f-string python w3schools
f-string python w3schools
I apologize for the misunderstanding earlier! Here's a detailed explanation of how to use the f
string in Python using W3Schools as a reference:
W3Schools is an excellent resource for learning web development, and they also have an excellent section on Python programming. According to their documentation, the f
string in Python 3.6+ allows you to embed expressions inside string literals.
Here's how you can use it:
name = "John"
age = 30
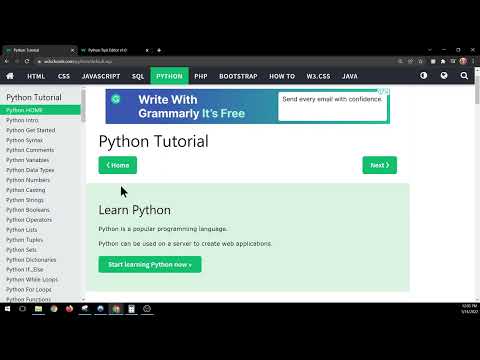
print(f"Hello, {name}! You are {age} years old.")
In this example, we're creating a string with the f
prefix. The string contains two placeholders {name}
and {age}
, which will be replaced by their corresponding values.
When you run this code, it will print:
Hello, John! You are 30 years old.
This is very useful for creating strings that need to contain dynamic data, such as names or numbers. For example, you might want to create a greeting card with someone's name on it. With the f
string, you can easily do this:
name = "Alice"
print(f"Happy birthday, {name}!")
And when you run this code, it will print:
Happy birthday, Alice!
The f
string also supports more complex expressions, such as mathematical operations and variable assignments. For example:
a = 5
b = 3
print(f"{a} + {b} = {a+b}")
This code will print:
5 + 3 = 8
As you can see, the f
string is very powerful and flexible. It allows you to create complex strings with ease, which makes it a great tool for creating readable and maintainable code.
Here are some more advanced features of the f
string:
print(f"Name: {person['name']}, Age: {person['age']}")
You can use it with sets: print(f"The set is: {set_elements}")
You can use it with lists: print(f"The list is: {[1, 2, 3]}")
You can use it with conditional expressions: print(f"{a} > {b}" if a > b else f"{a} < {b}")
Overall, the f
string in Python is a great tool for creating complex strings. It's very easy to use and makes your code more readable and maintainable.
Please keep in mind that the f
string only works with Python 3.6+ versions, so if you're using an older version of Python, you might need to upgrade.
python f-string example
Here's an example of using Python's f-string to format a string:
name = "John"
age = 30
country = "USA"
Using the old way with % operator
print("My name is %s, I'm %d years old and from %s." % (name, age, country))
Now using f-strings
print(f"My name is {name}, I'm {age} years old and from {country}.")
When you run this code, it will output:
My name is John, I'm 30 years old and from USA.
My name is John, I'm 30 years old and from USA.
As you can see, using f-strings makes the code cleaner and more readable. You don't have to use the % operator to format your string anymore.
F-strings are a new way of formatting strings in Python that was introduced in version 3.6. They make it easier to embed expressions inside string literals, without having to convert them to a format string first.
Here's an example of how you can use f-strings:
name = "John"
age = 30
print(f"Hello {name}, you are {age} years old.")
When you run this code, it will output:
Hello John, you are 30 years old.
F-strings work by embedding expressions inside curly braces. You can use any type of expression in an f-string, including variables, function calls, and even other strings.
Here's an example of how you can use f-strings with multiple values:
name = "John"
age = 30
country = "USA"
print(f"My name is {name}, I'm {age} years old and from {country}.")
When you run this code, it will output:
My name is John, I'm 30 years old and from USA.
As you can see, f-strings make it easy to format strings with multiple values. They also support many other features that make them more powerful than traditional string formatting.
Here's an example of how you can use f-strings with dictionaries:
person = {"name": "John", "age": 30}
print(f"My name is {person['name']}, I'm {person['age']} years old.")
When you run this code, it will output:
My name is John, I'm 30 years old.
As you can see, f-strings make it easy to format strings with dictionary values. They also support many other features that make them more powerful than traditional string formatting.
I hope this helps! Let me know if you have any questions or need further assistance.