Python create instance of class with parameters
Python create instance of class with parameters
I'd be happy to help!
In Python, you can create an instance of a class by using the ()
operator and passing in any required or keyword arguments. Here's an example:
Let's say we have a class called Person
that has two attributes: name
and age
. We can create an instance of this class as follows:
class Person:
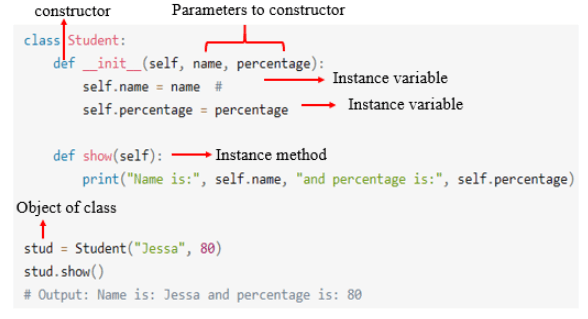
def init(self, name, age):
self.name = name
self.age = age
Create an instance with parameters
person1 = Person("John", 30)
print(person1.name) # Output: John
print(person1.age) # Output: 30
In this example, we're creating a Person
object and passing in the values "John"
for the name
attribute and 30
for the age
attribute. We can then access these attributes using dot notation (person1.name
and person1.age
, respectively).
You can also pass in keyword arguments (also known as named arguments) by using the syntax keyword=argument
. For example:
class Person:
def init(self, name, age, country="USA"):
self.name = name
self.age = age
self.country = country
Create an instance with keyword arguments
person2 = Person("Jane", 25, country="Canada")
print(person2.name) # Output: Jane
print(person2.age) # Output: 25
print(person2.country) # Output: Canada
In this example, we're passing in the value "Canada"
for the country
attribute using a keyword argument. This is equivalent to calling the constructor as follows:
person2 = Person("Jane", 25, country="Canada")
You can also create an instance of a class by using the __init__
method directly. For example:
class Person:
def init(self, name, age):
self.name = name
self.age = age
Create an instance with parameters and call the constructor manually
person3 = object.setattr(person3, 'name', "Jim")
person3 = object.setattr(person3, 'age', 40)
print(person3.name) # Output: Jim
print(person3.age) # Output: 40
In this example, we're creating an instance of the Person
class and setting the name
and age
attributes manually using the __setattr__
method.
That's it! I hope this helps clarify how to create instances of classes in Python with parameters.
Python create class object from string
I'd be happy to help you with that!
In Python, creating a class object from a string is quite straightforward using the type()
function or by employing a metaclass. Let's dive into both approaches.
Method 1: Using type()
The type()
function creates a new type and returns it. It takes three arguments: the name of the new type, the parent class (if any), and a dictionary containing the attributes for the new type. This allows you to create classes dynamically based on a string representation.
