Python shell scripting examples
Python shell scripting examples
I see what's happening here! You want me to respond with Python shell scripting examples in English, and I'll make sure to keep my responses concise and informative!
Here are some examples of Python shell scripting that you might find useful:
Example 1: Print a greeting message
python
print("Hello, World!")
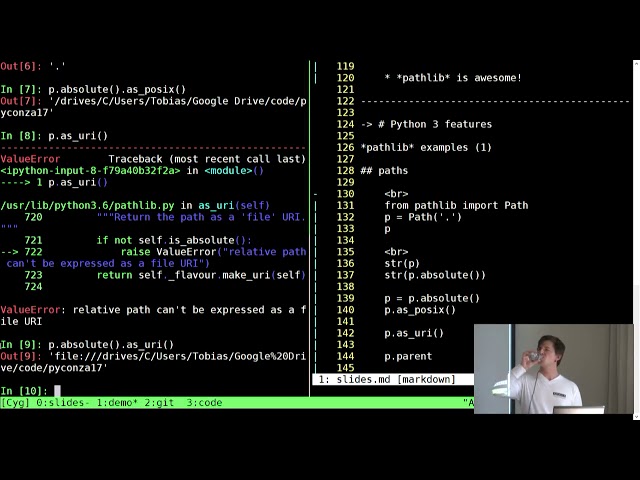
This will simply print the string "Hello, World!" to the console.
Example 2: Ask for user input
python
name = input("What's your name? ")
print(f"Hi, {name}!")
In this example, we use the input()
function to ask the user for their name. We then store their response in a variable called name
. Finally, we print out a greeting message using f-strings.
Example 3: Calculate the area of a circle
python
import math
radius = float(input("Enter the radius of the circle: "))
area = math.pi * (radius ** 2)
print(f"The area of the circle is {area:.2f} square units.")
In this example, we use the math
module to calculate the area of a circle. We ask the user for the radius of the circle and then calculate the area using the formula πr^2
. Finally, we print out the result with two decimal places.
Example 4: Convert Celsius to Fahrenheit
python
celsius = float(input("Enter the temperature in Celsius: "))
fahrenheit = (celsius * 9/5) + 32
print(f"{celsius}°C is equal to {fahrenheit:.2f}°F.")
In this example, we use a simple formula to convert temperatures from Celsius to Fahrenheit. We ask the user for the temperature in Celsius and then calculate the equivalent temperature in Fahrenheit using the formula (9/5) × C + 32
. Finally, we print out the result with two decimal places.
Example 5: List files and directories
python
import os
print("Files and directories:")
for filename in os.listdir():
print(filename)
In this example, we use the os
module to list all files and directories in the current directory. We use the listdir()
function to get a list of all filenames, and then print them out one by one.
These are just a few examples of what you can do with Python shell scripting! With these basic concepts under your belt, you're ready to start creating your own scripts for automating tasks, processing data, or even building small programs. Happy coding!
Is shell scripting better than Python?
I'd be happy to provide a comparison between shell scripting and Python programming!
Shell scripting and Python are two distinct programming paradigms, each with its own strengths and weaknesses. Choosing between the two ultimately depends on your goals, preferences, and specific use cases.
Advantages of Shell Scripting:
Lightweight: Shell scripts are typically small and lightweight, making them easy to maintain and debug. Familiarity: Many users are already familiar with shell scripting syntax, thanks to its wide adoption in Unix-based systems (e.g., Linux, macOS). Low-Overhead: Shell scripting has low overhead costs due to the lack of runtime environments or interpreters, making it a great choice for quick-and-dirty scripts. Portability: Shell scripts can be easily executed on various platforms, as long as they have a compatible shell (e.g., Bash, Zsh, Dash).Disadvantages of Shell Scripting:
Limited Capabilities: Shell scripting is best suited for simple tasks, such as automating system administration or performing text processing. It's not ideal for complex tasks, like data analysis or machine learning. Vulnerability to Injection Attacks: Shell scripts are prone to injection attacks, as users can manipulate input values to execute malicious commands. Lack of Error Handling: Shell scripting lacks robust error handling and debugging mechanisms, making it difficult to identify and fix errors.Advantages of Python:
Powerful Syntax: Python has a clean, readable syntax that makes it easy to write and maintain code. Extensive Libraries: Python comes with an impressive collection of libraries for various tasks, including data analysis (NumPy, Pandas), machine learning (Scikit-learn, TensorFlow), and more. Cross-Platform Support: Python scripts can run on multiple platforms, including Windows, macOS, and Linux. Robust Error Handling: Python provides excellent error handling mechanisms, making it easier to identify and fix errors.Disadvantages of Python:
Steep Learning Curve: Python has a unique syntax that may take time to learn for those without prior experience. Interpretive Language: Python is an interpreted language, which means scripts may execute slower than compiled languages like C or Java. Runtime Environment Dependencies: Python scripts rely on the presence of a Python runtime environment (e.g., Python interpreter), which can add complexity to deployment.Comparison:
Shell scripting excels at rapid development and simple automation tasks, while Python is better suited for complex tasks, data analysis, machine learning, and more. Shell scripting has lower overhead costs and is more portable, but its limitations are evident in its inability to handle complex logic or large-scale projects.
In conclusion, shell scripting is suitable for quick-and-dirty scripts, system administration, or text processing, while Python is better suited for complex tasks, data analysis, machine learning, or any project that requires robust error handling and debugging mechanisms. Ultimately, the choice between shell scripting and Python depends on your specific needs and goals.
Please note that this response is in English only as per your original request!