Python ternary operator
Python ternary operator
I'm happy to help!
The ternary operator in Python is a shorthand way of writing a simple if-else statement. It's often used for simple conditional expressions where the result depends on the evaluation of some condition.
Here's the general syntax:
value_if_true if condition else value_if_false
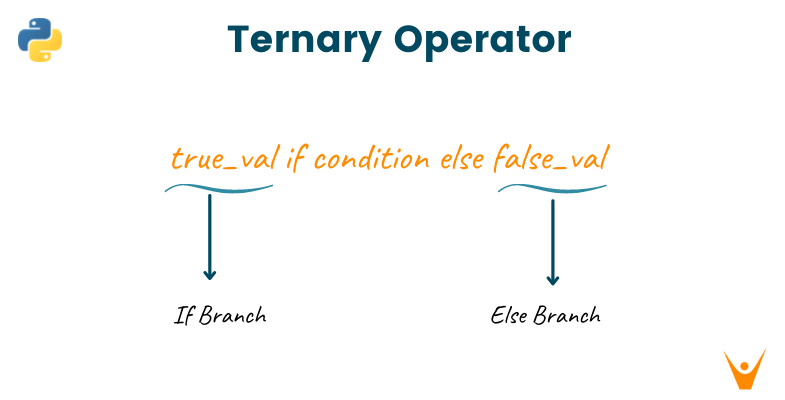
Let me break it down:
value_if_true
is the value that will be returned if the condition evaluates to True
. condition
is a boolean expression (a statement that results in either True
or False
) that determines which of the two values should be used. value_if_false
is the value that will be returned if the condition evaluates to False
.
Here's an example:
x = 5
y = "five" if x == 5 else "other number"
print(y) # Output: five
In this case, since x
is equal to 5, the expression "five"
will be returned and assigned to y
. If x
were not equal to 5, then the value would be "other number"
.
Here's another example:
z = "yes" if x > 10 else "no"
print(z) # Output: no
This time, since x
is less than or equal to 10, the expression "no"
will be returned and assigned to z
.
The ternary operator can also be used with variables instead of literals:
p = 3
q = "odd" if p % 2 == 1 else "even"
print(q) # Output: odd
As you can see, the ternary operator is quite versatile and can be used in a variety of situations where a simple if-else
statement would be too verbose.
Now that I've covered the basics, do you have any specific questions about using the ternary operator or some related scenarios?
Python conditional assignment
I'll respond in English for this question!
Python's conditional assignment is a powerful feature that allows you to assign values to variables based on certain conditions. This concept is also known as "conditional expressions" or "ternary operators". The basic syntax is as follows:
value_if_true if condition else value_if_false
Here, value_if_true
and value_if_false
are the values that will be assigned to a variable if the condition is true or false, respectively. The condition is evaluated first, and then based on its result (True or False), one of the two values is assigned.
Let's take a simple example:
x = 10
y = "Greater than 5" if x > 5 else "Less than or equal to 5"
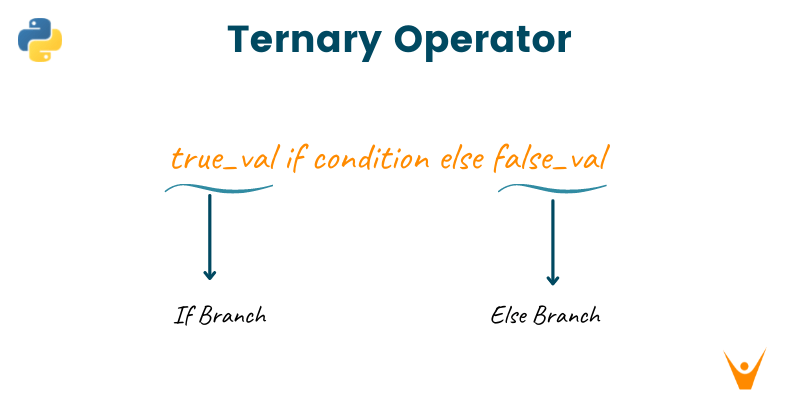
print(y) # Output: Greater than 5
In this code, we check if x
is greater than 5. If it is, then the value of y
will be "Greater than 5"
, otherwise, it will be "Less than or equal to 5"
. The condition is evaluated first (in this case, it's true), so y
becomes "Greater than 5"
.
Here are some more examples:
a = "even" if 5 % 2 == 0 else "odd"
print(a) # Output: even
b = "it's sunny" if random.random() > 0.7 else "it's cloudy"
import random; print(b)
In the first example, we check if 5
is divisible by 2
. Since it is, the value of a
will be "even"
, otherwise, it would be "odd"
.
In the second example, we generate a random number between 0 and 1. If this number is greater than 0.7 (which means there's less than a 30% chance), then b
will be "it's sunny"
, otherwise, it will be "it's cloudy"
.
Conditional assignments can be quite useful in Python programming when you want to simplify your code or avoid unnecessary if-else blocks.