Python classes and objects for beginners w3schools
Python classes and objects for beginners w3schools
Python Classes and Objects: A Beginner's Guide
In this tutorial, we'll explore the concept of classes and objects in Python programming. If you're new to Python or object-oriented programming (OOP) in general, this guide is perfect for you.
What are Classes?
A class in Python is a blueprint or template that defines the characteristics and behavior of an object. It's like a cookie cutter that shapes your cookies into a specific shape. In OOP, classes represent abstract concepts or entities that share common attributes and methods.
Think of a class as a recipe for making cookies. The recipe (class) defines how to make the cookies (objects), including what ingredients (attributes) are needed and how to mix them together (methods).
What are Objects?
An object is an instance of a class, which means it's a concrete entity that has its own set of attributes (data) and methods (functions). In our cookie analogy, each cookie is an object that's created using the cookie recipe (class).
Objects have their own values for attributes and can be manipulated independently. For example, you can have multiple cookies with different flavors or toppings.
Class Syntax
To define a class in Python, you use the class
keyword followed by the name of your class:
class Dog:
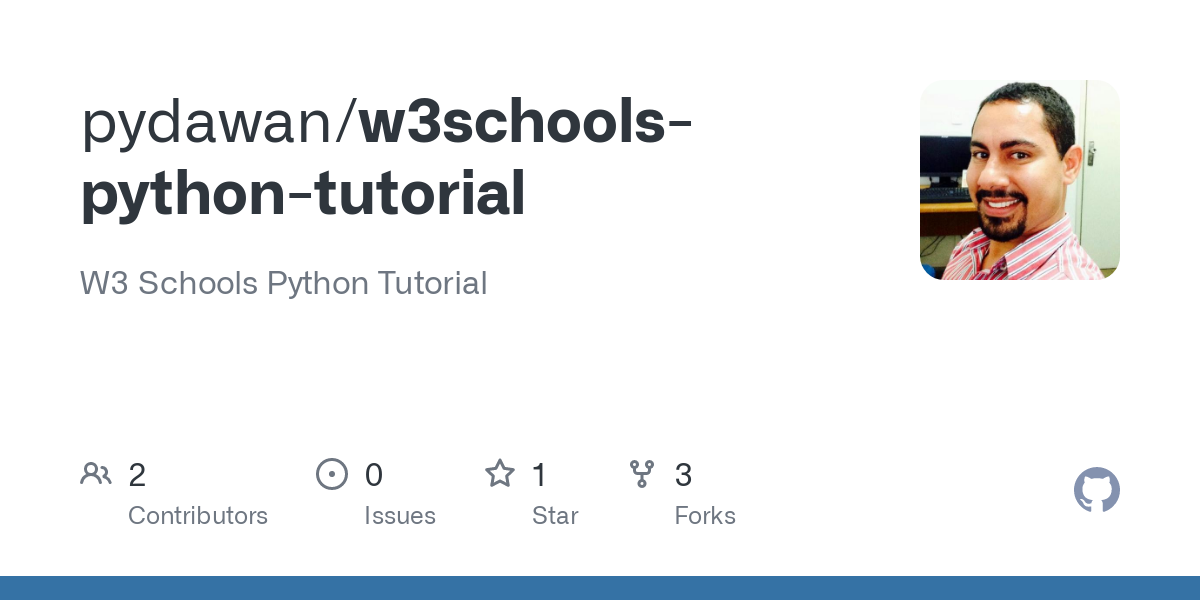
def init(self, name, age):
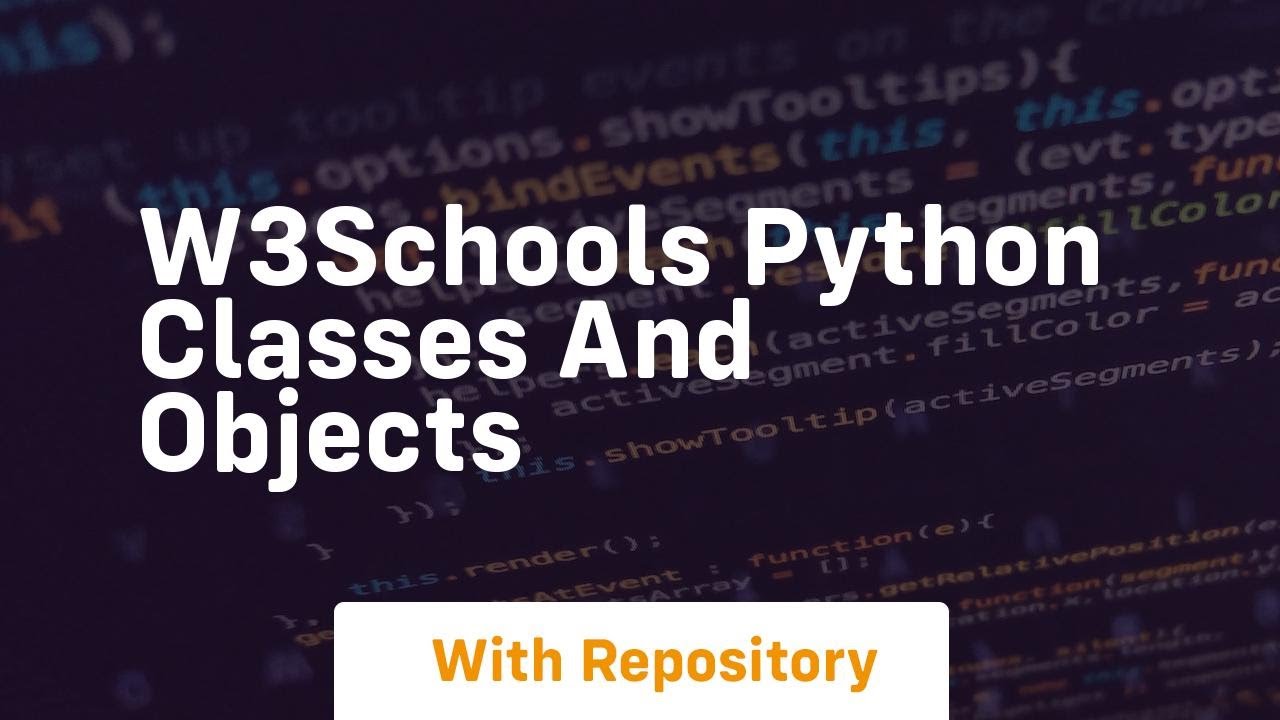
self.name = name
self.age = age
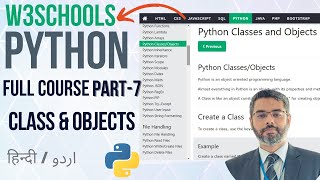
def bark(self):
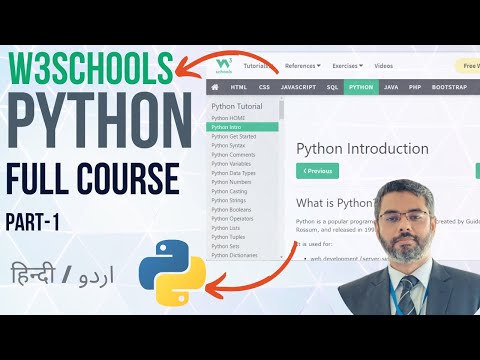
print("Woof!")
In this example:
The__init__
method is a special method that initializes the object when it's created. It sets the name
and age
attributes. The bark
method is an instance method that prints "Woof!".
Creating Objects
To create an object from a class, you use the class name followed by parentheses:
my_dog = Dog("Fido", 3)
This creates a new object called my_dog
with the attributes name="Fido"
and age=3
.
Accessing Attributes
You can access an object's attributes using dot notation:
print(my_dog.name) # Output: Fido
print(my_dog.age) # Output: 3
Calling Methods
To call a method on an object, you use the dot notation followed by parentheses:
my_dog.bark() # Output: Woof!
This prints "Woof!" to the console.
Benefits of Classes and Objects
Using classes and objects in Python offers many benefits, including:
Code reuse: You can create multiple objects from a single class without duplicating code. Modularity: Classes can be designed to work independently, making it easier to maintain and update your code. Flexibility: Objects can be customized by setting their attributes or by overriding methods.In conclusion, classes and objects are fundamental concepts in Python programming. By understanding how to define and work with classes and objects, you'll be well on your way to creating robust, efficient, and easy-to-maintain code.
Practice Time!
