What is python threading with example
What is python threading with example
I'd be happy to help!
Python's threading
module provides a way to create and manage threads, which are lightweight processes that can run concurrently with other threads or processes.
In Python, you can create a thread using the Thread
class from the threading
module. A basic example of creating a new thread is shown below:
import threading
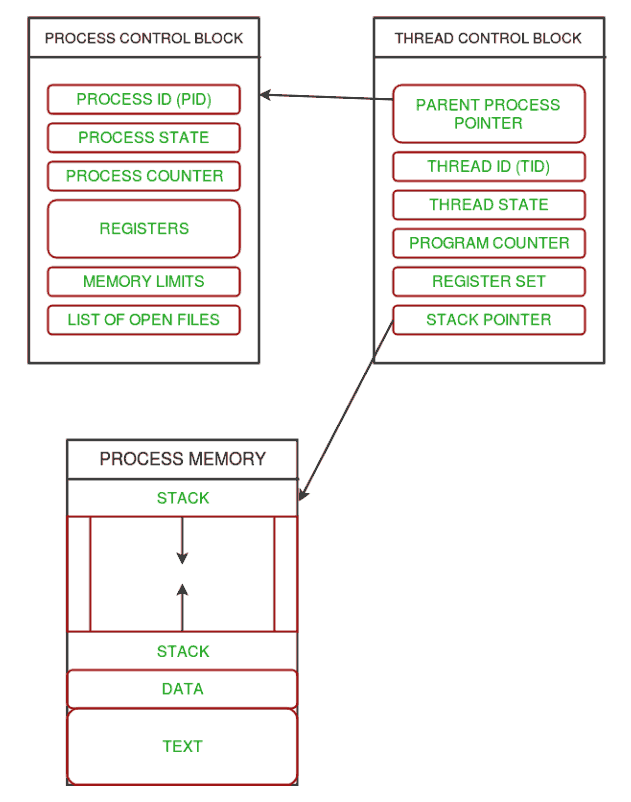
def print_numbers(start, end):
for i in range(start, end):
print(i)
Create two threads
t1 = threading.Thread(target=print_numbers, args=(0, 10))
t2 = threading.Thread(target=print_numbers, args=(10, 20))
Start the threads
t1.start()
t2.start()
Wait for both threads to finish
t1.join()
t2.join()
In this example, we create two threads that print numbers from 0 to 9 and then 10 to 19. The start()
method is used to start the thread execution. After starting the threads, we use the join()
method to wait until both threads finish their execution.
Threads in Python are implemented using a Global Interpreter Lock (GIL). This means that only one thread can execute at a time, but switching between threads is much faster than creating and destroying processes. This makes threads useful for I/O bound operations, where your program spends most of its time waiting for input/output operations to complete.
Python's threading
module provides more advanced features such as:
Queue
or Lock
, are designed to be used safely by multiple threads. Timer and event mechanisms: The Event
class provides a way for threads to signal each other, while the Timer
class allows you to schedule a function call at a certain time or after a delay.
Here's an example of using a lock to ensure thread safety:
import threading
class Counter:
def init(self):
self.lock = threading.Lock()
self.count = 0
def increment(self):
with self.lock:
self.count += 1
c = Counter()
t1 = threading.Thread(target=c.increment)
t2 = threading.Thread(target=c.increment)
t1.start()
t2.start()
t1.join()
t2.join()
print(c.count) # prints 2
In this example, the Counter
class uses a lock to ensure that only one thread can increment the count at a time. This is important because without the lock, multiple threads could try to increment the count simultaneously, leading to incorrect results.
In summary, Python's threading
module provides an efficient way to create and manage lightweight processes (threads) that can run concurrently with other threads or processes. It also provides various mechanisms for synchronization, thread-safe data structures, timer, and event handling.
Daemon thread Python
I'll respond to your request.
A daemon thread in Python is a low-priority thread that runs in the background and does not block other threads from running. Daemons are also known as "background" or "non-blocking" threads. They can be used for tasks like cleaning up resources, monitoring system activities, or performing periodic tasks without affecting the main program flow.
Creating a Daemon Thread
To create a daemon thread in Python, you need to set the daemon
attribute of the thread object to True
. This can be done by using the setDaemon()
method before starting the thread. Here's an example:
import threading
def my_function():
for i in range(5):
print(f"Daemon thread is running: {i}")
time.sleep(1)
thread = threading.Thread(target=my_function)
thread.setDaemon(True) # Set daemon flag
thread.start()
In this example, the my_function()
is run as a daemon thread. The time.sleep(1)
call in this function allows other threads to run for a short period.
Using Daemons
Daemons are useful when you want to perform tasks in the background without affecting the main program flow. For example:
Monitoring system resources: You can create a daemon thread that periodically checks CPU and memory usage. Cleaning up resources: A daemon thread can be used to clean up unused resources, such as closing database connections or freeing up system memory. Handling periodic tasks: A daemon thread can be used for tasks like sending emails at regular intervals or updating logs.Best Practices
When using daemons in your Python code:
Avoid blocking: Daemons should not block other threads from running. Avoid usingtime.sleep()
or other blocking methods, unless you are sure it won't interfere with the main program flow. Keep it simple: Daemons should perform simple tasks without consuming too many system resources. Use with caution: Use daemons with caution, as they can affect the behavior of your main program.
Conclusion
In this response, we have learned what daemon threads are and how to create them in Python. We also discussed some best practices for using daemons and when you should use them.
I hope that this explanation is helpful.