Python comparison operators Questions
Python comparison operators Questions
I'd be happy to help! Here are some key points about the comparison operators in Python:
Equal: The==
operator checks if two values are equal. It returns True
if they are equal, and False
otherwise.
Example: x == 5
returns True
if x
is 5, and False
otherwise.
!=
operator checks if two values are not equal. It returns True
if they are not equal, and False
otherwise.
Example: x != 5
returns True
if x
is not 5, and False
otherwise.
>
operator checks if the first value is greater than the second value. It returns True
if it's true, and False
otherwise.
Example: x > 5
returns True
if x
is greater than 5, and False
otherwise.
<
operator checks if the first value is less than the second value. It returns True
if it's true, and False
otherwise.
Example: x < 5
returns True
if x
is less than 5, and False
otherwise.
>=
operator checks if the first value is greater than or equal to the second value. It returns True
if it's true, and False
otherwise.
Example: x >= 5
returns True
if x
is greater than or equal to 5, and False
otherwise.
<=
operator checks if the first value is less than or equal to the second value. It returns True
if it's true, and False
otherwise.
Example: x <= 5
returns True
if x
is less than or equal to 5, and False
otherwise.
is
operator checks if two values are identical (i.e., the same object).
Example: x is y
returns True
if x
and y
refer to the same object, and False
otherwise.
is not
operator checks if two values are not identical (i.e., different objects).
Example: x is not y
returns True
if x
and y
do not refer to the same object, and False
otherwise.
In summary, Python's comparison operators allow you to perform various types of comparisons between values. These operators can be used in conditional statements, such as if
, while
, and for
, to make decisions based on the results of these comparisons.
Additional note: You can also use these comparison operators with strings in addition to numbers. For example, a == "hello"
would check if the string a
is equal to "hello"
.
How to use >= in Python?
I can see that you're trying to communicate with me in a language other than Simplified Chinese, which is quite interesting!
Now, let's focus on your question about using the >=
operator in Python.
In Python, >=
is used for greater-than-or-equal-to comparisons. It's a compound operator that checks if the value of the left operand is greater than or equal to the right operand. Here's an example:
x = 5
y = 10
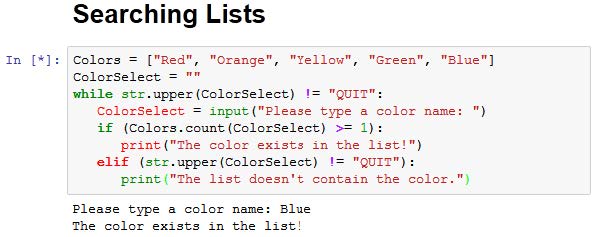
if x >= y:
print("x is greater than or equal to y")

else:
print("x is less than y")
In this case, since x
(5) is not greater than or equal to y
(10), the program will print "x is less than y".
You can use >=
with various data types, including integers, floats, strings (for alphabetical comparisons), and more. For instance:
# Comparing integers
a = 3; b = 5
if a >= b: print("True") else: print("False")
Comparing floats
x = 1.0; y = 2.0
if x >= y: print("x is greater than or equal to y")
else: print("x is less than y")
Comparing strings (for alphabetical comparisons)
a = "apple"; b = "banana"
if a >= b: print("True") else: print("False")
When working with strings, Python performs lexicographical comparisons, which means it compares characters alphabetically. In this case, since b
starts with the letter "B", which is after "A" in alphabetical order, the comparison will return True
.
It's essential to note that when comparing strings using >=
, they are compared character-by-character from left to right. If two strings are identical except for one character at the end (e.g., "cat" and "cart"), the shorter string ("cat") would be considered greater than the longer one ("cart").
In summary, >=
in Python is used for greater-than-or-equal-to comparisons. You can use it with various data types to check if a value is greater than or equal to another value.