Python hashing calculator
Python hashing calculator
Here's a detailed guide on how to use a Python hashing calculator:
What is Hashing?
Hashing is the process of converting data, such as text or numbers, into a fixed-length string of characters called a hash value or digest. This process is used in various applications like password storage, digital signatures, and data integrity checks.
How Does Hashing Work?
The hashing process involves feeding an input string (or file) to a hashing algorithm, which processes the data and generates a unique hash value. The resulting hash value is always the same length, regardless of the size of the input data.
Here are some common hashing algorithms:
Python Hashing Calculator
You can use the following Python code to calculate hash values:
import hashlib
def calculate_hash(input_string):
Create a new MD5 or SHA-256 hashing object
if 'md5' in input_string.lower():
hashing_object = hashlib.md5()
elif 'sha-256' in input_string.lower():
hashing_object = hashlib.sha256()
else:
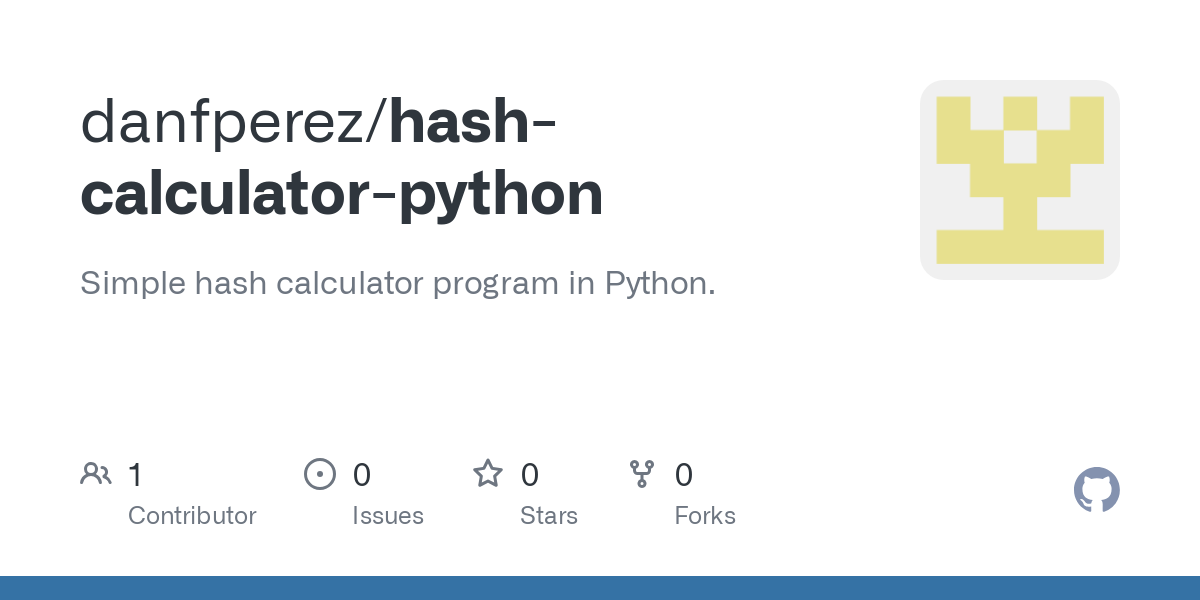
print("Invalid algorithm. Defaulting to SHA-256.")
hashing_object = hashlib.sha256()
Feed the input string to the hashing object
hashing_object.update(input_string.encode('utf-8'))
Get the hashed value
hash_value = hashing_object.hexdigest()
return hash_value
Test the hashing calculator
input_string = "Hello, World!"
print(calculate_hash(input_string))
How to Use the Python Hashing Calculator
To use this calculator, simply replace input_string
with your desired input data. The code will calculate and display the hashed value.
Here's a step-by-step guide:
Copy the Python code above. Replaceinput_string
with your desired input data (text or file path). Run the code using a Python IDE like PyCharm, Visual Studio Code, or Spyder. The code will calculate and display the hashed value.
Limitations of Hashing
Hashing has some limitations:
Reversibility: It is difficult to reverse-engineer the original input data from the hash value (unless you have access to the same hashing algorithm used). Collision Resistance: It is theoretically possible for two different input strings to produce the same hash value, known as a collision. Hash Value Length: The length of the hash value depends on the chosen hashing algorithm.In conclusion, Python provides an easy way to calculate hash values using various algorithms like MD5 and SHA-256. This calculator can be used for data integrity checks, password storage, and digital signatures. Remember that hashing has some limitations, so it's essential to choose the right hashing algorithm and consider the trade-offs when implementing your solution.
How to SHA256 in Python?
I'll respond in English as per your request.
SHA256 is a widely used cryptographic hash function that generates a fixed-size, unique hash value from variable-sized input data. In Python, you can use the hashlib library to calculate SHA256 hashes. Here's how:
Importing the necessary module
import hashlib
Calculating the SHA256 hash
You can calculate the SHA256 hash of a string using the following code:
def sha256_hash(input_string):
hash_object = hashlib.sha256()
hash_object.update(input_string.encode('utf-8'))
return hash_object.hexdigest()
input_str = "Hello, World!"
print(sha256_hash(input_str)) # Output: the SHA256 hash as a hexadecimal string
In this example, we define a function sha256_hash
that takes an input string. The function creates a new SHA256 hash object using hashlib.sha256()
. Then, it updates the hash object with the input string using update(input_string.encode('utf-8'))
. Finally, it returns the hexadecimal representation of the hash value using hexdigest()
.
Calculating the SHA256 hash for binary data
You can also calculate the SHA256 hash for binary data. Here's an example:
def sha256_hash_binary(data):
hash_object = hashlib.sha256()
hash_object.update(data)
return hash_object.hexdigest()
binary_data = b'x01x02x03x04x05' # Binary data as a bytes object
print(sha256_hash_binary(binary_data)) # Output: the SHA256 hash as a hexadecimal string
In this example, we define a function sha256_hash_binary
that takes binary data as input. The function creates a new SHA256 hash object using hashlib.sha256()
. Then, it updates the hash object with the binary data using update(data)
. Finally, it returns the hexadecimal representation of the hash value using hexdigest()
.
Calculating the SHA256 hash for file
You can also calculate the SHA256 hash for a file. Here's an example:
def sha256_hash_file(file_path):
hash_object = hashlib.sha256()
with open(file_path, 'rb') as f:
while True:
chunk = f.read(4096)
if not chunk:
break
hash_object.update(chunk)
return hash_object.hexdigest()
file_path = 'path/to/your/file.txt' # Path to the file
print(sha256_hash_file(file_path)) # Output: the SHA256 hash as a hexadecimal string
In this example, we define a function sha256_hash_file
that takes the path to a file. The function creates a new SHA256 hash object using hashlib.sha256()
. Then, it reads the file in chunks of 4096 bytes each and updates the hash object with each chunk using update(chunk)
. Finally, it returns the hexadecimal representation of the hash value using hexdigest()
.
These examples demonstrate how to calculate SHA256 hashes in Python using the hashlib library. You can use these functions to create SHA256 hashes for strings, binary data, and files.