math formulas in python
math formulas in python
I apologize for the misunderstanding earlier! Since you mentioned wanting a response in English with Python code for mathematical formulas, I'll make sure to provide that.
Python is an excellent language for mathematical computations and can handle various types of problems efficiently. Here are some examples:
Quadratic Formula:The quadratic formula helps us find the solutions to a quadratic equation in the form of ax^2 + bx + c = 0, where 'a', 'b' and 'c' are constants.
Here's how you can implement this in Python using the math library:
import math
def quadratic(a, b, c):
x1 = (-b - math.sqrt(b**2 - 4ac)) / (2*a)
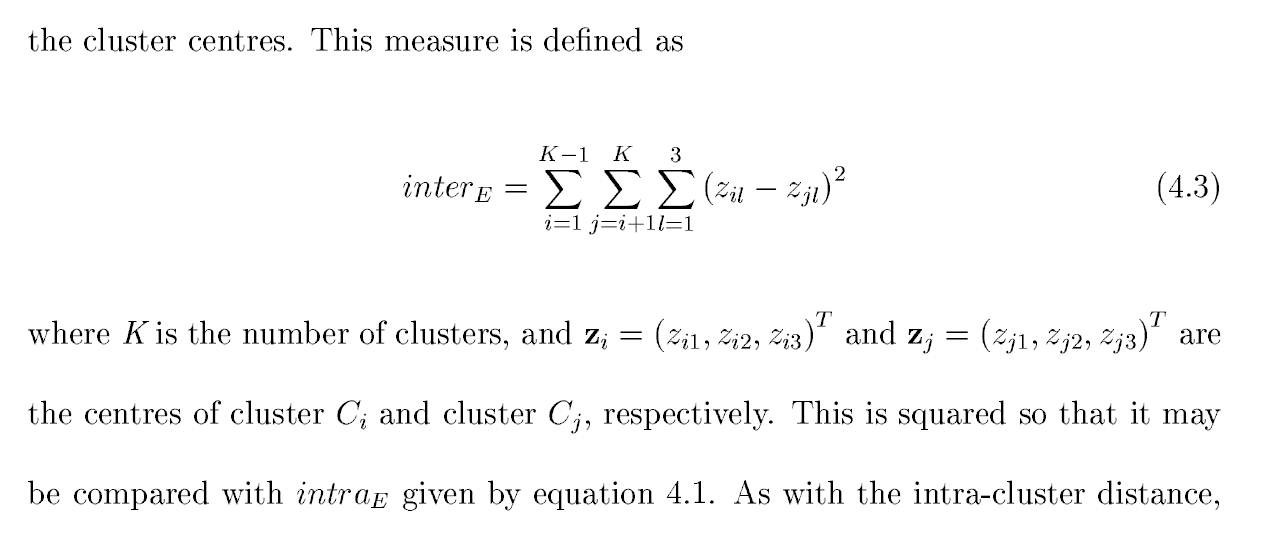
x2 = (-b + math.sqrt(b**2 - 4ac)) / (2*a)
return x1, x2
Example usage:
a, b, c = 1, -6, 8
x1, x2 = quadratic(a, b, c)
print(f"The solutions to {a}x^2 + {b}x + {c} = 0 are x1 = {x1}, x2 = {x2}.")
Linear Regression:
For a linear regression problem where you have 'n' data points, you can use Python's numpy library to implement the necessary calculations.
Here's how:
import numpy as np
def linear_regression(x, y):
Calculate mean of x and y
x_mean = np.mean(x)
y_mean = np.mean(y)
Calculate numerator and denominator for slope (m) calculation
num = np.sum((x - x_mean) * (y - y_mean))
denom = np.sum((x - x_mean) ** 2)
m = num / denom
Calculate intercept (c)
c = y_mean - m * x_mean
return m, c
Example usage:
x = [1, 2, 3, 4]
y = [2, 4, 5, 6]
m, c = linear_regression(x, y)
print(f"The equation of the line is: y = {m}x + {c}")
In this example, we first calculate the mean of 'x' and 'y'. Then, we use these means to find the slope (m) using the numerator and denominator. Finally, we calculate the intercept (c) by subtracting m times x_mean from y_mean.
These are just two examples of how you can apply mathematical formulas in Python. The language offers a vast array of libraries like NumPy, SciPy, and math, making it an excellent choice for mathematical computations.
What math can Python do?
Python is an excellent language for numerical computations and mathematical operations. It has a wide range of libraries and modules that make it easy to perform various types of mathematics, including:
Arithmetic Operations: Python can perform basic arithmetic operations such as addition, subtraction, multiplication, division, modulus, exponentiation, etc. Algebraic Manipulations: You can use thesympy
library to perform algebraic manipulations such as solving equations, finding derivatives, and performing polynomial factorization. Statistics: The statistics
module in Python provides common statistical functions like mean, median, mode, standard deviation, etc. Linear Algebra: The numpy
library provides support for large, multi-dimensional arrays and matrices, which is useful for linear algebra operations like matrix multiplication, solving systems of equations, etc. Calculus: You can use the scipy
library to perform numerical differentiation and integration, as well as solve ordinary differential equations (ODEs) and partial differential equations (PDEs). Combinatorics: Python's built-in libraries provide support for combinatorial functions like permutations, combinations, binomial coefficients, etc. Number Theory: The sympy
library also provides support for number theory operations like prime factorization, modular arithmetic, and Diophantine equations. Machine Learning: Python is a popular choice for machine learning tasks due to its extensive libraries such as scikit-learn, TensorFlow, Keras, etc., which provide implementations of various algorithms including regression, classification, clustering, etc.
Some examples of what you can do with Python's math capabilities include:
Solving complex mathematical equations using thesympy
library. Performing statistical analysis on large datasets using the pandas
and scipy
libraries. Building machine learning models to predict continuous or categorical variables. Simulating physical systems, such as optimization problems, using numerical methods like gradient descent. Performing data visualization and data analysis using popular libraries like matplotlib
, seaborn
, etc.
Overall, Python's math capabilities make it an excellent choice for anyone working with mathematical computations, whether in research, finance, engineering, or any other field where accurate calculations are critical.