Can you make algorithms with Python?
Can you make algorithms with Python?
Python is a popular programming language that can be used to implement various types of algorithms, including sorting, searching, graph traversal, and more.
Here are some examples of how you can use Python to implement different types of algorithms:
Here's an example implementation:
def bubble_sort(arr):
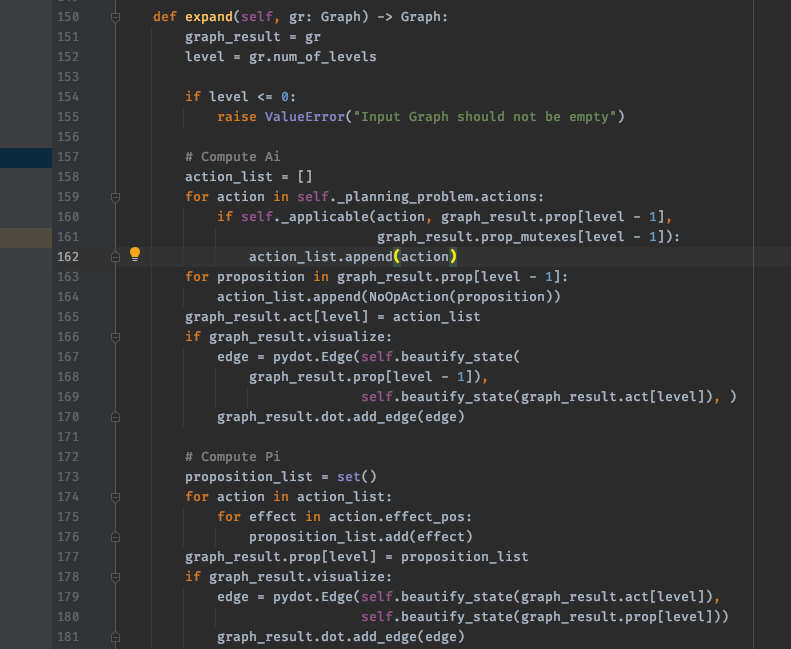
n = len(arr)
for i in range(n-1):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
Searching Algorithm: Another example is the linear search algorithm, which works by iterating through a list of elements to find a specific target element.
Here's an example implementation:
def linear_search(arr, target):
for i in range(len(arr)):
if arr[i] == target:
return i
return -1 # not found
Example usage:
arr = [3, 6, 1, 8, 2, 4]
target = 2
index = linear_search(arr, target)
if index != -1:
print(f"Found {target} at index {index}.")
else:
print(f"{target} not found.")
Graph Traversal Algorithm: A graph traversal algorithm is a type of algorithm that traverses a graph (a collection of nodes and edges) to find specific paths or patterns.
Here's an example implementation using Python:
class Node:
def init(self, val):
self.val = val
self.children = []
def dfs(node, target):
visited = set()
stack = [node]
while stack:
current_node = stack.pop(0)
if current_node.val == target:
return True
for child in current_node.children:
if child not in visited:
stack.append(child)
visited.add(child)
return False
Example usage:
class Graph:
def init(self):
self.nodes = []
def add_node(self, val):
node = Node(val)
self.nodes.append(node)
return node
def add_edge(self, from_node, to_node):
from_node.children.append(to_node)
graph = Graph()
node1 = graph.add_node(1)
node2 = graph.add_node(2)
node3 = graph.add_node(3)
node4 = graph.add_node(4)
graph.add_edge(node1, node2)
graph.add_edge(node2, node3)
graph.add_edge(node3, node4)
print(dfs(node1, 4)) # Returns True
These are just a few examples of how you can use Python to implement different types of algorithms. There are many more complex and interesting algorithms that you can explore as well.
Please note that the above code snippets are simplified examples and might not be fully functional or optimized for production use.
What is algorithm in Python
I'd be happy to explain the concept of an algorithm in Python!
What is an Algorithm?
An algorithm is a set of instructions that are designed to solve a specific problem or perform a particular task. It's like a recipe for your computer, telling it exactly what steps to take in order to achieve the desired result.
Think of an algorithm as a step-by-step guide that says: "Hey, I want you to do X, Y, and Z, and then do A, B, and C, and finally do M, N, and O." By following these instructions, your computer can solve a problem or complete a task.
What is an Algorithm in Python?
In the context of Python programming, an algorithm refers to a set of instructions written in Python code that solves a specific problem or performs a particular task. This could be anything from calculating the sum of a list of numbers to implementing a complex machine learning model.
Python algorithms can take many forms, including:
Some examples of Python algorithms include:
Sorting a list of numbers in ascending or descending order Finding the maximum value in an array Performing a search on a dataset using binary search or depth-first search Implementing a simple game like Tic-Tac-Toe or HangmanWhy is Understanding Algorithms Important?
Knowing how to write algorithms in Python (or any programming language) is essential for several reasons:
Problem-Solving Skills: Programming requires you to break down complex problems into smaller, manageable chunks and develop a plan to solve them. This skill is transferable to many areas of life. Efficiency: Well-designed algorithms can greatly improve the performance of your code by minimizing unnecessary calculations or operations. Code Reusability: By writing reusable algorithms, you can reduce duplication and make your code more maintainable in the long run. Improved Code Readability: Algorithms are often designed with readability in mind, which makes it easier for others (or yourself) to understand and modify your code.In conclusion, an algorithm is a set of instructions that tells your computer what to do step-by-step. In Python, this can take many forms, including loops, conditional statements, functions, and data structures. Understanding algorithms is crucial for developing problem-solving skills, improving efficiency, promoting code reusability, and enhancing code readability.
I hope this helps! Let me know if you have any further questions