What is a Python sentinel?
What is a Python sentinel?
I'd be happy to explain the concept of a Python sentinel!
In Python programming, a sentinel (also known as a marker) is a special value used to indicate the end of a sequence, such as a list or tuple. It's a clever way to simplify working with loops and conditional statements when dealing with lists, especially when you need to check if an element exists in the list.
The concept of a sentinel is often used in situations where you want to avoid having to explicitly check for "None" or other special values indicating the end of the sequence. In Python, this is particularly useful because there's no built-in way to directly determine the length of a list (like length()
in JavaScript). Instead, you can create a sentinel value that indicates when you've reached the end of the list.
Here are some examples of how a Python sentinel works:
Checking if an element exists in a list: When searching for an element within a list, it's common to check if the element is present at all. A sentinel helps in this situation. For instance, suppose you have a list of numbers, and you want to know if 5 is contained within that list: # Initialize a flag to assume 5 is not found
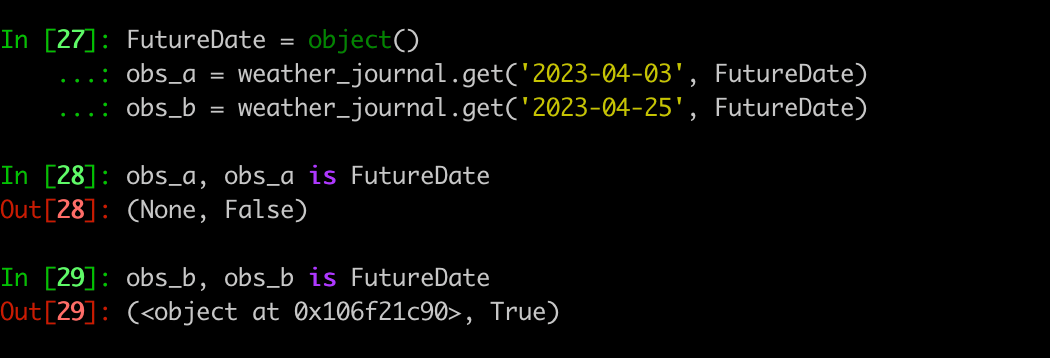
is_five_found = False
Create the list with a sentinel (e.g., None)
num_list = [1, 2, 3, 4, 5, None]
for num in num_list:
if num == 5:
is_five_found = True
break # no need to check further
if is_five_found:
print("5 exists in the list")
else:
print("5 does not exist in the list")
In this case, we're using a sentinel (None
) as a marker to signal when we've reached the end of the list. When 5 is found within the list, is_five_found
becomes True, and the loop breaks.
for... in
. Imagine you need to process all elements within a list (e.g., print their values):
sentinel_list = [1, 2, 3, "stop", None]
for item in sentinel_list:
if item == "stop":
break # stop processing
else:
print(item)
Here, we're creating a list that has a sentinel (None
) to indicate the end. When we reach "stop" during iteration, we can use break
to terminate the loop.
In both examples, having a sentinel simplifies your code by removing unnecessary checks for special values or handling edge cases like "end of list." This concept is useful when working with lists and sequences in Python, as it allows you to focus on the core logic without worrying about manually checking for end conditions.
Hope this explanation helps!
Python iter sentinel tutorial
I'd be happy to provide a detailed tutorial on Python's iterator sentinel value!
What is an Iterator?
In Python, an iterator is an object that allows you to traverse through a sequence (like a list or string) or other iterable objects one element at a time. Iterators are used to implement loops that can iterate over the elements of a collection.
The Sentinel Value Concept
When working with iterators, it's essential to understand the concept of the sentinel value. A sentinel value is a special value that indicates the end of an iteration. In other words, when the iterator reaches this value, it signals that there are no more elements left in the sequence or iterable.
In Python, the default sentinel value for many built-in iterators (like list, tuple, str) is None
. When you try to iterate over an empty sequence or a collection with no remaining elements, the iterator will return None
as its next value. This is equivalent to saying that the end of iteration has been reached.
Creating Your Own Sentinel Value
While None
works perfectly fine for many use cases, you might want to create your own sentinel value in specific situations. For example:
None
won't suffice
In Python, you can easily create your own sentinel value by returning it from the iterator when there are no more elements left.
Here's an example:
class MyIterator:
def init(self, data):
self.data = data
self.index = 0
def iter(self):
return self
def next(self):
if self.index >= len(self.data):
return "END_OF_ITERATION" # Your custom sentinel value!
else:
result = self.data[self.index]
self.index += 1
return result
In this example, we're creating a custom iterator class that iterates over a given list data
. When the end of iteration is reached (i.e., the index
exceeds the length of data
), our custom sentinel value "END_OF_ITERATION"
is returned.
Using Sentinel Values with Python's Built-in Iterators
When using built-in iterators, like those for lists or strings, you can't change their default sentinel values. However, you can work around this by creating a wrapper class that returns your custom sentinel value when there are no more elements left.
Here's an example:
def my_iter(some_list):
it = iter(some_list)
while True:
try:
yield next(it)
except StopIteration:
yield "END_OF_ITERATION" # Your custom sentinel value!
In this example, we're creating a wrapper function my_iter
that takes an iterable as input and returns an iterator. The wrapper uses the built-in iter
function to create an iterator and then enters a loop where it tries to get the next element from the iterator using next
. If a StopIteration
exception is raised (which means there are no more elements), we return our custom sentinel value "END_OF_ITERATION"
.
Conclusion
In this tutorial, you learned how to work with Python's iterator sentinel values! You saw how None
is used as the default sentinel value for many built-in iterators and how you can create your own custom sentinel value when needed. Additionally, we discussed how to use sentinel values with both built-in iterators and custom iterators.
Remember: understanding sentinel values will help you write more robust and efficient code when working with iterators in Python!