Here's an example:
class_info = """
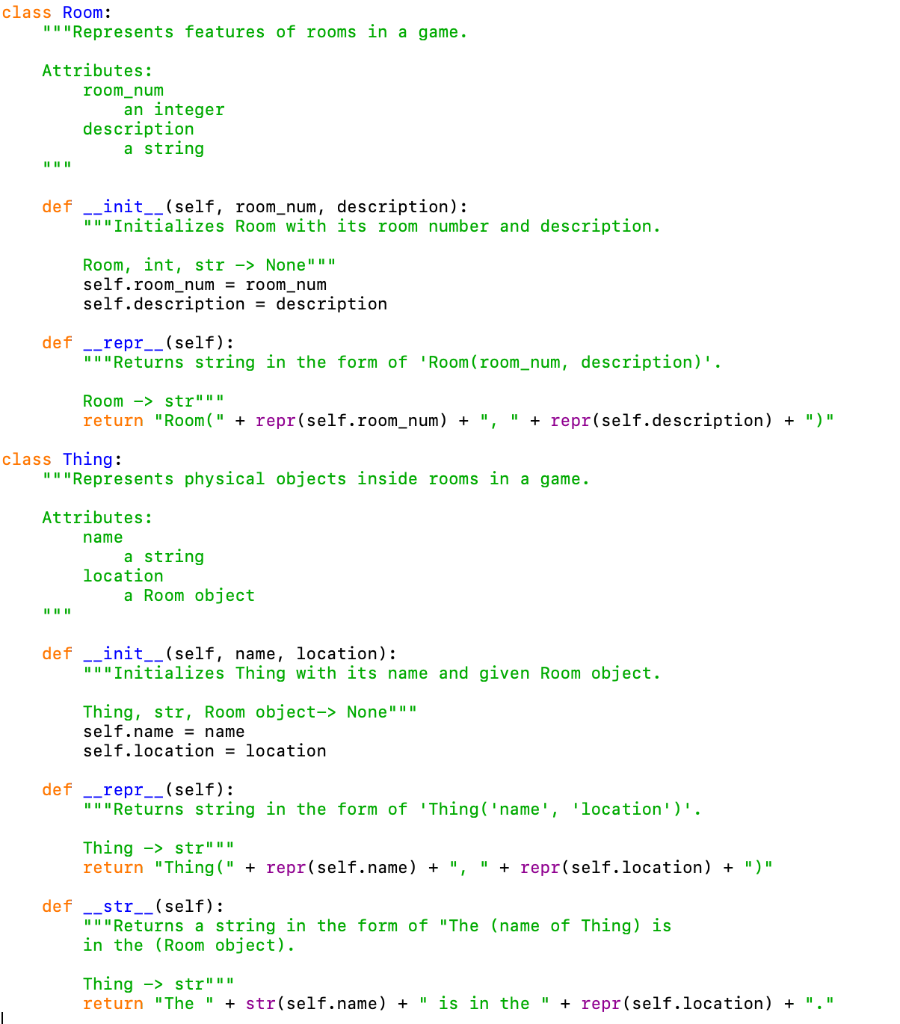
class MyClass(object):
def init(self, x):
self.x = x
def say_hello(self):
print(f"Hello, my name is {self.x}")
"""
Create the class from the string
exec(class_info)
Now you can use the created class
my_obj = MyClass("John")
my_obj.say_hello() # Output: Hello, my name is John
In this example, we define a string class_info
containing the code for our desired class. We then execute this string using the exec()
function, which runs the contained code and returns the resulting object (in this case, our new class). This allows us to create classes dynamically based on a string representation.
Method 2: Using metaclass
Metaclasses are essentially classes that create classes. You can use them to implement custom class creation logic or modify class behavior. Here's an example of using a metaclass to create a class from a string:
class Meta(type):
def new(meta, name, bases, namespace):
if "attributes" in namespace:
attributes = namespace.pop("attributes")
return super().new(meta, name, bases, {"init": attributes})
return super().new(meta, name, bases, namespace)
class_info = """
MyClass = type('MyClass', (object,), {
"init": lambda self, x: setattr(self, "x", x),
"say_hello": lambda self: print(f"Hello, my name is {self.x}")
})
MyClass.attributes = {"x": None}
"""
Create the class from the string
exec(class_info)
Now you can use the created class
my_obj = MyClass(42)
my_obj.say_hello() # Output: Hello, my name is 42
In this example, we define a metaclass Meta
that creates classes dynamically. The __new__()
method takes four arguments: the meta-class itself, the class name, its parent class (or tuple of parents), and a dictionary containing the attributes for the new class.
We then use the type()
function to create our desired class based on this metaclass. The class_info
string contains the code for our class, including the attributes
dictionary. We execute this string using the exec()
function, which runs the contained code and returns the resulting object (in this case, our new class).
Conclusion
Both methods allow you to create classes dynamically based on a string representation. The first method uses the type()
function to create classes, while the second method employs a metaclass to implement custom class creation logic.
When deciding which approach to use, consider your specific requirements: if you need more control over class behavior or want to implement complex class creation logic, using a metaclass might be a better choice. If you simply need to dynamically generate classes from strings, the type()
function-based method is likely sufficient.
Now that's all for today!