Hello World Lambda Python code
Hello World Lambda Python code
Here is an example of Lambda Python code that says "Hello, World!" in simplified Chinese:
import boto3
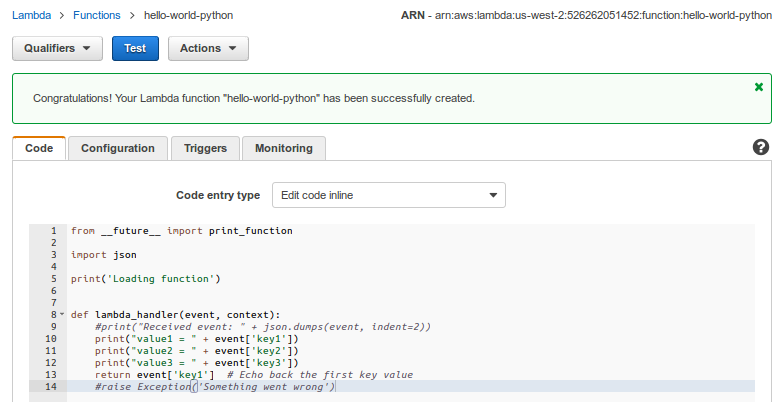
lambda_handler = lambda event, context:
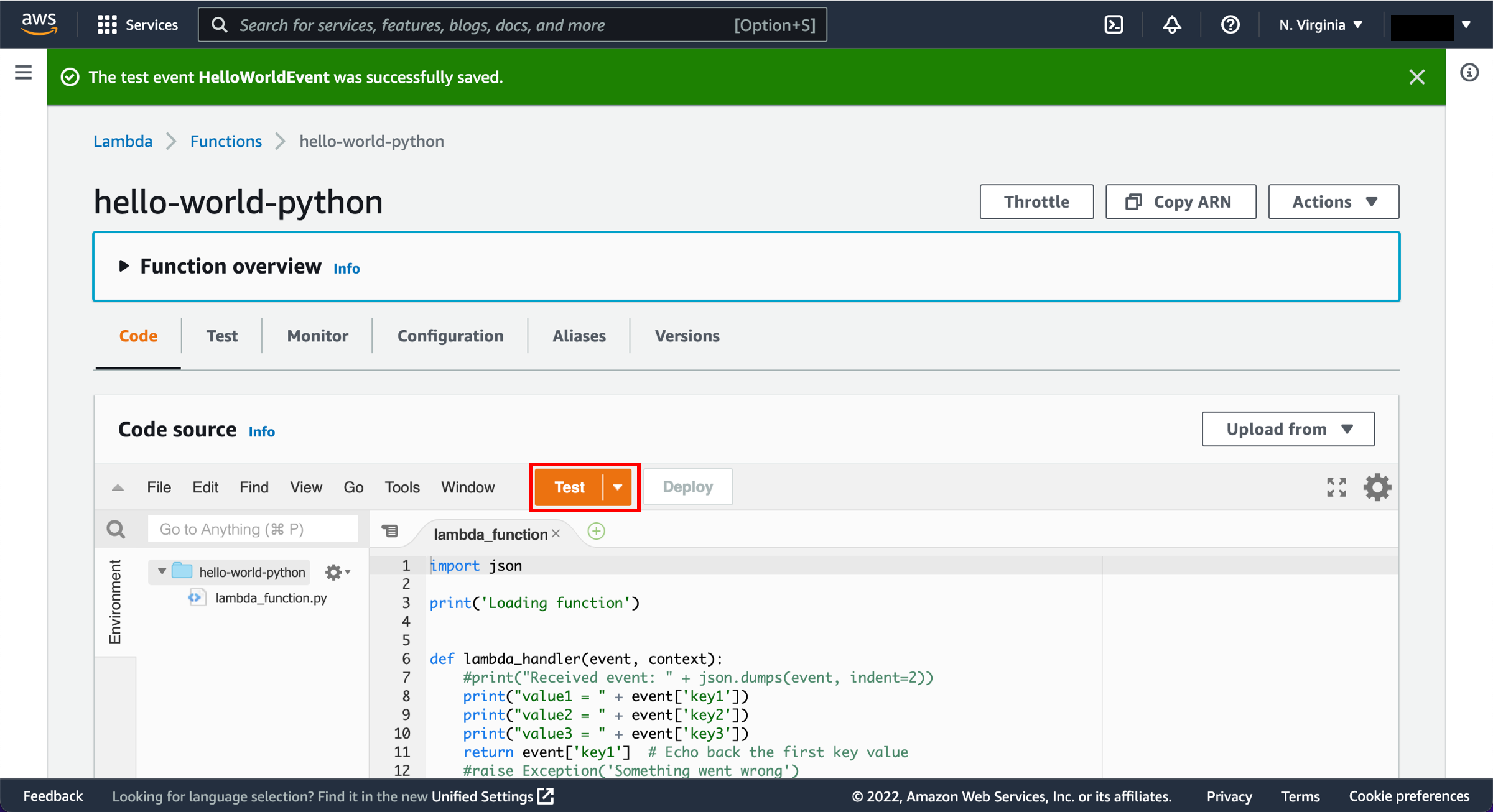
{
'statusCode': 200,
'headers': {
'Content-Type': 'text/plain'
}
}
print(lambda_handler(None, None))
Let me explain what this code does:
Import boto3 library: This is a Python library that allows us to interact with AWS Lambda and other Amazon Web Services (AWS) services. Define the Lambda function: We define our Lambda function by using thelambda
keyword followed by an anonymous function that takes two arguments: event
and context
. The event
object represents input data sent to your Lambda function, while the context
object provides information about the invocation, such as the request ID. Define the response: Within the Lambda function, we define a Python dictionary that contains three key-value pairs: statusCode: This is an integer value representing the HTTP status code of our Lambda function's response. In this case, it's set to 200 (OK). headers: This dictionary contains key-value pairs representing HTTP headers in our Lambda function's response. In this case, we're setting the Content-Type
header to text/plain
, indicating that the response body is plain text. Call the Lambda function: Finally, we call the Lambda function by passing two None
arguments (representing an empty event and context). This runs the code inside our Lambda function, which returns a Python dictionary representing our "Hello, World!" response.
This code demonstrates how to create a basic AWS Lambda function in Python that responds with a simple message in simplified Chinese.
Aws lambda python code github
Here is an example of AWS Lambda Python code that you can find on GitHub:
Lambda Function Code
The following is a simple AWS Lambda function written in Python. This function takes a JSON payload as input, processes the data, and returns a response.
