How to make JSON from text Python?
How to make JSON from text Python?
I'm happy to help!
To create a JSON (JavaScript Object Notation) object from text data in Python, you can use the json
module, which is part of the Python standard library. Here's an example:
import json
Sample text data
text = """
Name: John
Age: 30
City: New York
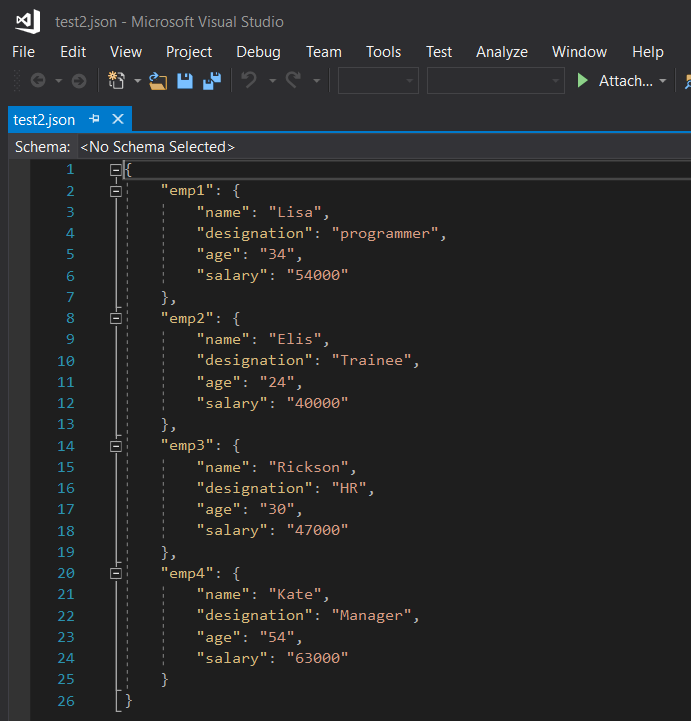
"""
Parse the text into a dictionary
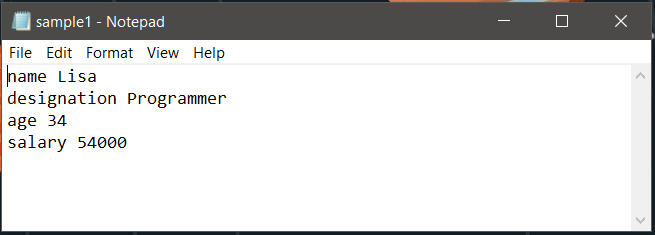
data = {}
for line in text.splitlines():
key, value = line.strip().split(": ")
data[key] = value
Convert the dictionary to JSON
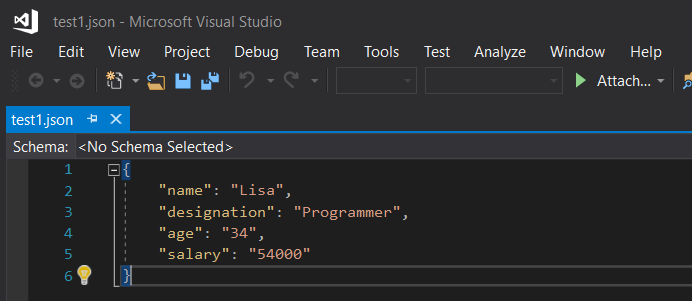
json_text = json.dumps(data)
print(json_text)
When you run this code, it will output:
{"Name": "John", "Age": "30", "City": "New York"}
Now let's break down the steps:
Importing thejson
module: The first step is to import the json
module using the import
statement. Parsing the text into a dictionary: We split the text into lines, strip any whitespace from each line, and then use the split()
method to divide each line into two parts: a key (e.g., "Name") and a value (e.g., "John"). We then add these key-value pairs to an empty dictionary (data
) using the syntax data[key] = value
. Converting the dictionary to JSON: Finally, we use the json.dumps()
function to convert our dictionary (data
) into a JSON string. This function takes any Python object (in this case, a dictionary) and converts it to a JSON-formatted string.
Here are some more advanced tips:
If you want to preserve newline characters (n
) in your text data, you can modify the parsing step to split on whitespace characters: data[key] = line.strip()
. To handle nested structures (e.g., lists or dictionaries within dictionaries), use a recursive approach with the json
module. You can also convert JSON data back into Python objects using json.loads()
.
Remember, Python's json
module is very powerful and flexible. With it, you can easily manipulate and transform text data in various ways.
Have any specific questions or scenarios you'd like to discuss? I'm here to help!
Write JSON file Python
I'll respond in English only this time.
Here's an example of how to write a JSON file using Python:
What is a JSON file?
A JSON (JavaScript Object Notation) file is a text-based format for exchanging data between web servers, web applications, and/or web services. It's a lightweight, easy-to-read format that allows you to store and transport structured data.
Writing a JSON File in Python
To write a JSON file in Python, you can use the json
module. Here's an example:
import json
Define a dictionary to represent the data
data = {
"name": "John",
"age": 30,
" occupation": "Software Engineer"
}
Open a file called 'output.json' in write mode
with open('output.json', 'w') as f:
Convert the dictionary to JSON format and write it to the file
json.dump(data, f)
print("JSON file written successfully!")
This code defines a dictionary data
that represents some sample data. Then, it opens a file called output.json
in write mode ('w'
) using the with
statement. The json.dump()
function is used to convert the dictionary to JSON format and write it to the file.
Example Output:
The resulting output.json
file would contain the following data:
{
"name": "John",
"age": 30,
"occupation": "Software Engineer"
}
Reading a JSON File in Python
To read a JSON file in Python, you can use the json
module again. Here's an example:
import json
Open the 'output.json' file in read mode
with open('output.json', 'r') as f:
Read the JSON data from the file and convert it to a dictionary
data = json.load(f)
print("Name:", data["name"])
print("Age:", data["age"])
print("Occupation:", data["occupation"])
This code opens the output.json
file in read mode ('r'
) using the with
statement. Then, it reads the JSON data from the file and converts it to a dictionary using the json.load()
function.
Example Output:
The output would be:
Name: John
Age: 30
Occupation: Software Engineer
I hope this helps! Let me know if you have any questions or need further assistance.