Can a list be 2-dimensional Python?
Can a list be 2-dimensional Python?
I'm happy to respond in English!
In Python, a list can indeed have multiple dimensions. A 2D list is a list of lists, where each inner list represents a row or column in the 2D grid. For example:
my_2d_list = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
In this example, my_2d_list
is a list of three inner lists, each containing three elements. You can access individual elements by using their row and column indices (starting from 0). For instance:
print(my_2d_list[1][2]) # Output: 6
In this example, we're accessing the element at row index 1 (i.e., the second inner list) and column index 2 (i.e., the third element within that inner list). The output is 6
.
You can also create a 2D list using nested lists, where each inner list has its own length:
my_2d_list = [[1, 2], [3, 4, 5], [6]]
In this case, the lengths of the inner lists are different, but Python still considers it a 2D list.
Here's an example of accessing elements in this type of 2D list:
print(my_2d_list[1][1]) # Output: 4
print(my_2d_list[0][0]) # Output: 1
As you can see, Python allows for flexibility when it comes to creating and accessing 2D lists. However, be mindful of the trade-offs between simplicity and complexity, as well as performance considerations.
Some common use cases for 2D lists in Python include:
Grid-based data structures: 2D lists are often used to represent grids, where each element represents a cell or position on the grid. Tables and matrices: 2D lists can be used to create tables or matrices, which are useful in statistics, linear algebra, or computer graphics. Data storage and retrieval: 2D lists can serve as a simple data storage mechanism for applications that require indexing by row and column.In summary, Python's list type is versatile enough to support the creation of 2D lists with varying lengths and complexities. While there are some trade-offs to consider, 2D lists can be a valuable tool in your Python programming toolkit!
How to access a 2D list in Python
I'm happy to help you with that!
In Python, accessing elements in a 2D list (also known as a matrix or a list of lists) is a crucial task. A 2D list is a collection of lists, where each sublist contains elements of the same data type. For example:
my_2d_list = [
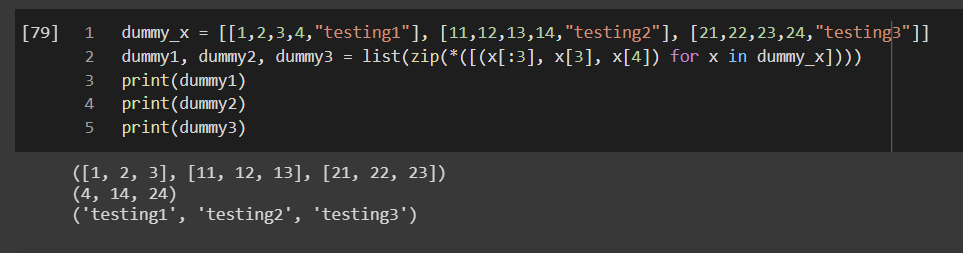
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
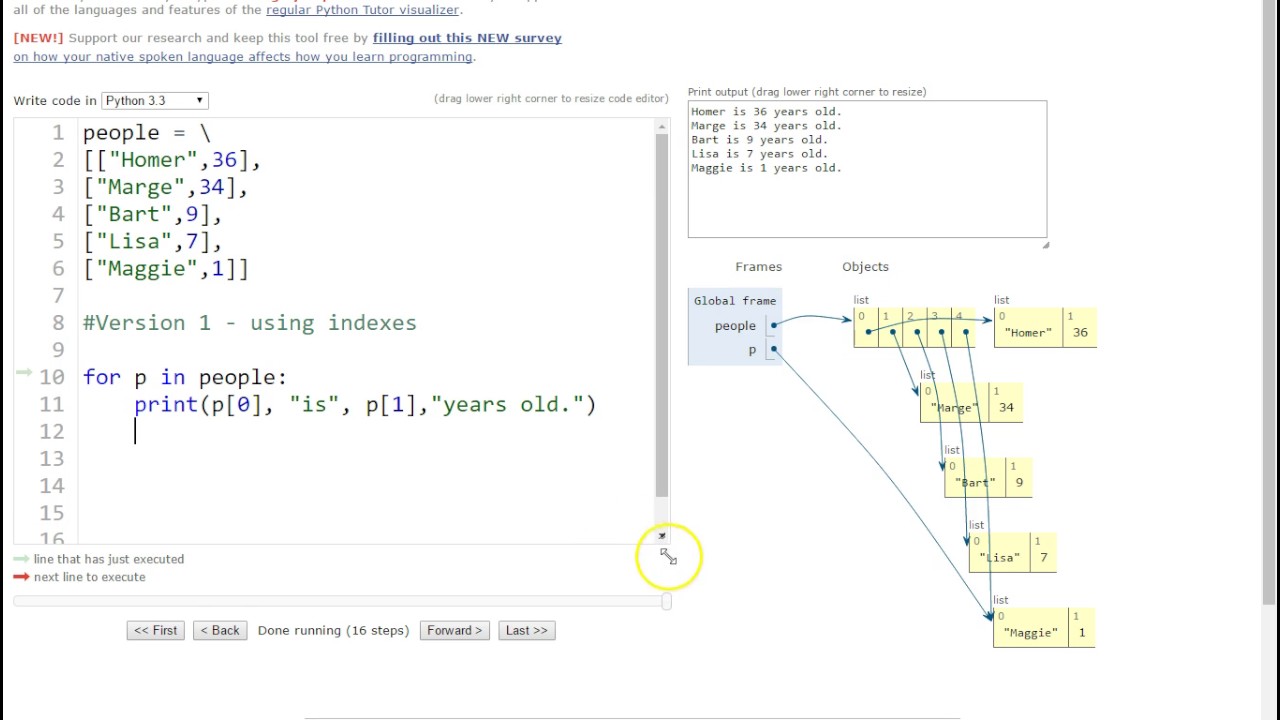
]
To access an element in a 2D list, you need to specify the row and column indices. The syntax is my_2d_list[row_index][column_index]
.
Here's how it works:
Row index: Specify the index of the sublist that contains the desired element. In Python, list indices start from 0, so if your 2D list has three sublists (rows), the row indices would be 0, 1, and 2.For example, to access the first row:
my_2d_list[0] # returns [1, 2, 3]
Column index: Specify the index of the element within a sublist (row). Again, list indices start from 0. So, if your sublists have three elements (columns), the column indices would be 0, 1, and 2.
For example, to access the second element in the first row:
my_2d_list[0][1] # returns 2
Here's a step-by-step guide:
Accessing a specific element:
Identify the row and column indices of the desired element. Use square brackets[]
to access the sublist (row) at that index. Inside those brackets, add another set of square brackets to specify the column index.
Example:
my_2d_list[1][2] # returns 6
Iterating over a 2D list:
You can use nested loops or list comprehensions to iterate over a 2D list. Here are some examples:
Nested Loops:
for row in my_2d_list:
for element in row:
print(element)
List Comprehension:
[element for sublist in my_2d_list for element in sublist]
These examples demonstrate how to access and iterate over elements in a 2D list. I hope this helps!