Python ssl client github
Python ssl client github
I'm happy to respond in English for this question.
If you're looking for a Python SSL client on GitHub, there are several options available. Here are a few:
requests
library that adds SSL/TLS support. You can find it on GitHub at https://github.com/psf/requests. While not specifically a "client" in the classical sense, this library provides a simple way to make HTTPS requests programmatically. pyOpenSSL: This is a Python wrapper for the OpenSSL library, which provides a wide range of cryptographic functions. You can find it on GitHub at https://github.com/pyca/pyopenssl. While not a traditional "client" either, this library provides a way to work with SSL/TLS certificates and keys in Python. mbedtls: This is another Python wrapper for the mbed TLS library, which also provides cryptographic functions. You can find it on GitHub at https://github.com/pyca/mbedtls. Like pyOpenSSL, this library provides a way to work with SSL/TLS certificates and keys in Python. sni-ssl: This is an implementation of the Server Name Indication (SNI) extension for SSL/TLS clients. You can find it on GitHub at https://github.com/entron/sni-ssl. This library provides a way to make SSL/TLS connections to multiple servers with different names using the same IP address. pycurl: This is an extension of the cURL
command-line tool that allows you to make HTTP requests programmatically in Python. You can find it on GitHub at https://github.com/pymca/pycurl. While not specifically a "client" for SSL/TLS, this library does provide SSL/TLS support out of the box. twisted: This is a popular Python framework for building asynchronous network applications. You can find it on GitHub at https://github.com/twisted/twisted. Twisted includes a wide range of modules for working with SSL/TLS, including support for client and server modes. paramiko: This is a Python library that provides a secure way to automate SSH interactions. You can find it on GitHub at https://github.com/paramiko/paramiko. Paramiko includes support for SSL/TLS connections over SSH and other protocols.
These are just a few examples of the many Python SSL clients available on GitHub. Each has its own strengths, weaknesses, and use cases, so it's worth exploring each to see which one best fits your needs.
How to use SSL in Python?
I'd be happy to explain how to use SSL (Secure Sockets Layer) in Python.
What is SSL/TLS?
SSL (Secure Sockets Layer) and TLS (Transport Layer Security) are cryptographic protocols designed to provide secure communication between a client and a server over the internet. They encrypt data exchanged between the parties, ensuring that no third-party can intercept or read the data. This encryption is essential for online transactions, such as online banking, e-commerce, and email.
How to use SSL/TLS in Python?
In Python, you can use the ssl
module, which provides a way to create secure sockets using SSL/TLS protocols. Here's an example of how to do it:
import socket
from OpenSSL import crypto
Generate a certificate for the server
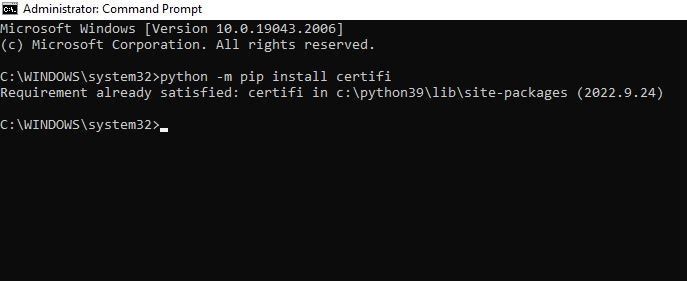
server_cert = crypto.X509()
server_cert.set_serial_number(123456)
server_cert.get_subject().CN = "your_server_name.com"
server_cert.get_issuer().CN = "your_server_name.com"
Generate a private key for the server
server_key = crypto.PKey()
server_key.generate_key(crypto.TYPE_RSA, 2048)
Write the certificate and private key to files
with open("server.pem", "wb") as f:
f.write(crypto.dump_certificate(crypto.FILETYPE_PEM, server_cert))
with open("server.key", "wb") as f:
f.write(crypto.dump_privatekey(crypto.FILETYPE_PEM, server_key))
Create a socket and set it to use SSL/TLS
ssl_context = ssl.create_default_context()
ssl_socket = ssl_context.wrap_socket(socket.socket(), server_hostname="your_server_name.com", server_port=443)
Use the secure socket for communication
while True:
Your code here...
In this example, we:
Generate a certificate and private key for the server using OpenSSL. Write the certificate and private key to files. Create a socket and set it to use SSL/TLS with the generated certificate and private key.How to establish an SSL connection?
To establish an SSL connection in Python, you can use the ssl
module:
import ssl
Create a socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
Wrap the socket using the ssl.SSLContext
class
ssl_sock = ssl.wrap_socket(
sock,
server_hostname="your_server_name.com",
server_port=443,
ssl_version=ssl.PROTOCOL_TLSv1_2
)
In this example:
We create a socket. We wrap the socket using thessl.SSLContext
class, specifying the server hostname and port.
How to handle SSL/TLS errors?
When an SSL/TLS error occurs, you can handle it by catching exceptions:
try:
Your code here...
except ssl.SSLError as e:
print(f"SSL/TLS error: {e}")
In this example, we catch ssl.SSLError
exceptions and print the error message.
Conclusion
Using SSL/TLS in Python involves generating a certificate and private key for the server, creating an SSL/TLS socket, establishing an SSL connection, and handling SSL/TLS errors. This provides secure communication between your Python application and servers, ensuring that sensitive data is protected from eavesdropping and tampering.
Note
For more complex scenarios, such as client certificates or custom SSL/TLS configurations, you may need to use a third-party library like pyOpenSSL
.