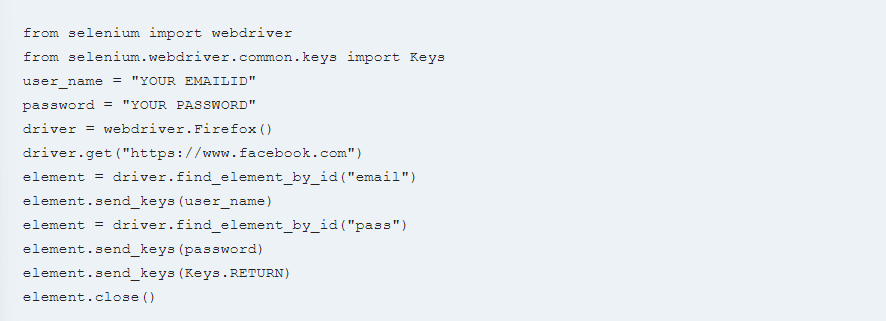
This will install the Selenium WebDriver package for Python.
Step 2: Choose a Browser
Next, you need to choose which browser you want to test with. Selenium supports several browsers including Chrome, Firefox, Edge, and Safari. You can download the corresponding driver executable (e.g., ChromeDriver for Google Chrome) and place it in your project directory or specify the path to the executable when creating the WebDriver instance.
Step 3: Write Your Test Code
Now that you have Selenium installed and a browser chosen, it's time to write your test code. This involves creating a Python class with methods that represent individual test cases. Each test case should cover specific scenarios or functionality in your web application.
For example:
import unittest
from selenium import webdriver
class TestLogin(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome()
def test_login_success(self):
Navigate to the login page
driver = self.driver
driver.get("https://example.com/login")
Enter valid credentials
driver.find_element_by_id("username").send_keys("valid_username")
driver.find_element_by_id("password").send_keys("valid_password")
Submit the form
driver.find_element_by_name("submit").click()
Verify that the user is logged in
assert driver.title == "Logged In"
driver.quit()
def test_login_failure(self):
Navigate to the login page
driver = self.driver
driver.get("https://example.com/login")
Enter invalid credentials
driver.find_element_by_id("username").send_keys("invalid_username")
driver.find_element_by_id("password").send_keys("invalid_password")
Submit the form
driver.find_element_by_name("submit").click()
Verify that the user is not logged in
assert driver.title == "Invalid Credentials"
driver.quit()
def tearDown(self):
self.driver.quit()
if name == "main":
unittest.main()
In this example, we have a TestLogin
class with two test methods: test_login_success
and test_login_failure
. Each method represents a specific login scenario and covers the corresponding functionality in your web application.
Step 4: Run Your Tests
Finally, you can run your tests using the unittest.main()
function. This will execute each test method in sequence and report any failures or errors.
Tips:
Make sure to import the necessary modules (e.g.,selenium
, unittest
) at the top of your script. Use meaningful variable names and comments to make your code easier to understand. Consider using a test framework like Behave or Pytest for more advanced testing scenarios. Always clean up after each test by closing the browser window to avoid leaving unwanted tabs open.
By following these steps, you can write effective test cases using Selenium with Python. Happy testing!
Python web automation without Selenium
I'll respond in English for this special request.
Python web automation without Selenium? That's an interesting topic! While Selenium is a popular choice for automating web browsers, there are alternative approaches you can take to automate websites using Python. Here are some options:
1. Requests and Beautiful Soup
You can use the requests
library to send HTTP requests to a website and then parse the HTML response using Beautiful Soup
. This approach allows you to extract specific data or perform simple actions like submitting forms.
Here's an example of sending a POST request with form data:
import requests
url = "https://example.com/form"
data = {"username": "john", "password": "hello"}
response = requests.post(url, data=data)
if response.status_code == 200:
print("Form submitted successfully!")
else:
print("Error submitting form:", response.text)
2. PyQuery
PyQuery is a Python port of jQuery, which allows you to manipulate and query HTML documents using CSS selectors. You can use it to extract specific elements or perform actions like clicking buttons.
Here's an example of extracting all links on a webpage:
import pyquery as pq
url = "https://example.com"
response = requests.get(url)
doc = pq.PyQuery(response.text)
links = doc("a")
print([link.text() for link in links])
3. Scrapy
Scrapy is a full-fledged web crawling framework that allows you to extract data from websites using XPath expressions or CSS selectors. You can use it to scrape data, submit forms, and more.
Here's an example of scraping titles from a website:
import scrapy
class TitleScraper(scrapy.Spider):
name = "title_scraper"
start_urls = ["https://example.com"]
def parse(self, response):
for title in response.css("h1"):
yield {"title": title.get()}
4. Playwright
Playwright is a Python library that allows you to automate browsers using the Chromium engine. It's similar to Selenium but with some key differences.
Here's an example of opening a browser and navigating to a website:
import playwright
browser = playwright.chromium.launch()
page = browser.new_page()
page.goto("https://example.com")
print(page.title())
Close the browser
browser.close()
These are just a few examples of Python web automation without Selenium. Each approach has its own strengths and weaknesses, so it's essential to choose the right one depending on your specific use case.
Do you have any specific requirements or questions about these approaches?