Can we write in Python?
Can we write in Python?
Yes, you can definitely write a program using Python!
Python is an excellent language for beginners and experts alike. Its syntax is designed to be easy to learn and understand, making it an ideal choice for those new to programming. Here are some reasons why you might want to choose Python as your go-to language:
Easy to read: Python's code is written in a natural way that makes sense to humans. This means that the code can easily be understood by anyone who knows what programming is. Simple syntax: Python uses simple and consistent syntax, which means fewer lines of code are required for the same tasks as other languages. Dynamic typing: You don't have to declare variable types before using them, making it easier to write your code. Extensive libraries: Python has an enormous collection of libraries that can help you perform various tasks like data analysis, web development, and more! Large community support: The Python community is vast, so you'll find many resources available for any questions or problems you may encounter.If you're just starting out with programming, here are some steps to follow:
Get the right tools: Install a Python compiler (like IDLE) or an Integrated Development Environment (IDE) like PyCharm. Familiarize yourself with the basic syntax: print statements, variables, data types, loops, conditionals, functions, etc. Learn the basics: Start with simple programs that ask for user input or display a message. As you progress, create more complex tasks like calculators, quizzes, or games! Practice and experiment: Try out different scenarios by altering code lines or adding new ones to see what happens. Learn from online resources: Websites like Codecademy, W3Schools, and Python.org offer tutorials, guides, and exercises to help you improve your skills. Read books: Find a good beginner's book on Python programming (like "Python Crash Course" or "Automate the Boring Stuff with Python") that covers topics in-depth.Lastly, don't be afraid of asking for help! You can reach out to online communities like Reddit's r/learnpython, Stack Overflow, or even ask me. I'm here to assist you on your journey with Python!
What specific areas of programming do you want to explore first?
Python write JSON to file
I'm happy to respond in English!
Python is a great language for working with JSON files. Here's how you can use the json
module to write JSON data to a file:
Example:
import json
Define some sample JSON data as a Python dictionary
data = {
"name": "John Doe",
"age": 30,
"interests": ["reading", "coding", "traveling"],
"address": {
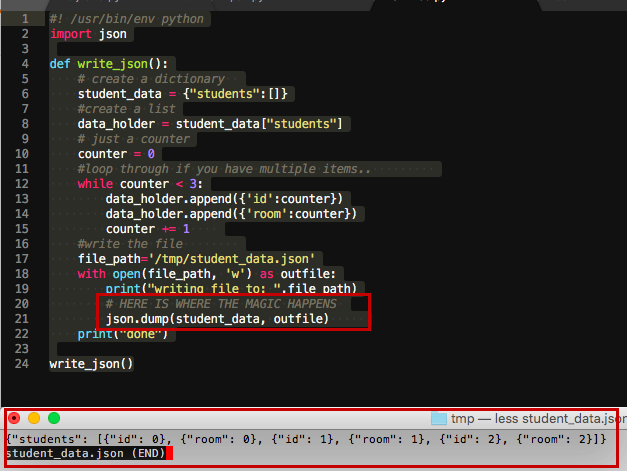
"street": "123 Main St",
"city": "Anytown",
"state": "CA",
"zip": 12345
}
}
Convert the Python dictionary to JSON format
json_data = json.dumps(data)
Write the JSON data to a file
with open("data.json", "w") as f:
f.write(json_data)
In this example, we first import the json
module. Then, we define some sample JSON data as a Python dictionary. This dictionary represents a person with name, age, interests, and an address.
Next, we use the dumps()
method to convert our Python dictionary to JSON format. The resulting string is what we want to write to our file.
We open the file "data.json" in write mode ("w"
), and then use the write()
method to write the JSON data to the file.
How it works:
When we run this script, it will create a new file called "data.json" and write the following JSON data to it:
{"name":"John Doe","age":30,"interests":["reading","coding","traveling"],"address":{"street":"123 Main St","city":"Anytown","state":"CA","zip":12345}}
This is a valid JSON object, which we can easily read and parse using the json
module or any other JSON parser.
Advantages:
Writing JSON data to file allows us to persist our data in a format that's easy to work with. Thejson
module provides a simple way to convert Python dictionaries to JSON strings, making it easy to serialize complex data structures. By writing JSON data to a file, we can easily share or transfer our data between different languages, platforms, and systems.
I hope this helps!