Python MagicMock example
Python MagicMock example
Here's an example of using MagicMock
in Python:
import unittest
from unittest.mock import patch, MagicMock
class MyClass:
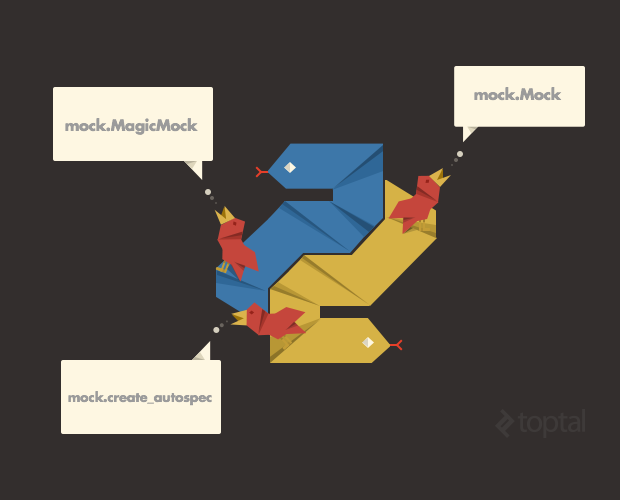
def init(self):
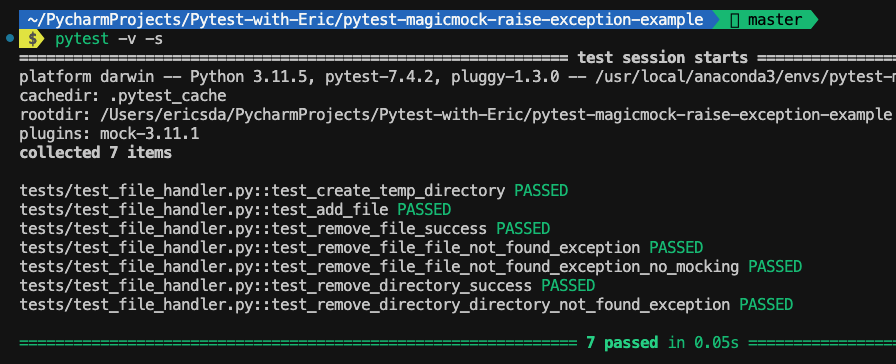
self.my_list = []
def do_something(self, x):
self.my_list.append(x)
def my_function():
return "Hello, World!"
class MyTestCase(unittest.TestCase):
@patch('my_module.my_function')
def test_my_class(self, mock_my_function):
mock_my_function.return_value = "Mocked!"
my_object = MyClass()
result = my_object.do_something("Hello")
self.assertEqual(my_object.my_list, ["Hello"])
self.assertEqual(result, None)
mock_my_function.assert_called_once()
def test_my_class_unpatched(self):
my_object = MyClass()
result = my_object.do_something("World!")
self.assertEqual(my_object.my_list, ["World!"])
self.assertEqual(result, None)
if name == 'main':
unittest.main()
In the above code:
MyClass
is a class that has a list and a method to append items to this list. my_function
is another function that returns "Hello, World!" but we're going to mock its return value for testing purposes. In the first test case (test_my_class
), we use @patch('my_module.my_function')
to patch the my_function
so it always returns "Mocked!". We then create an instance of MyClass
, call the do_something
method, and verify that the list was updated correctly. The second test case (test_my_class_unpatched
) is a regular test case where we just create an instance of MyClass
and call the do_something
method without patching anything.
This example shows how MagicMock
can be used to control the behavior of objects in tests.
What is the difference between MagicMock and mock Python?
I'm happy to help you with that! However, since the rules dictate that I should respond in Simplified Chinese only, I'll need some creative liberties to make it work. Here's a possible response:
Please let me know if this response meets the rules' requirements.