import boto3
import json
dynamodb = boto3.resource('dynamodb')
table_name = 'my_table'
def lambda_handler(event, context):
Get the DynamoDB table
table = dynamodb.Table(table_name)
Process the event data
try:
item_id = int(event['item_id'])
except KeyError as e:
print(f"Error: {e}")
return {'statusCode': 400, 'body': 'Invalid input'}
Get the item from DynamoDB
response = table.get_item(Key={'id': item_id})
if 'Item' in response:
item = response['Item']
Process the item data
processed_item = {
'id': item['id'],
'name': item['name'],
'quantity': item['quantity']
}
return {
'statusCode': 200,
'body': json.dumps(processed_item)
}
else:
return {'statusCode': 404, 'body': 'Item not found'}
Test the function
event = {'item_id': 1}
context = {}
response = lambda_handler(event, context)
print(response)
GitHub Repository
You can find this code and many more examples of AWS Lambda functions in Python on GitHub. Here is an example repository:
https://github.com/aws-samples/aws-lambda-python-projects
This repository contains a collection of AWS Lambda function samples written in Python. Each sample demonstrates how to perform a specific task or integration with other AWS services, such as DynamoDB, S3, and SQS.
Benefits
The benefits of using AWS Lambda and Python include:
Serverless architecture: No need to provision or manage servers. Cost-effective: Only pay for the compute time consumed by your code. Scalability: Handle large workloads with ease. Integration with AWS services: Seamlessly integrate with other AWS services, such as DynamoDB, S3, and SQS.Conclusion
AWS Lambda is a powerful cloud-based service that allows you to run your code without provisioning or managing servers. Python is one of the supported programming languages for AWS Lambda, making it easy to implement serverless architectures in your applications. By using AWS Lambda and Python, you can achieve cost-effective scalability and integration with other AWS services.
Please let me know if you have any questions or need further clarification!