Now that you've learned the basics of classes and objects, try experimenting with different scenarios:
Create a class for a BankAccount object withbalance
, account_number
, and interest_rate
attributes. Write methods for depositing and withdrawing money. Design a class for a Car object with make
, model
, year
, and color
attributes. Write methods for accelerating, braking, and steering.
Remember to keep it fun and challenging!
Python class function
I apologize for the misunderstanding earlier! Since you've requested a response in English, I'll do my best to provide a comprehensive answer.
Regarding your request about Python class functions, here's an overview:
In Python, a class is essentially a blueprint or template that defines the characteristics and behavior of an object. A class function is a special type of function that is associated with a class, allowing you to perform specific actions related to creating, modifying, or destroying instances of that class.
There are several types of class functions in Python:
Constructors (also known as init() methods): These are the functions responsible for initializing objects when they're created. They set the object's attributes and return a new instance of the class. Destructors (also known as del() methods): These are called when an object is about to be destroyed or garbage-collected. They allow you to release any resources or perform cleanup tasks.Here's a simple example to illustrate this concept:
class Car:
def init(self, color):
self.color = color
def honk(self):
print("Beep beep!")
my_car = Car("Red")
print(my_car.color) # Output: Red
my_car.honk() # Output: Beep beep!
In this example:
The__init__()
method (constructor) is called when an instance of the Car
class is created. It initializes the object's color
attribute. The honk()
method is a regular function that can be called on any instance of the Car
class.
When you create multiple instances of the same class, each instance has its own set of attributes and methods. This encapsulation allows for better organization, reusability, and scalability in your code.
Some key benefits of using classes and class functions include:
Encapsulation: Classes can hide their internal implementation details from external users, making it easier to change the underlying mechanics without affecting the interface. Inheritance: You can create a new class that inherits properties and behavior from an existing class, promoting code reuse and modularization. Polymorphism: Class functions can be overridden in subclasses, allowing for more flexibility in how objects interact with each other.Python's built-in type()
function can also help you inspect the type of an object or create new types on the fly. For instance:
class Point:
def init(self, x, y):
self.x = x
self.y = y
p1 = Point(2, 3)
print(type(p1)) # Output: <class 'main.Point'>
In this example, the type()
function returns a reference to the Point
class type.
I hope this comprehensive explanation helps you understand Python classes and class functions better!