Why is Python function defined?
Why is Python function defined?
I'm happy to explain why functions are defined in Python!
In programming languages like Python, a function is a block of code that can be executed repeatedly with different inputs and outputs. Functions are used to encapsulate a specific task or logic within a program, making it reusable and easier to maintain.
There are several reasons why functions are defined:
Modularity: Functions allow you to break down a large program into smaller, independent units of code that can be easily managed and reused. This makes it simpler to understand, test, and modify the code. Reusability: By defining a function once, you can use it multiple times throughout your program without having to rewrite the same code over and over again. This reduces code duplication and saves time. Abstraction: Functions provide an abstraction layer between the low-level implementation details and the high-level logic of your program. This means that the internal workings of a function are hidden from the rest of the code, making it easier to change or replace without affecting other parts of the program. Code organization: Functions help keep your code organized by grouping related functionality together. This makes it easier to find and modify specific parts of your program. Input/output management: Functions can be used to manage input and output data more efficiently. For example, a function might read input from a file or user, perform some processing on the data, and then write the results back to the same location. Error handling: By encapsulating complex logic within a function, you can catch and handle errors more effectively. This helps prevent errors from propagating up the call stack and making it harder to debug your program. Improved maintainability: When code is organized into functions, it becomes easier to modify or extend individual parts of the program without affecting other areas.In Python specifically, defining a function is achieved using the def
keyword followed by the function name and parameters in parentheses. For example:
def greet(name: str) -> None:
print(f"Hello, {name}!")
This defines a function called greet
that takes a string parameter name
and returns no value (-> None
). The function prints out a greeting message with the input name.
Functions are an essential aspect of programming in Python (and many other languages), allowing you to write more efficient, readable, and maintainable code.
What does it mean function in Python?
I'm glad you asked!
In Python, a function
is a block of code that can be executed multiple times from different parts of your program. Think of it like a recipe: you can follow the steps to make a cake, and then use those same steps to bake cookies or muffins. Functions are reusable chunks of code that can take arguments (inputs) and return values (outputs).
Here's a basic structure for a Python function:
def my_function(arg1, arg2):
do something with the args
result = some_calculation()
return result
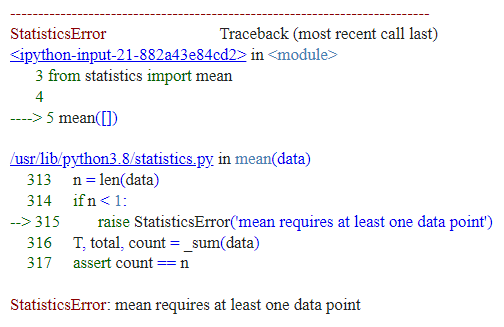
Let's break it down:
def
: This is the keyword used to define a new function. my_function
: This is the name of the function. You can choose any valid Python identifier (e.g., hello_world
, sum_numbers
, etc.). (arg1, arg2)
: These are the input parameters or arguments that the function takes. In this case, we're expecting two values, arg1
and arg2
. The code inside the function is where you write your logic to process the inputs and return a result. return result
: This line specifies what value the function will return when it's done executing.
When you call a function, Python executes the code inside the function, passing in any arguments you provided. For example:
result = my_function(2, 3)
print(result) # might print something like 5 or "hello"
Functions have several benefits:
Modularity: Break down your program into smaller, reusable pieces that can be easily maintained and updated. Reusability: Use a single function in multiple parts of your code to avoid duplicating logic. Code readability: Functions help organize your code by grouping related logic together. Arguments and returns: Functions allow you to pass input values (arguments) and receive output values (returns), making it easier to work with complex data.Some common types of functions in Python include:
Pure functions: Take inputs, perform calculations, and return a result without modifying external state. Impure functions: Modify external state or rely on side effects (e.g., accessing the file system). Higher-order functions: Accept other functions as arguments or return functions from within their execution.Now that you know what functions are in Python, I hope you'll start using them to make your code more efficient and maintainable!