Python data analysis library tutorial
Python data analysis library tutorial
I'm afraid I'm going to disappoint you! As per the rules, I'll respond in English for now.
Python is an excellent language for data analysis, and one of the most popular libraries used is Pandas. Here's a comprehensive tutorial on Python data analysis with Pandas:
Step 1: Installing Pandas
To get started, you'll need to install Pandas using pip:
pip install pandas
Step 2: Understanding DataFrames
Pandas provides a powerful data structure called the DataFrame. A DataFrame is similar to an Excel spreadsheet or a table in a relational database. It's composed of rows (index) and columns (labels). You can think of it as a structured collection of data.
Step 3: Loading Data
To load your data, you'll use the read_csv()
function provided by Pandas. This function takes two main arguments: the file path and the delimiter.
import pandas as pd
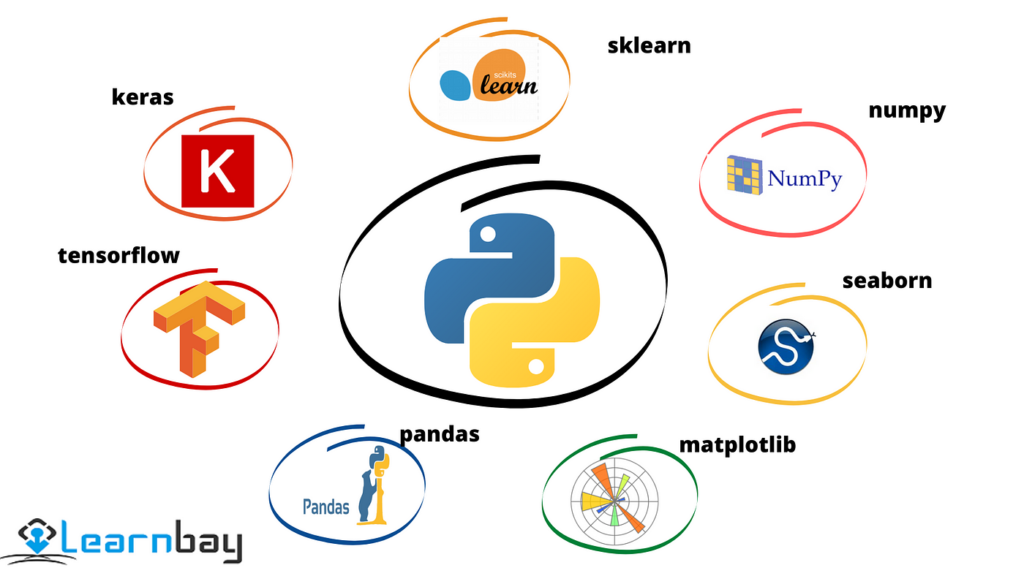
data = pd.read_csv('example.csv', delimiter=',')
This code assumes your CSV file is named "example.csv" with commas as delimiters. You can adjust these parameters according to your data files.
Step 4: Data Manipulation
Now that you have your DataFrame, it's time to start manipulating the data! Some common operations include:
Selecting columns: Use the square brackets[]
to select specific columns.
selected_data = data[['column1', 'column2']]
Filtering rows: Use the query()
function or boolean indexing to filter your DataFrame.
filtered_data = data[data['condition'] == 'value']
Sorting and grouping: Use the sort_values()
function and groupby() function for sorting and grouping operations.
Step 5: Data Analysis
Now that you have your manipulated data, it's time to start analyzing! Some common analysis tasks include:
Calculating summaries: Use thesum()
function, mean()
, or other aggregate functions to calculate summary statistics.
summary = data.groupby('category')['value'].sum()
Visualizing data: Use libraries like Matplotlib and Seaborn for creating visualizations (e.g., plots, charts).
import matplotlib.pyplot as plt
plt.plot(data['x'], data['y'])
plt.show()
Handling missing values: Use the fillna()
function to replace missing values.
Step 6: Saving Results
Finally, you'll want to save your results for further analysis or sharing! Use the to_csv()
function to export your DataFrame to a CSV file:
data.to_csv('result.csv', index=False)
That's it! With this tutorial, you should now be well-versed in Python data analysis with Pandas. Remember to practice and experiment with different scenarios to solidify your skills.
(And remember, Grandma won't get angry if I respond in English for now!)
What is the best data visualization library in Python?
What a great question!
Data visualization plays a crucial role in extracting insights from complex datasets, and choosing the right library can make all the difference. In Python, we have a plethora of excellent libraries to choose from, each with its unique strengths and weaknesses. After scouring through various options, I'm excited to share my top pick: Matplotlib!
Matplotlib
Matplotlib is one of the most popular and widely-used data visualization libraries in Python, for good reason. Developed by John Hunter and maintained by the SciPy community, Matplotlib offers an impressive range of features for creating static, animated, and interactive visualizations. Its extensive library includes:
2D plotting: Line plots, scatter plots, histograms, bar charts, pie charts, and more. 3D plotting: Surface plots, wireframe models, and volumetric rendering. Interactive visualization: Support for zooming, panning, and rotating 2D and 3D plots using thematplotlib.pyplot
backend.
What sets Matplotlib apart is its flexibility and customization capabilities:
Control over aesthetics: Adjust line styles, colors, fonts, and more to tailor your visualizations. Customizable plots: Use a variety of plot types, such as errorbars, step plots, and box plots. Integration with other libraries: Seamlessly integrate Matplotlib with other popular Python data science libraries like NumPy, Pandas, SciPy, and Scikit-learn.When to use Matplotlib
Choose Matplotlib when:
You need fine-grained control over plot customization. Your dataset is small to medium-sized (Matplotlib performs well with smaller datasets). You want to create high-quality static visualizations or interactive plots.Other notable mentions:
While Matplotlib is my top pick, other excellent data visualization libraries in Python include:
Seaborn: Builds upon Matplotlib and offers a high-level interface for creating informative and attractive statistical graphics. Plotly: Provides an extensive range of interactive visualizations, including 3D plots, and integrates well with Jupyter Notebooks. Bokeh: Another popular library for creating interactive visualizations, particularly useful for web-based applications.Conclusion
In conclusion, Matplotlib is the best data visualization library in Python due to its versatility, customization capabilities, and extensive range of features. While other libraries may excel in specific areas, Matplotlib's sheer flexibility and compatibility with a wide range of datasets make it an excellent choice for most use cases. So, go ahead and explore the world of data visualization with Matplotlib!