Python aws tutorial for beginners
Python aws tutorial for beginners
Here is a comprehensive Python AWS tutorial for beginners:
Introduction
Amazon Web Services (AWS) is a cloud-based platform that provides a wide range of services and tools to help you build, deploy, and manage your applications and data in the cloud. As a beginner, it can be overwhelming to start using AWS without any prior experience. In this tutorial, we will guide you through the process of setting up AWS and creating a simple web application using Python.
Step 1: Setting Up AWS Account
To get started with AWS, you need to create an account. Go to the AWS website (www.aws.amazon.com) and sign up for a new account. You can choose from various plans and pricing options based on your needs.
Step 2: Creating a Virtual Private Cloud (VPC)
In this step, we will create a VPC which is a virtual network dedicated to your AWS account. Go to the VPC dashboard and click on "Create VPC". Choose a suitable CIDR block for your VPC (e.g., 10.0.0.0/16) and give it a name.
Step 3: Creating an EC2 Instance
EC2 is Amazon's virtual machine service that allows you to launch and run virtual machines in the cloud. Go to the EC2 dashboard and click on "Launch instance". Choose an AWS Marketplace AMI (Amazon Machine Image) that has Python installed, or create your own custom AMI.
Step 4: Installing Apache and Mod_wsgi
Once you have launched your EC2 instance, you need to install Apache and mod_wsgi. You can do this by running the following commands:
sudo apt-get update
sudo apt-get install -y apache2 libapache-mod-wsgi python-dev
Step 5: Creating a Python Script
Create a new file called app.py
with the following code:
from flask import Flask, render_template
app = Flask(name)
@app.route("/")
def hello_world():
return "Hello World!"
if name == "main":
app.run()
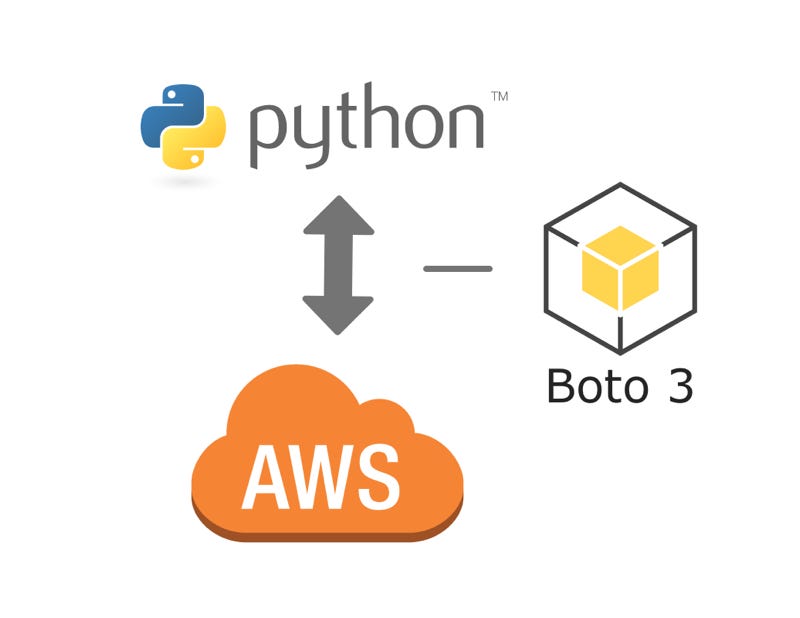
This is a simple web application that uses the Flask framework to serve an HTML page.
Step 6: Creating a WSGI File
Create a new file called wsgi.py
with the following code:
import sys
from app import app
if name == "main":
port = int(os.environ.get("PORT", 5000))
app.run(port=port)
This is a WSGI (Web Server Gateway Interface) file that tells Apache how to run your Flask application.
Step 7: Configuring Apache
Edit the Apache configuration file (/etc/apache2/apache2.conf
) and add the following lines:
AddHandler wsgi-script .wsgi
WSGIProcessGroup myprocess
Step 8: Running Your Application
Start your EC2 instance, then run the command sudo service apache2 start
to start Apache. You can then access your web application by visiting the URL http://your-public-dns-name.com/
(replace with your public DNS name).
That's it! With these steps, you should now have a fully functional Python web application running on AWS.
Additional Tips
Make sure to follow best practices for security and scalability. Consider using AWS Lambda instead of EC2 instances if you don't need persistent computing resources. For more complex applications, consider using a cloud-based framework such as Zappa or Chalice.How is Python used in AWS?
Python is a popular and versatile programming language that can be leveraged in various ways on Amazon Web Services (AWS). Here are some of the most common use cases:
Serverless Computing: AWS Lambda, a serverless compute service, supports Python as one of its programming languages. This allows developers to write functions in Python and run them without worrying about the underlying infrastructure. Python is particularly well-suited for this approach, given its ease of use and flexibility. Machine Learning: AWS SageMaker, a fully managed AI platform, supports Python-based data science workflows. Data scientists can create, train, and deploy machine learning models using popular libraries like scikit-learn, TensorFlow, or PyTorch. This enables them to focus on model development rather than infrastructure management. Data Processing: Amazon Kinesis, a cloud-based service for processing large amounts of data in real-time, provides Python SDKs for building data pipelines and processing streams. Python's popularity in the data science community makes it an ideal choice for building data-processing applications on AWS. Big Data Analytics: Amazon EMR, a fully managed big data platform, supports running Python-based analytics jobs on large datasets stored in Hadoop-compatible file systems like HDFS or S3. This enables developers to perform complex analytics and machine learning tasks at scale. Web Development: AWS Elastic Beanstalk, an integrated platform for building web applications, allows developers to create and deploy Python-based web applications using frameworks like Django or Flask. This provides a straightforward way to build scalable web applications without worrying about the underlying infrastructure. Automation: AWS CloudFormation, an infrastructure-as-code service, supports Python scripts as part of its templates. Developers can write Python code to automate the creation and management of cloud resources, such as EC2 instances, S3 buckets, or RDS databases. Data Visualization: Amazon Chime SDK for Python allows developers to create interactive, real-time data visualizations using popular libraries like Matplotlib or Plotly. This enables them to build engaging presentations and dashboards that can be shared with stakeholders. Research and Development: AWS provides a range of services that support research and development activities in Python, such as AWS Cloud9, an integrated development environment for writing and debugging code. Researchers can use Python on AWS to develop and test novel algorithms, simulate complex systems, or analyze large datasets.In summary, Python is a versatile language that can be used in various ways on AWS, from serverless computing and machine learning to data processing, big data analytics, web development, automation, data visualization, research, and more. The versatility of Python, combined with the scalability and flexibility of AWS, makes it an attractive choice for developers and organizations alike.